freeCodeCamp just launched a major upgrade to our JavaScript Algorithms and Data Structures Certification. You can now learn JavaScript by building 21 projects!
Instead of coding through a series of challenges, you will learn the fundamentals of JavaScript by building 16 practice projects + 5 certification projects. Each of the practice projects will lead you through a step by step process for building out dynamic HTML, CSS and JavaScript applications.
Also note that any older certifications you have earned will continue to remain valid going forward.
I have created a video walkthrough demoing each of the projects that you will build throughout this course.
Here is the complete list of projects you will build:
- Learn Basic JavaScript by Building a Role Playing Game
- Learn Form Validation by Building a Calorie Counter
- Learn Basic String and Array Methods by Building a Music Player
- Learn the Date Object By Building a Date Formatter
- Build a Palindrome Checker - Certification project
- Learn Modern JavaScript Methods By Building Football Team Cards
- Learn localStorage by Building a Todo List
- Learn Recursion by Building a Decimal to Binary Converter
- Build a Roman Numeral Converter - Certification project
- Learn Basic Algorithmic Thinking by Building a Number Sorter
- Learn Advanced Array Methods by Building a Statistics Calculator
- Learn Functional Programming by Building a Spreadsheet
- Learn Regular Expressions by Building a Spam Filter
- Build a Telephone Number Validator - Certification Project
- Learn Basic OOP by Building a Shopping Cart
- Learn Intermediate OOP by Building a Platformer Game
- Learn Intermediate Algorithmic Thinking by Building a Dice Game
- Build a Cash Register - Certification Project
- Learn Fetch and Promises by Building an fCC Authors Page
- Learn Asynchronous programming by Building an fCC Forum Leaderboard
- Build a Pokémon Search App - Certification Project
Learn Basic JavaScript by Building a Role Playing Game
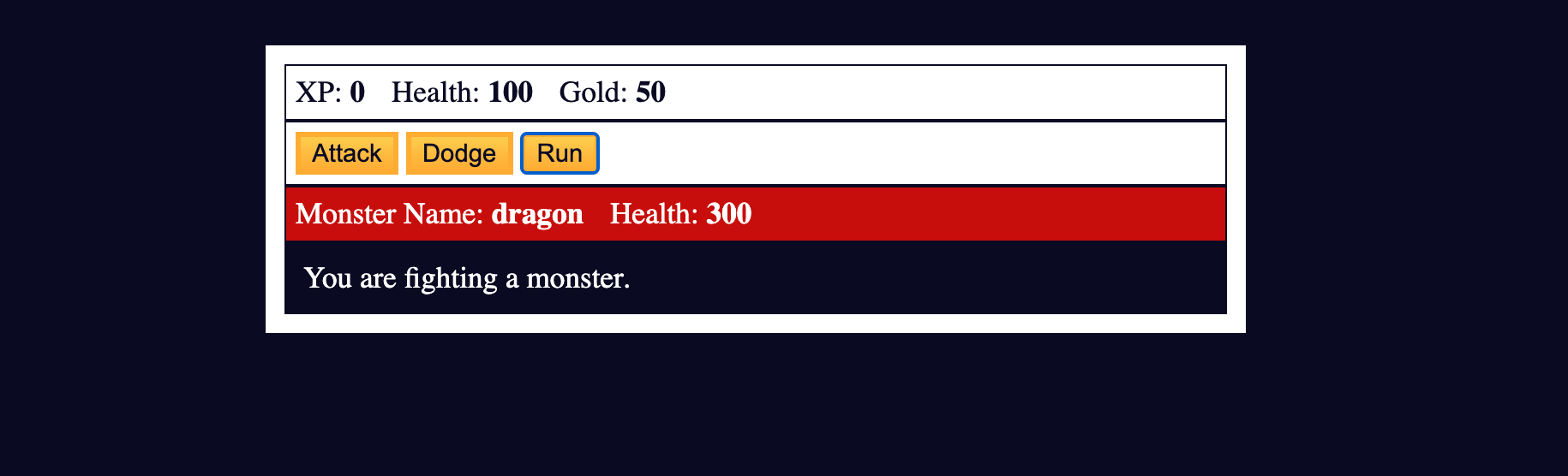
For the first project, you'll learn fundamental programming concepts in JavaScript by coding your own Role Playing Game. You'll learn how to work with arrays, strings, objects, functions, loops, if/else statements, and more.
You will start off by building the HTML and CSS structure inside the freeCodeCamp editor. Then you will move onto building out the JavaScript functionality.
