原文:How to Use GitHub Copilot with Visual Studio Code,作者:Nishant Kumar
大家好!在本文中,我们将学习如何将 GitHub Copilot AI 工具与 Visual Studio Code 结合使用。
GitHub Copilot 是什么
GitHub Copilot 是一个可以帮助你更简单、更快速地编写代码的工具,由 GPT-3 提供支持。你只需编写所需代码的描述——例如,编写一个函数来生成一个随机数,或对一个数组进行排序——Copilot 就会为你创建它。
它不只是创建一种解决方案,而是创建多个,你可以选择你想要的一个。
在本教程中,我们将学习如何为 Visual Studio Code 设置 GitHub Copilot AI 工具,以及如何生成 JavaScript、React 和 HTML 代码。
如何安装 GitHub Copilot
要添加 GitHub Copilot,请打开你的 GitHub 并转到设置。
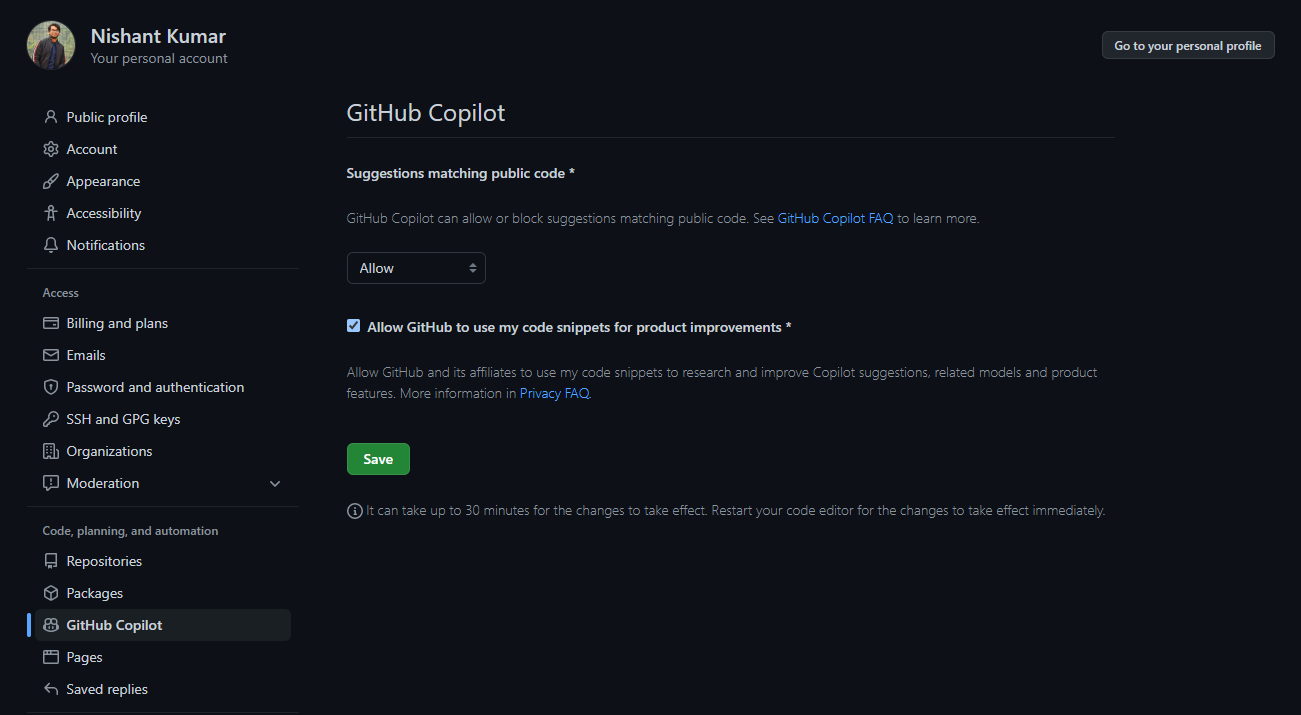
在左侧菜单中选择 GitHub Copilot 并勾选 Allow 以启用它,然后单击 Save 按钮以保存。
现在打开 Visual Studio Code 并转到 Extensions(扩展),在搜索栏中搜索 GitHub Copilot。
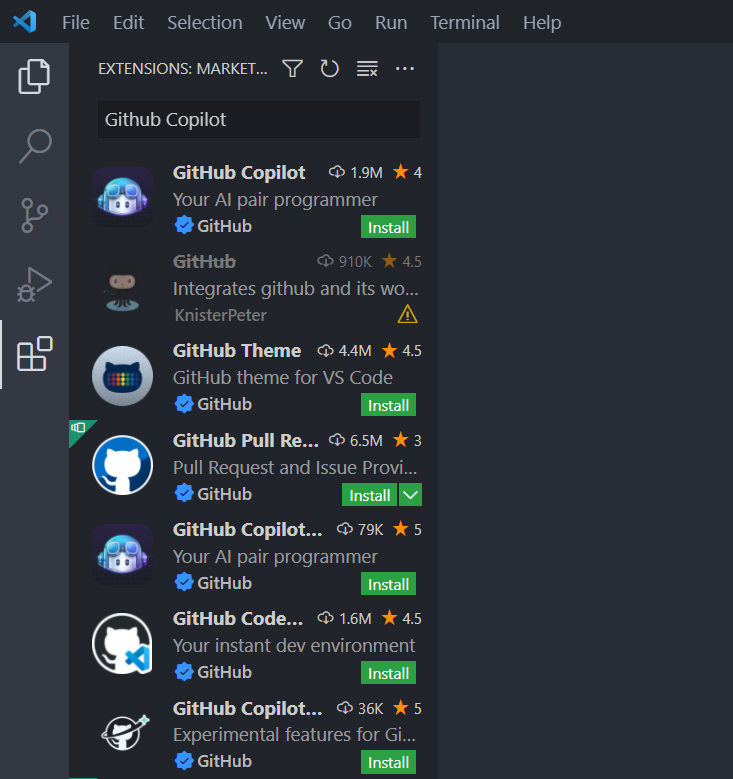
安装 Github Copilot,并重新启动你的 Visual Studio Code。

在底部,你会看到 GitHub Copilot 已被激活。

但请记住,我们目前只有试用版。而且它只有两个月的有效期——免费试用版将于 8 月 22 日结束。试用结束后,我们必须购买完整版。
每月花费 10 美元,或每年 100 美元。
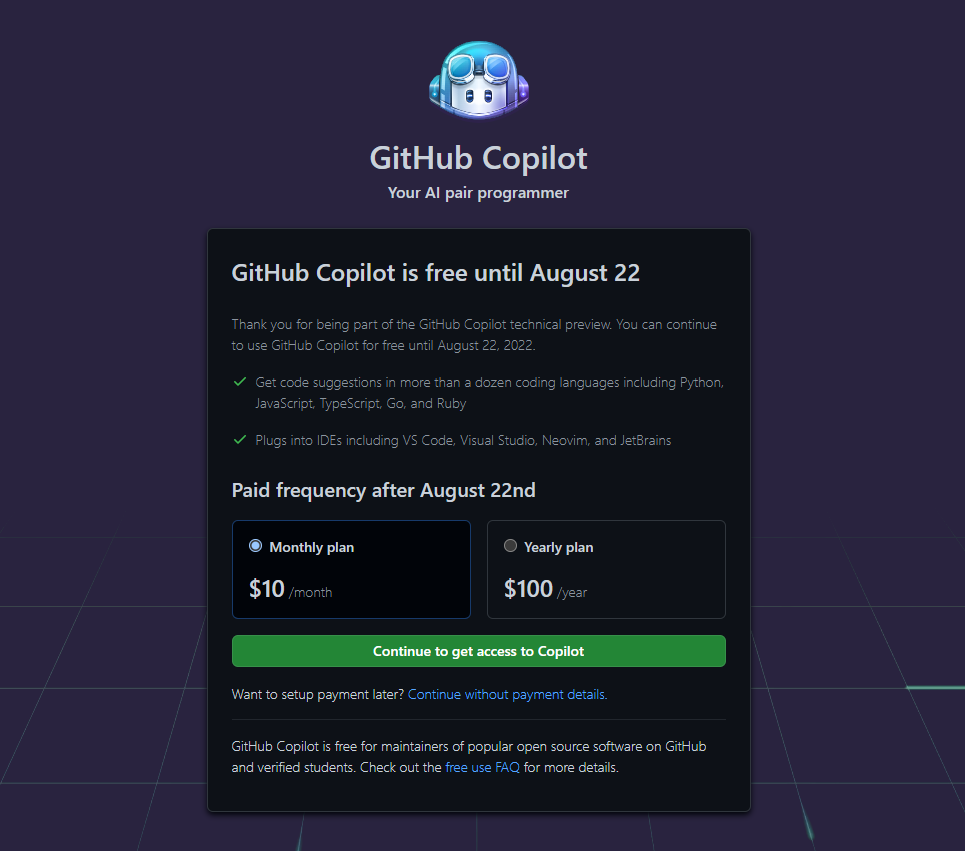
