In JavaScript, getting the first element of an array or string is pretty easy. But when it comes to getting the first line of a multiline string, some approaches can hurt your app's performance in certain cases.
In this article, we will look at the three best ways you can get the first line of a multiline string in JS.
For those of you in a hurry, here is the best solution:
input.slice(0, input.indexOf("\n"));
And now if you want to learn more, let's dive deep into the details.
The Problem
The main problem in most solutions is that, in order to simply get the first line of the string, you have to operate over the entire string. If the input is too big, this operation takes an unnecessarily long time and consumes many resources.
Let's explain with an example.
Let's say, I have a gigantic string that contains 10 million lines of text and I want to get the first line of it. So I choose to use the Array.split
method like in the following example:
input.split("\n")[0];
In this code, the split
method operates over the entire 10 million lines to get only the first line. As a result, to get only the first item, I have an array containing 10 million items.
This operation takes approximately 1.7
seconds on my PC. This is unnecessarily long for such a small operation.
Let's look at the solutions to overcome this problem.
The Solutions
After some research, I found the three best ways of doing this operation:
- Using the
String.indexOf
andString.slice
methods. - Using the
Array.split
method. - Using the
String.match
method.
Here are the benchmark results of each solution:
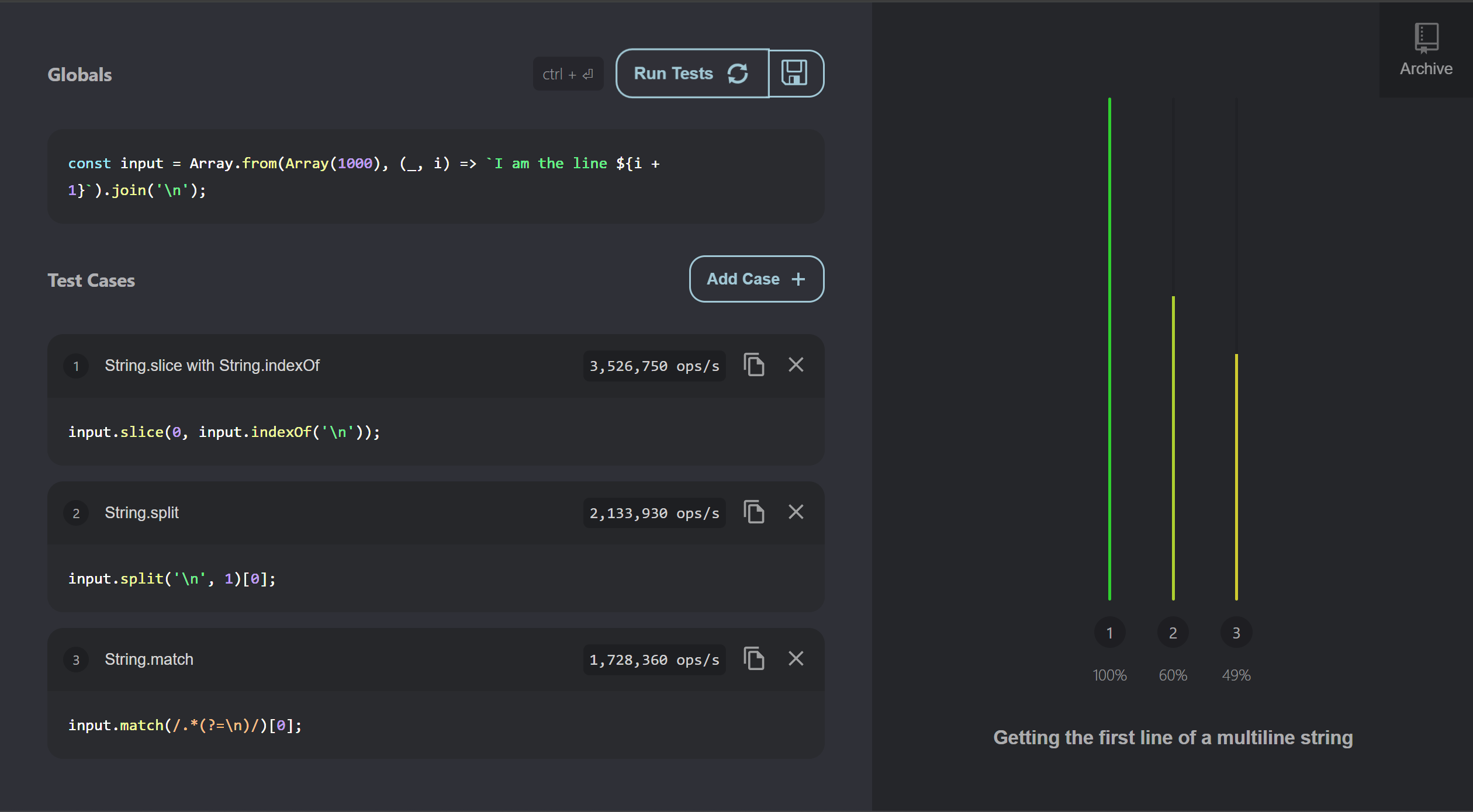
Also, you can access the benchmark results from this link and perform benchmarks yourself, too.
Let's explore each solution separately.
1. Using the String.indexOf
and String.slice
Methods
In this solution, we will use the String.indexOf
method to find the index of the new line (\n
) character, and then will slice the string to this index with the String.slice
method.
input.slice(0, input.indexOf("\n"));
This solution takes first place in the benchmark results.
2. Using the Array.split
Method
As you have seen in the problem, the Array.split
method operates over the entire string. But it also accepts an optional limit
parameter. We can leverage this parameter to avoid unnecessary operations.
input.split("\n", 1)[0];
In this code, we took advantage of the optional limit
parameter and so the operation stops after the first split operation.
This solution takes second place in the benchmark results.
3. Using the String.match
Method
This approach is for those of you who particularly want to use regex.
We will use the String.match
method in this case. We will create a regex literal that matches with any character group which is followed by a new line character, and pass it to the method. The first element of the returned array (which is the match) is the first line.
input.match(/.*(?=\n)/)[0];
This solution takes third place in the benchmark results and performs two times slower than the first solution.
According to the MDN:
Before regular expressions can be used, they have to be compiled.
The performance downgrade is probably because of that.
Conclusion
In this article, we have looked at three different approaches to get the first line of a multiline string.
As developers, even for such a small operation, we should consider edge cases to avoid potential problems in the future.
Thank you for reading. You can connect with me on Twitter or explore more on my personal blog. Feel free to reach out — I'd love to hear from you!