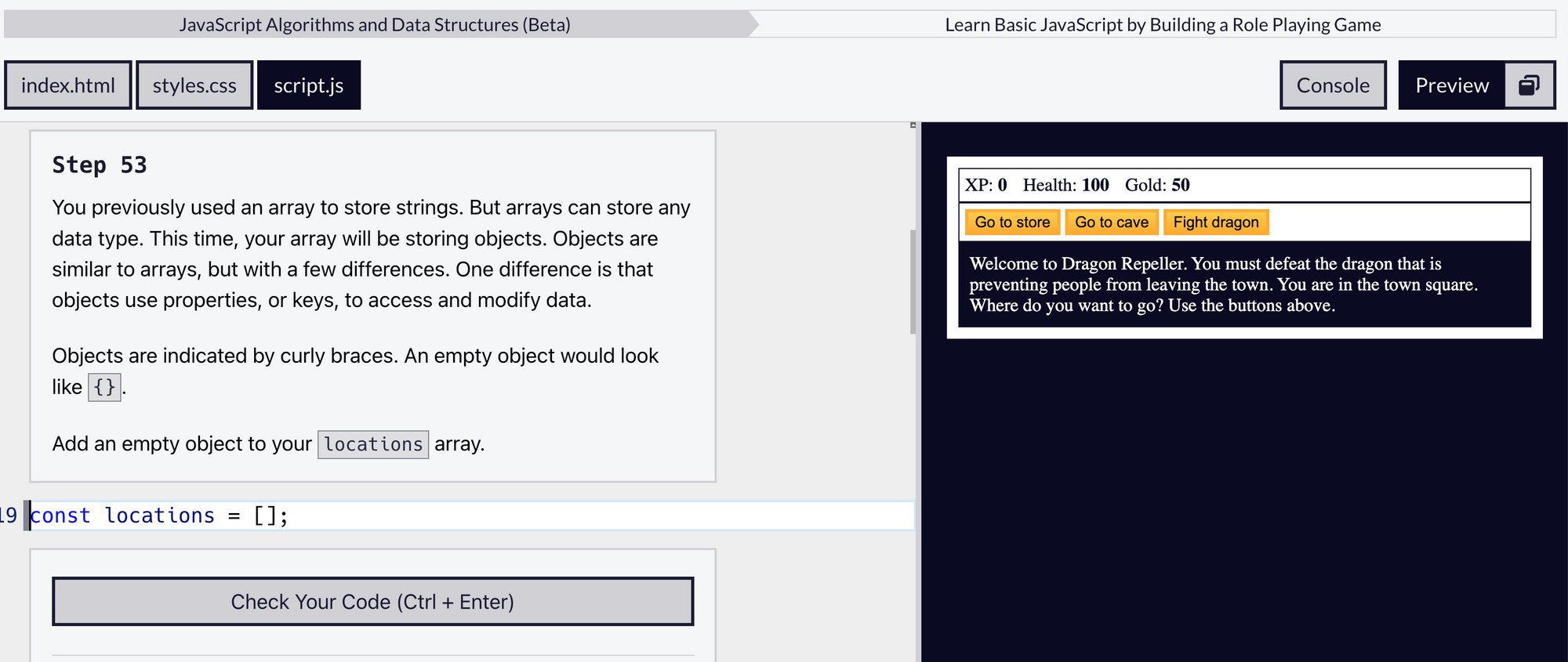
This project will give you tons of repetition and practice working with the basics of JavaScript. And if you get stuck on a step, then you will be guided to the solution through a series of hints.
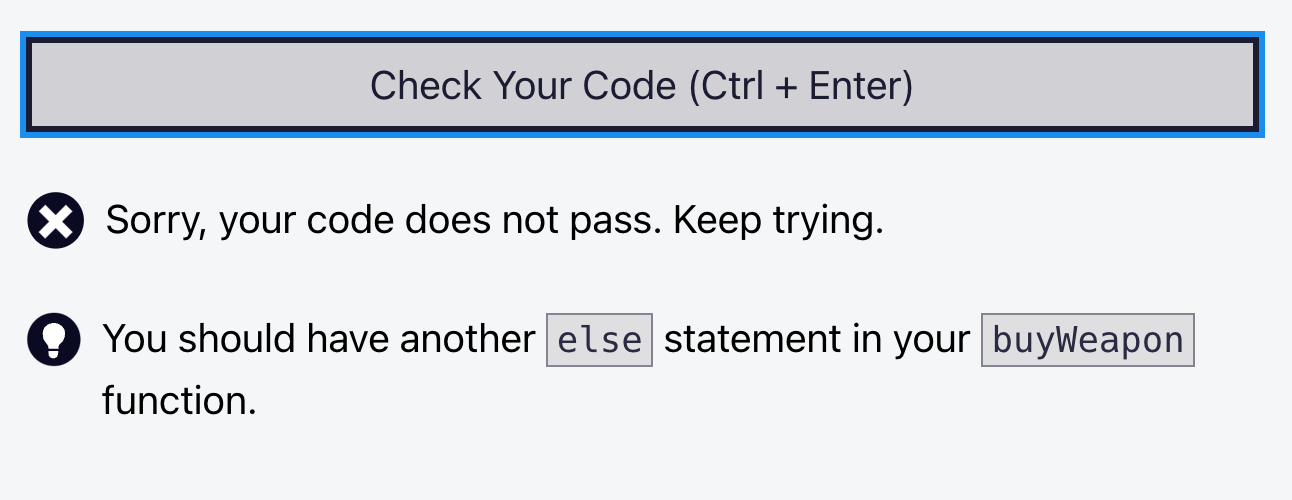
Learn Form Validation by Building a Calorie Counter
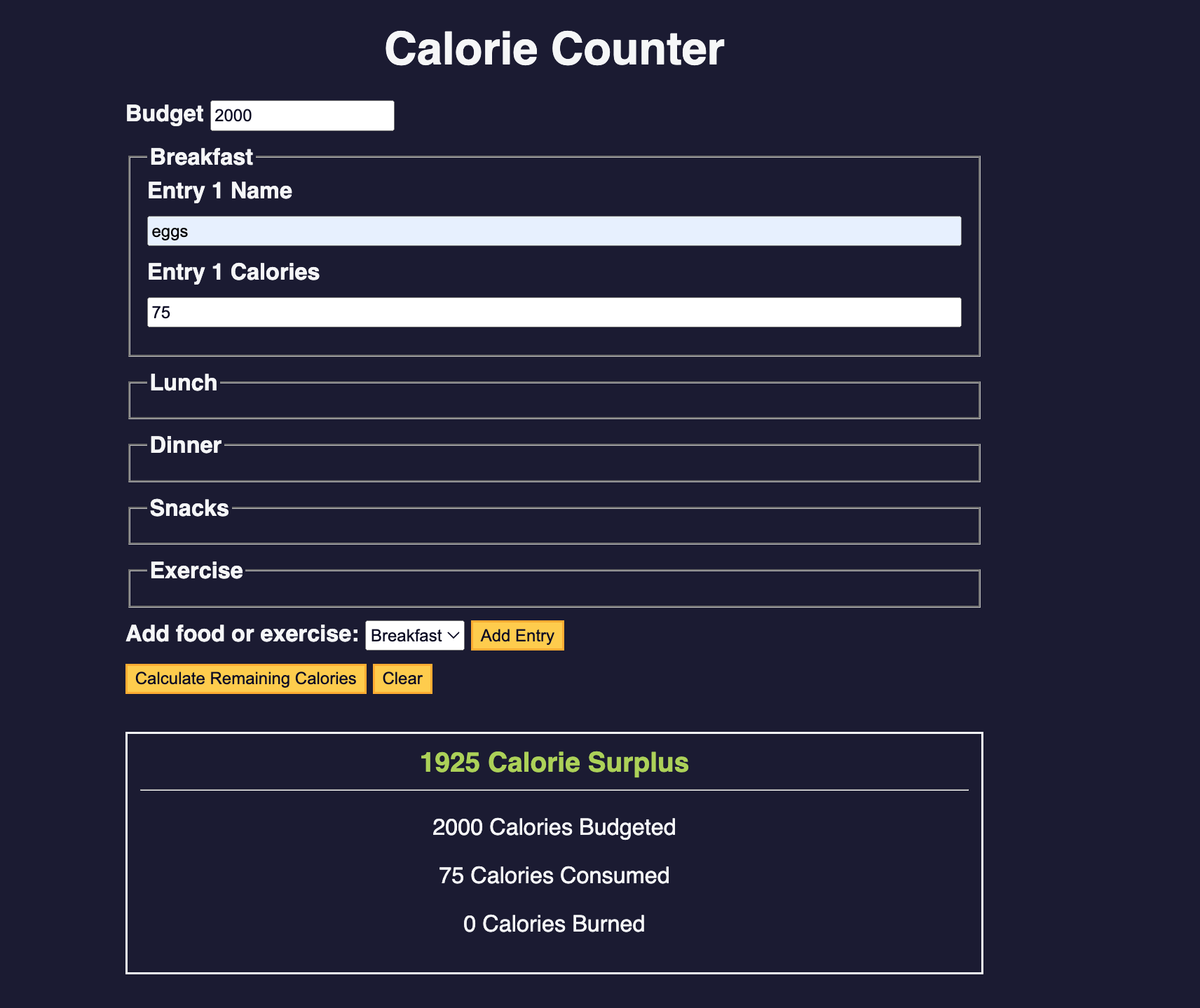
In this calorie counter project, you’ll learn how to validate user input, perform calculations based on that input, and