现在我们已经安装了 Copilot,让我们开始使用它的更有趣的部分。
如何使用 GitHub Copilot 生成 JavaScript 代码
让我们从简单的事情开始。让我们创建一个函数来添加两个数字。
在 JavaScript 文件中,只需写一个注释,例如 “Generate a function to add two numbers(生成一个函数以将两个数字相加)”。
//Generate a function to add two numbers
然后按 Enter 键,它会向你抛出建议,你可以通过按 Tab 键接受这些建议。
//Generate a function to add two numbers
function add(a, b) {
然后按 Enter 键进入下一行,当出现下一行代码时,再次按 Tab。
//Generate a function to add two numbers
function add(a, b) {
return a + b;
}
这是添加两个数字的函数。
现在让我们调用函数 add()
。编写函数调用,它会自动接受一些随机参数。
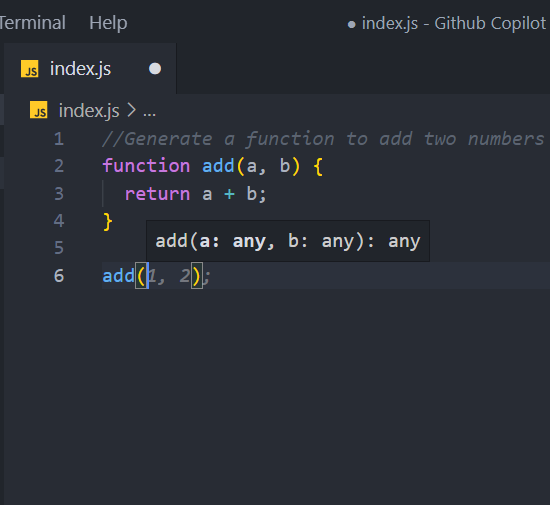
我们还可以对数字进行减法、乘法和除法。
如何使用 GitHub Copilot 生成函数以在数组中显示彩虹的颜色
我们将从注释 “Generate an array of all the colors from the rainbow(从彩虹中生成所有颜色的数组)”开始。
//Generate an array of all the colors from the rainbow
然后就像之前一样,我们将按 Enter 键。

它将生成彩虹中所有颜色的数组。
//Generate an array of all the colors from the rainbow