dynamically update your interface to display the results. This project covers basic regular expressions, template literals, the addEventListener()
method, and more.
Learn Basic String and Array Methods by Building a Music Player
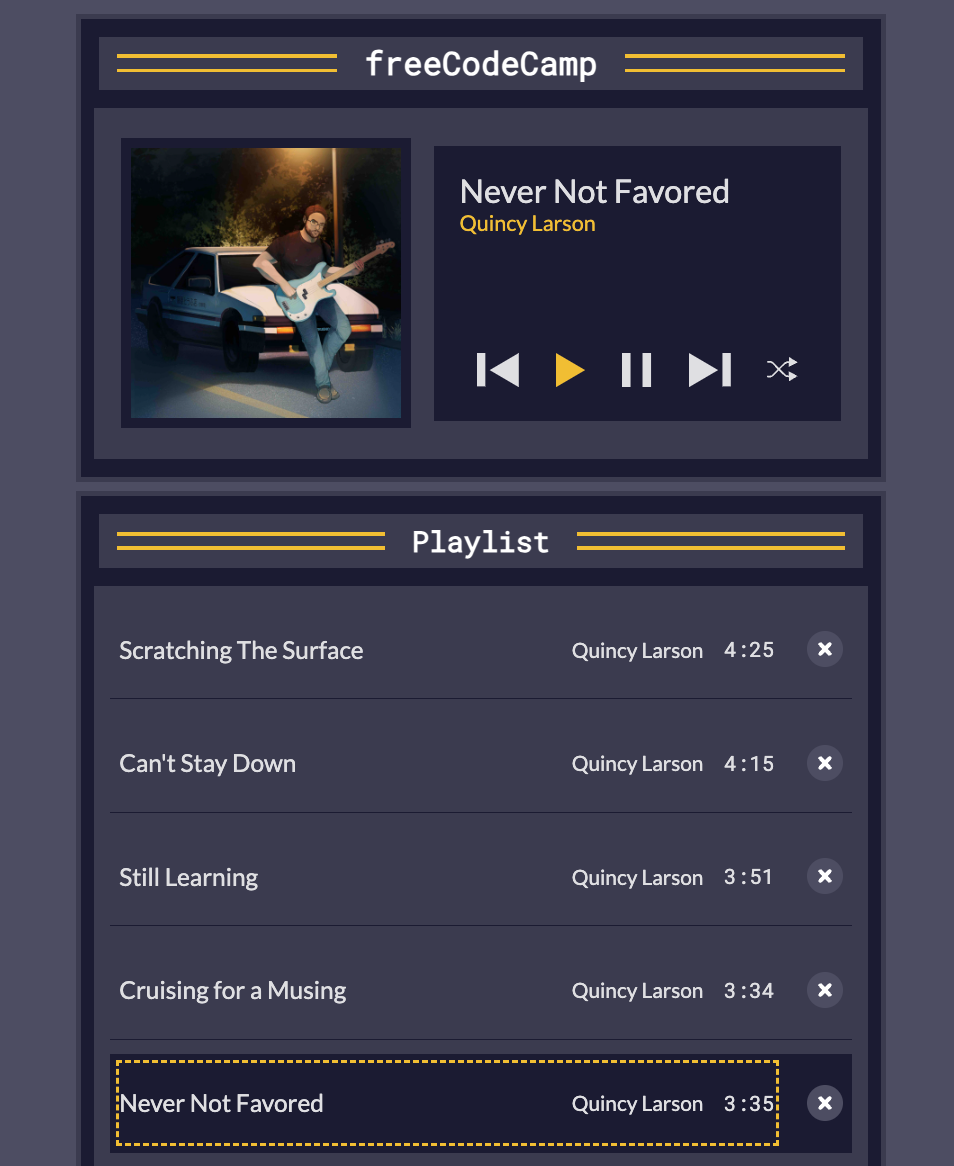
In this project, you’ll code a basic MP3 player using HTML, CSS, and JavaScript. The project covers fundamental concepts such as handling audio playback, managing a playlist, implementing play, pause, next, previous, and shuffle functionalities.