var colors = ['red', 'orange', 'yellow', 'green', 'blue', 'indigo', 'violet'];
如何使用类型 Number、String 和 Boolean 创建三个数组并将它们合并到一个对象中
现在让我们尝试创建一个包含数字、字符串和布尔值的数组。
//Create an array of numbers
var numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
//Create an array of strings
var strings = ["hello", "world", "how", "are", "you"];
//Create an array of booleans
var booleans = [true, false, true, false, true];
现在,让我们将它们合并到一个对象中。我们将创建一个像这样的对象:
var objects = [
{
number: 1,
string: "hello",
boolean: true
},
{
number: 2,
string: "world",
boolean: false
},
{
number: 3,
string: "how",
boolean: true
},
]
写一条注释 “Create an array of objects with the above array items as key value pairs(创建一个对象数组,将上述数组项作为键值对)”。
你可以按 Tab 键接受解决方案,或按 CTRL + Enter 键打开 Copilot 解决方案页面。
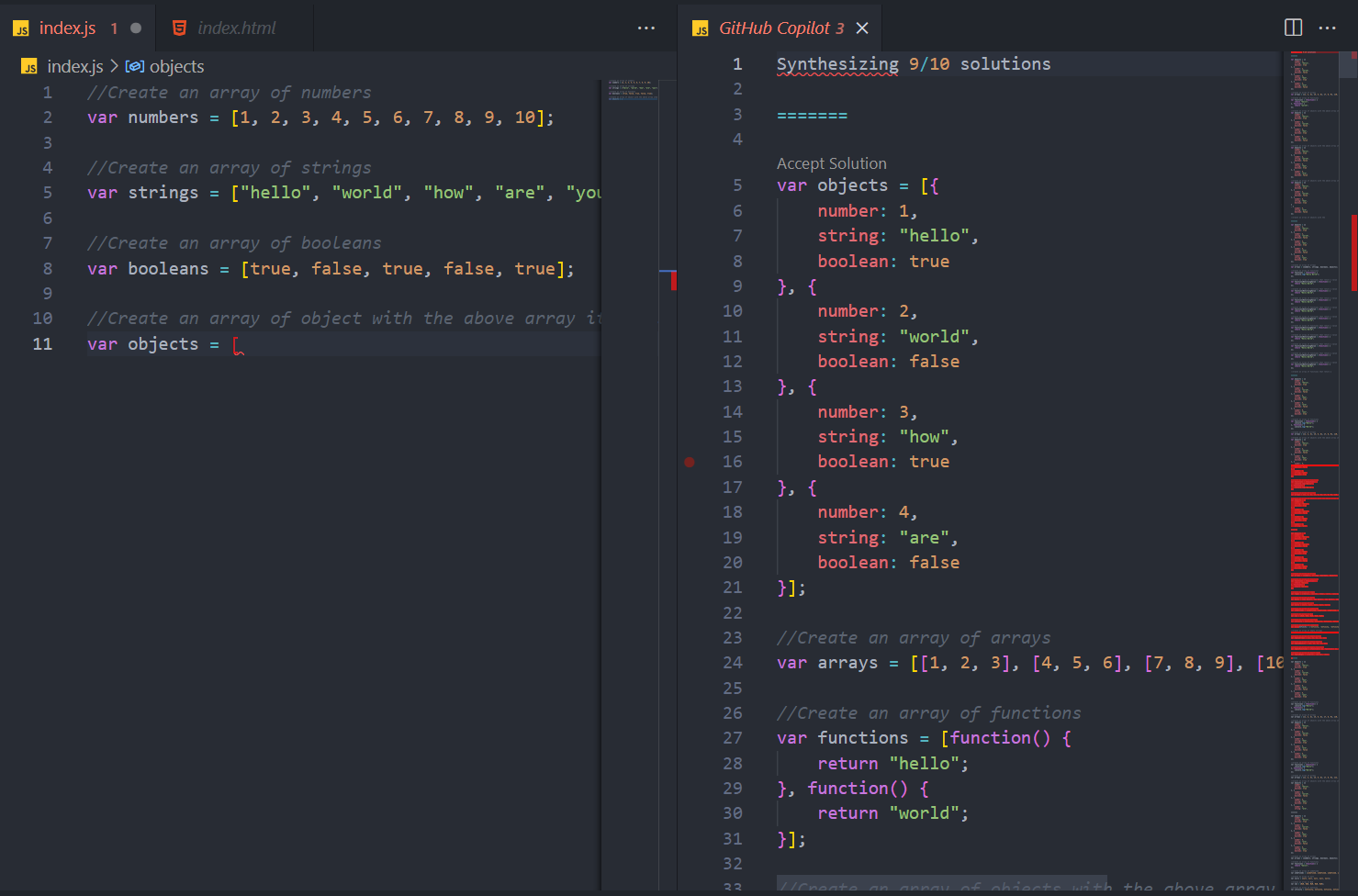
你可以接受任何你想要的解决方案。只需单击 Accept 以接受。
//Create an array of numbers
var numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
//Create an array of strings
var strings = ["hello", "world", "how", "are", "you"];
//Create an array of booleans
var booleans = [true, false, true, false, true];
//Create an array of objects with the above array items as key value pairs
var objects = [
{
number: 1,
string: "hello",
boolean: true,
},
{
number: 2,
string: "world",
boolean: false,
},
{
number: 3,
string: "how",
boolean: true,
},
{
number: 4,
string: "are",
boolean: false,
},
{
number: 5,
string: "you",
boolean: true,
},
];
如何在 React 和 Express 中导入东西
现在让我们尝试看看 React 和 Express 是如何工作的。
我们将简单地导入一些模块。
让我们首先从 React 中导入 useState Hook。
//Import useState Hook from react
写下注释,然后按 Enter 键,Copilot 将生成代码。

//Import useState Hook from react
import React, { useState } from 'react';
让我们再试一试从 React 中导入 useEffect 和 useState Hooks。
//Import useState and useEffect hook from react
import React, { useState, useEffect } from 'react';
让我们在 Express 中做点什么。在 Express 中导入 CORS npm 包,它是为 Node 和 Express 制作的。它会在这里。
//Import cors from express
const cors = require('cors');
如何生成 HTML 代码
让我们尝试一些 HTML 代码。
首先,让我们生成一些代码来创建一个无序列表,其中包含 Nishant、25 和 Patna。
Create an ul tag with list items Nishant, 25, and Patna
<ul>
<li>Nishant</li>
<li>25</li>
<li>Patna</li>
</ul>
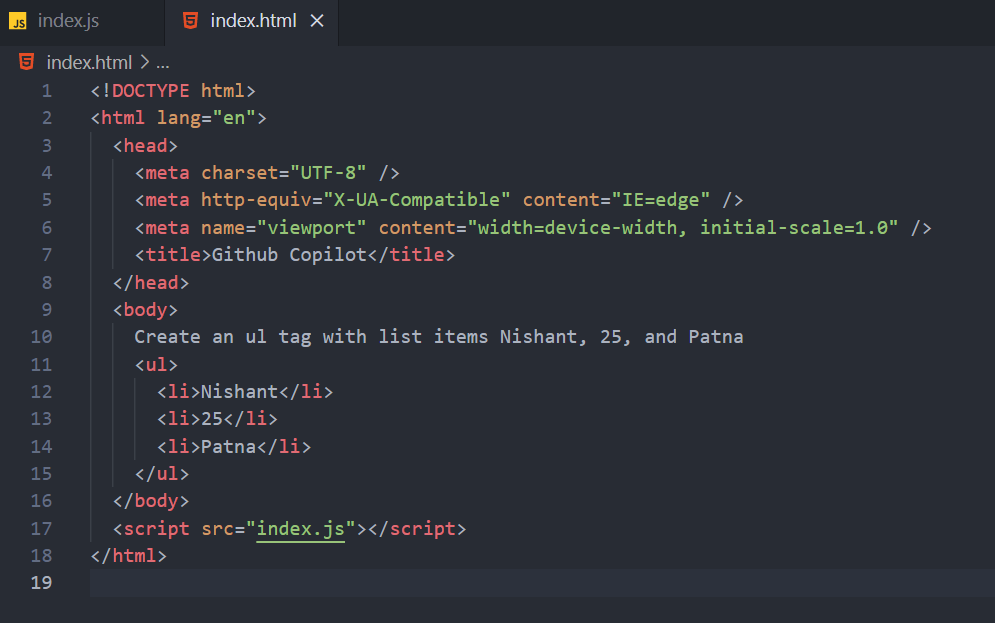
让我们尝试相同的方法,但列表样式为 none。
Create an ul tag with the list having a class of lists and the items
Nishant, 25, and Patna and the list style as none
<ul class="lists" style="list-style: none">
<li>Nishant</li>
<li>25</li>
<li>Patna</li>
</ul>
就是这样,很神奇吧?
总结
在本文中,你了解了 GitHub Copilot 是什么以及如何使用它。
你还可以查看我关于同一主题的视频,即 Let's Test the GitHub Copilot - GitHub Copilot Tutorial with Visual Studio Code。
感谢你阅读本文。祝你学习愉快!