You will learn how to work with essential string and array methods like the find()
, forEach()
, map()
, and join()
.
Learn the Date Object By Building a Date Formatter
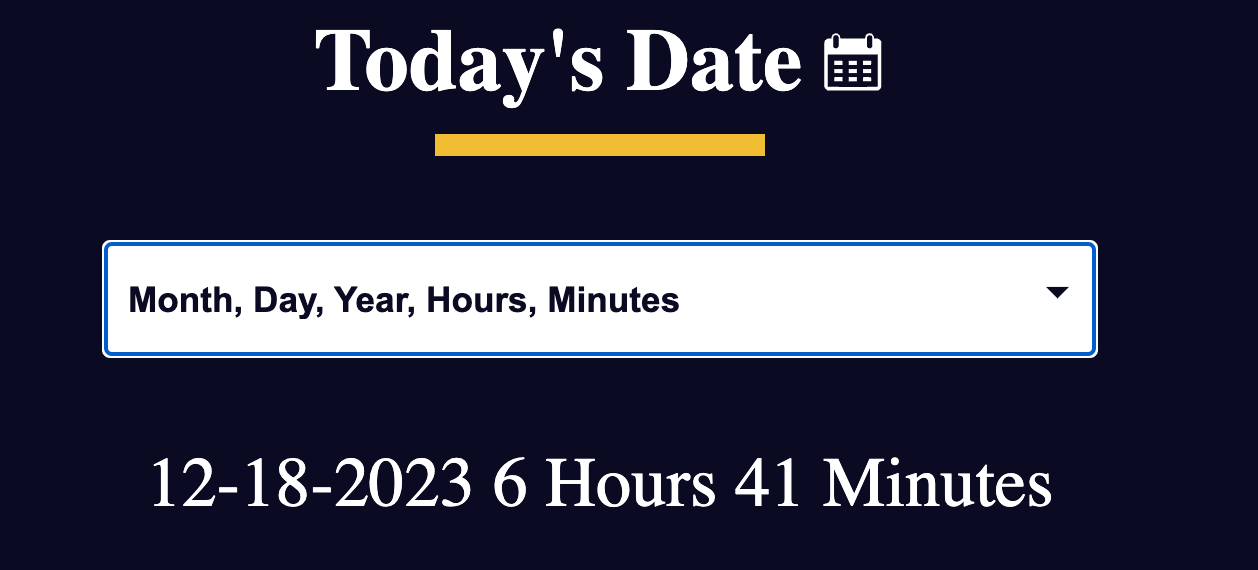
In this project, you’ll learn how to work with the JavaScript Date
object, including its methods and properties. You’ll also learn how to correctly format dates.
This project will cover concepts such as the getDate()
, getMonth()
, and getFullYear()
methods.
Certification project: Build a Palindrome Checker
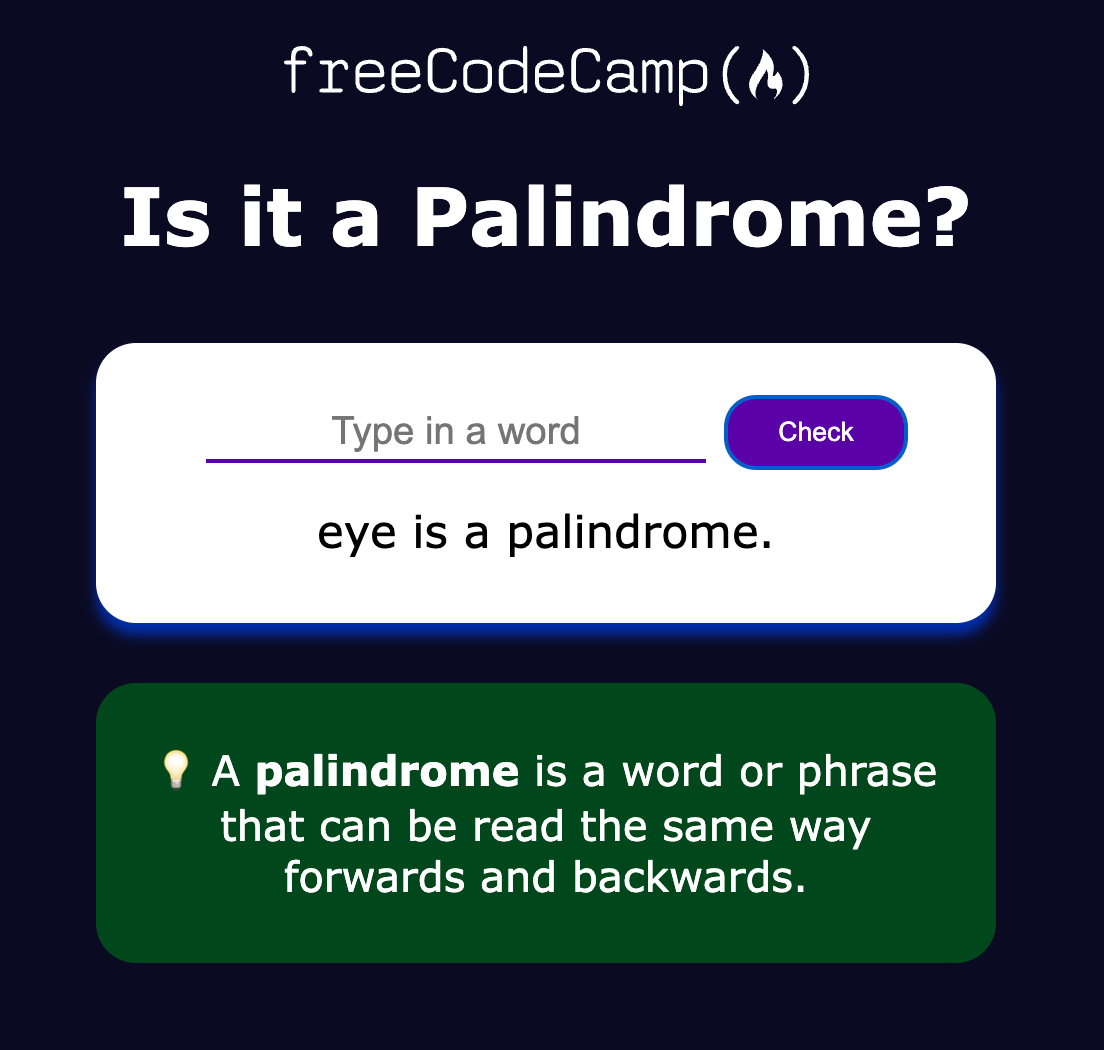
For this certification project, you will build out a palindrome checker. A user should be able to input a word or phrase and your application should correctly check if is a palindrome.
A palindrome is a word or phrase that can be read the same way forwards and backwards, ignoring punctuation, case, and spacing.
This is one of the five certification projects that count towards earning the JavaScript Algorithms and Data Structures Certification.
Learn Modern JavaScript Methods By Building Football Team Cards

In this football team cards project, you'll learn how to work with DOM manipulation, object destructuring, event handling, and data filtering.
This project will cover concepts like switch
statements, default parameters, Object.freeze()
, the map()
method, and more.
Learn localStorage by Building a Todo List
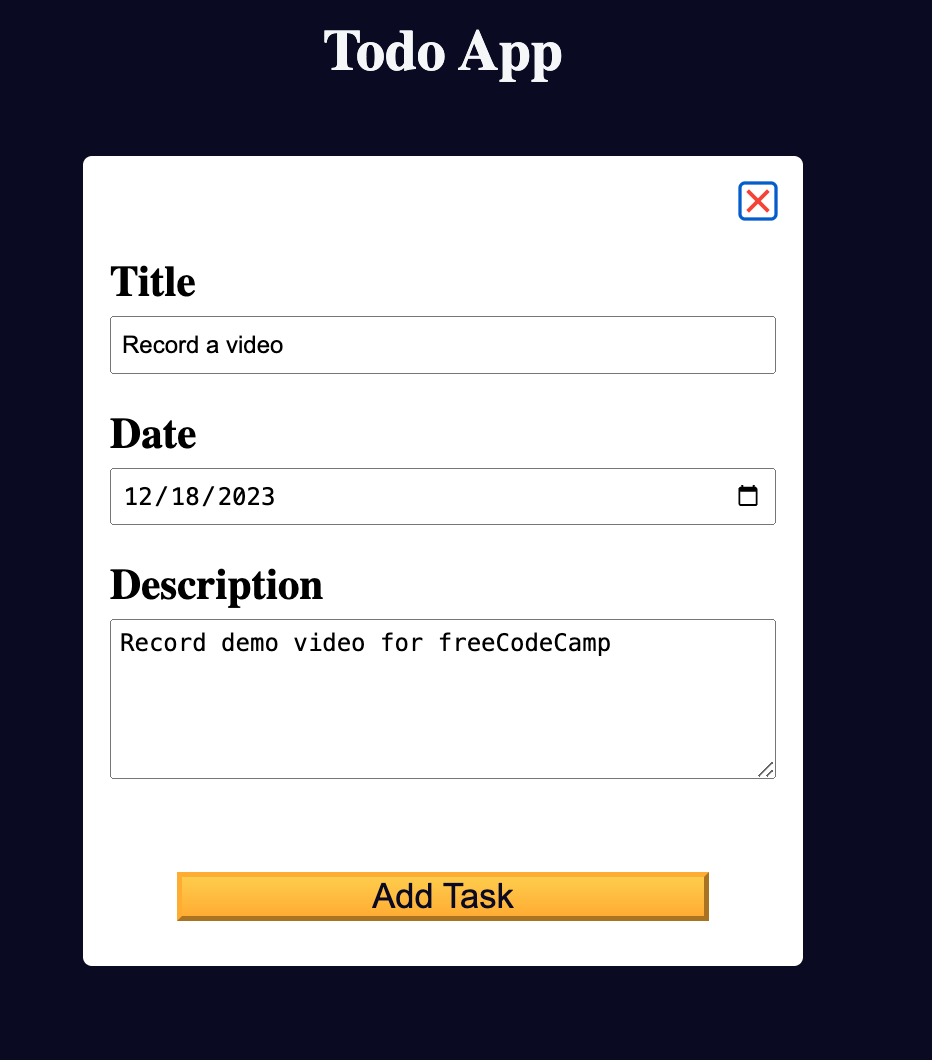
Local storage is a web browser feature that lets web applications store key-value pairs persistently within a user's browser. This allows web apps to save data during one session, then retrieve it in a later page session.
In this TODO application, you’ll learn how to handle form inputs, manage local storage, perform CRUD (Create, Read, Update, Delete) operations on tasks, implementing event listeners, and toggle UI elements.
Learn Recursion by Building a Decimal to Binary Converter

Recursion is a programming concept where a function calls itself. This can reduce a complex problem into simpler sub-problems, until they become straightforward to solve.
In this project, you’ll build a decimal-to-binary converter using JavaScript. You’ll learn the fundamental concepts of recursion, explore the call stack, and build out a visual representation of the recursion process through an animation.
Certification Project: Build a Roman Numeral Converter

For this certification project, you will build out a Roman numeral converter. A user should be able to input an integer and your application should correctly return a Roman numeral.
This is one of the five certification projects that count towards earning the JavaScript Algorithms and Data Structures Certification.
Learn Basic Algorithmic Thinking by Building a Number Sorter

In computer science, there are fundamental sorting algorithms that all developers should learn. In this number sorter project, you’ll learn how to implement and visualize different sorting algorithms like bubble sort, selection sort, and insertion sort – all with JavaScript.
This project will help you understand the fundamental concepts behind these algorithms, and how you can apply them to sort numerical data in web applications.
Learn Advanced Array Methods by Building a Statistics Calculator
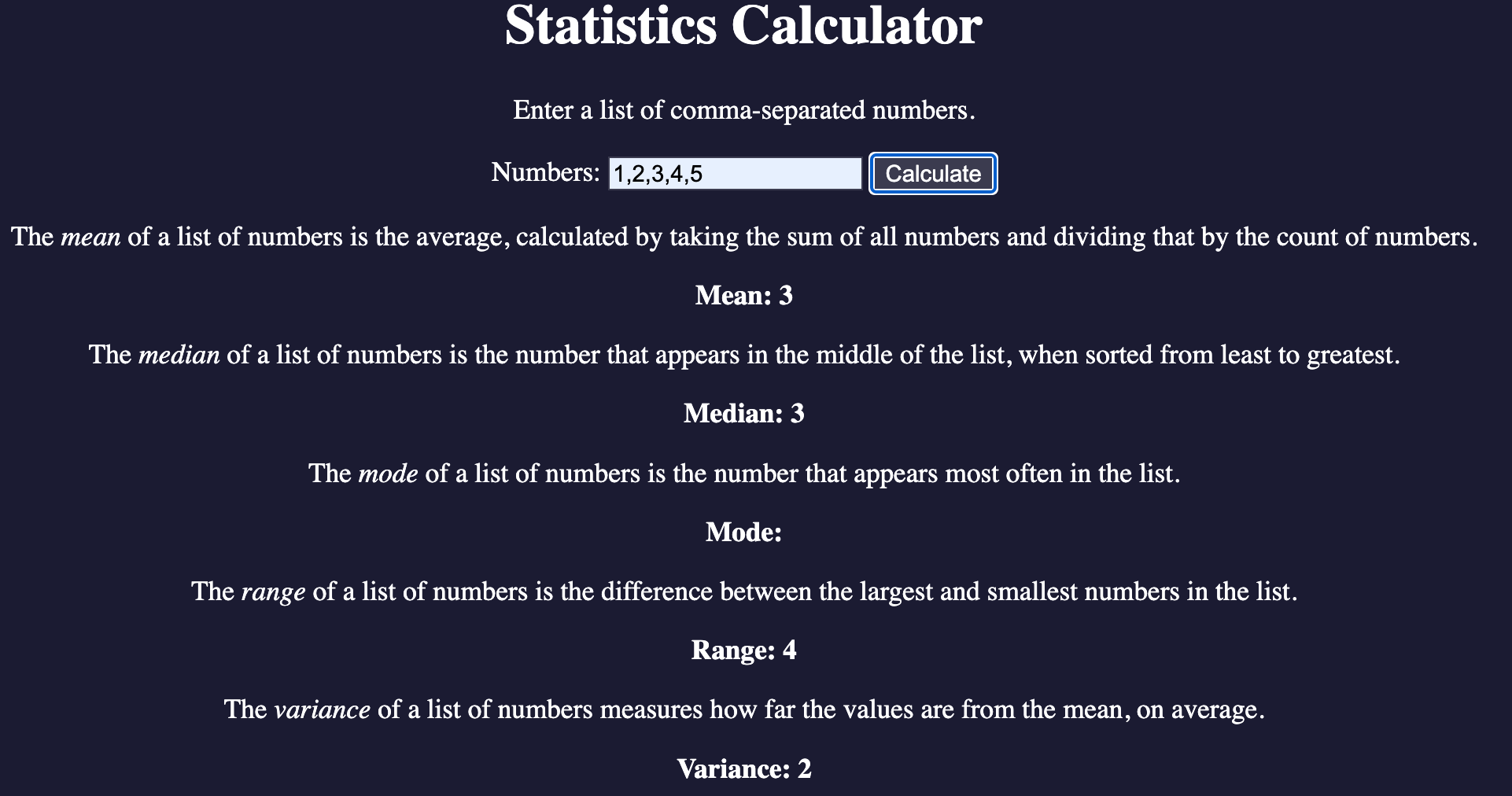
In this statistics calculator project, you'll gain experience with handling user input, DOM manipulation, and method chaining. You’ll get practice by performing statistical calculations like mean, median, mode, variance, and standard deviation.
Learn Functional Programming by Building a Spreadsheet

In Functional Programming, developers organize code into smaller functions, then combine those functions to build complex programs.
In this spreadsheet application project, you'll learn about parsing and evaluating mathematical expressions, implementing spreadsheet functions, handling cell references, and creating interactive web interfaces. You’ll learn how to dynamically update the page based on user input.
This project will cover concepts like the map()
method, find()
method, parseInt()
, the includes()
method.
Learn Regular Expressions by Building a Spam Filter
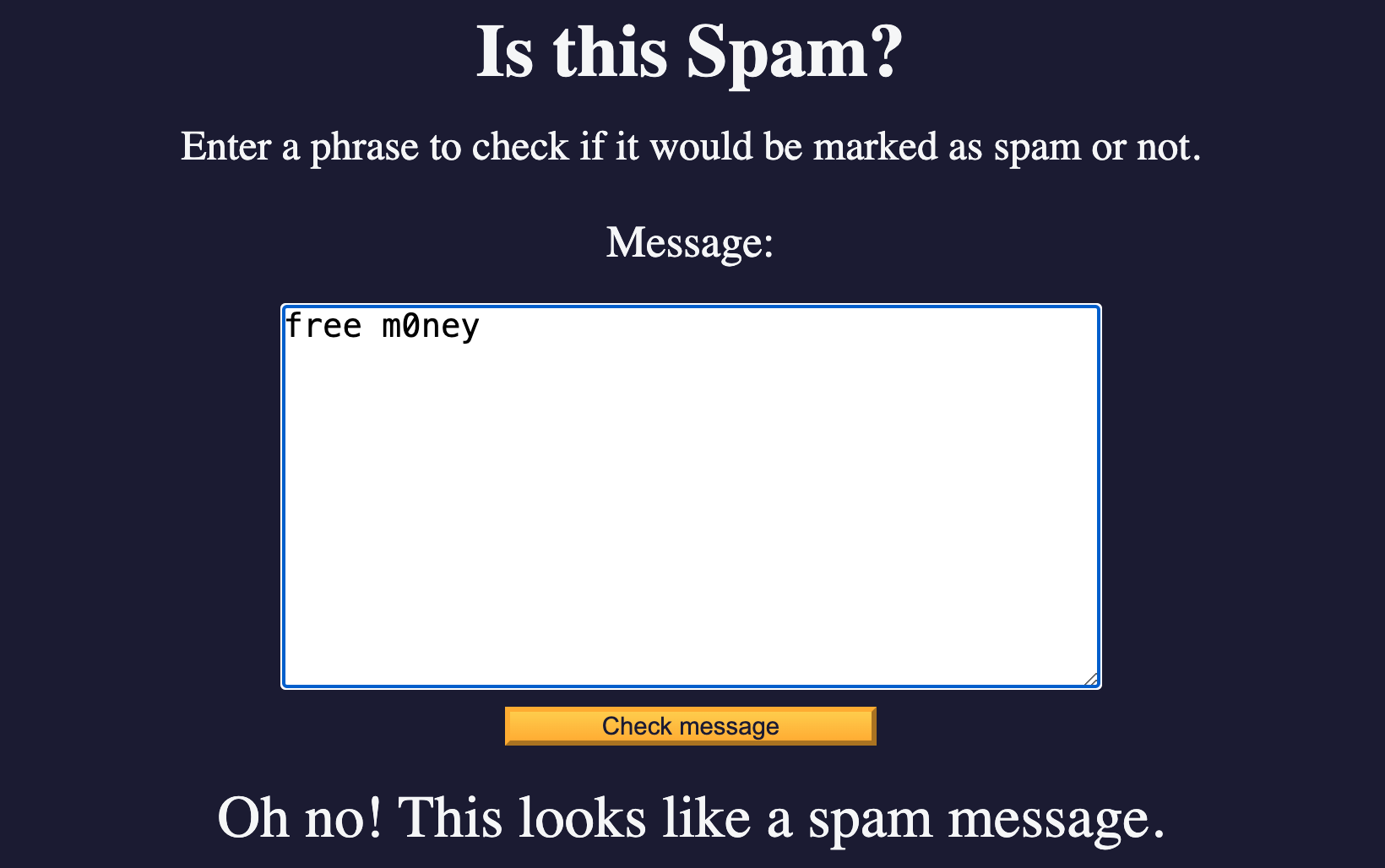
Regular expressions, often shortened to "regex" or "regexp", are patterns that help programmers match, search, and replace text. Regular expressions are powerful, but can be difficult to understand because they use so many special characters.
In this spam filter project, you'll learn about capture groups, positive lookaheads, negative lookaheads, and other techniques to match any text you want.
Certification Project: Telephone Number Validator
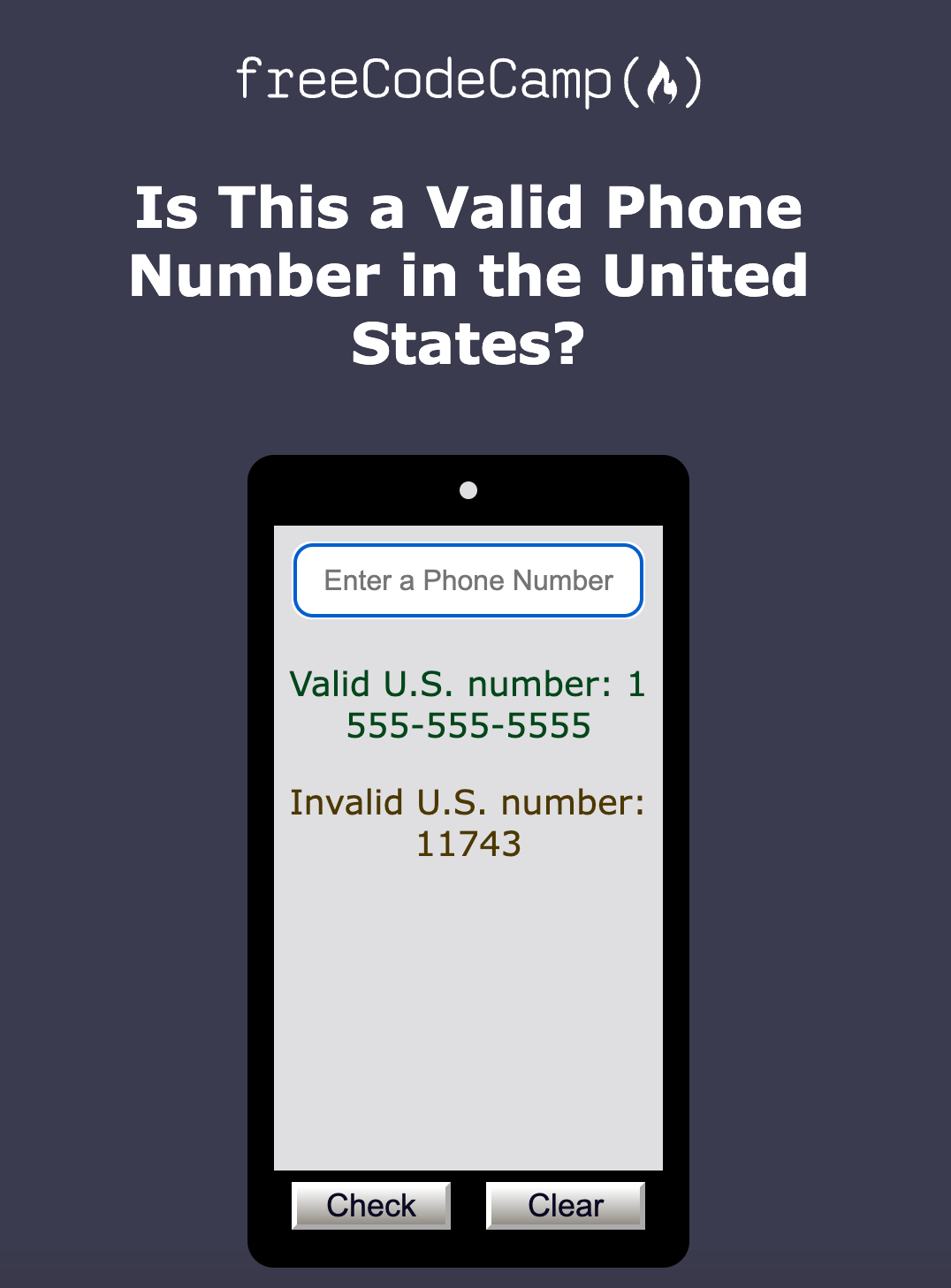
For this certification project, you will build out a telephone number validator. A user should be able to input a number or random set of characters and your application should correctly check if it is a valid United States phone number or not.
This is one of the five certification projects that count towards earning the JavaScript Algorithms and Data Structures Certification.
Learn Basic OOP by Building a Shopping Cart

OOP, or Object Oriented Programming, is one of the major approaches to the software development process. In OOP, developers use objects and classes to structure their code.
In this shopping cart project, you'll learn how to define classes and use them. You’ll create class instances and implement methods for data manipulation.
This project will cover concepts like the ternary
operator, the spread
operator, the this
keyword, and more.
Learn Intermediate OOP by Building a Platformer Game
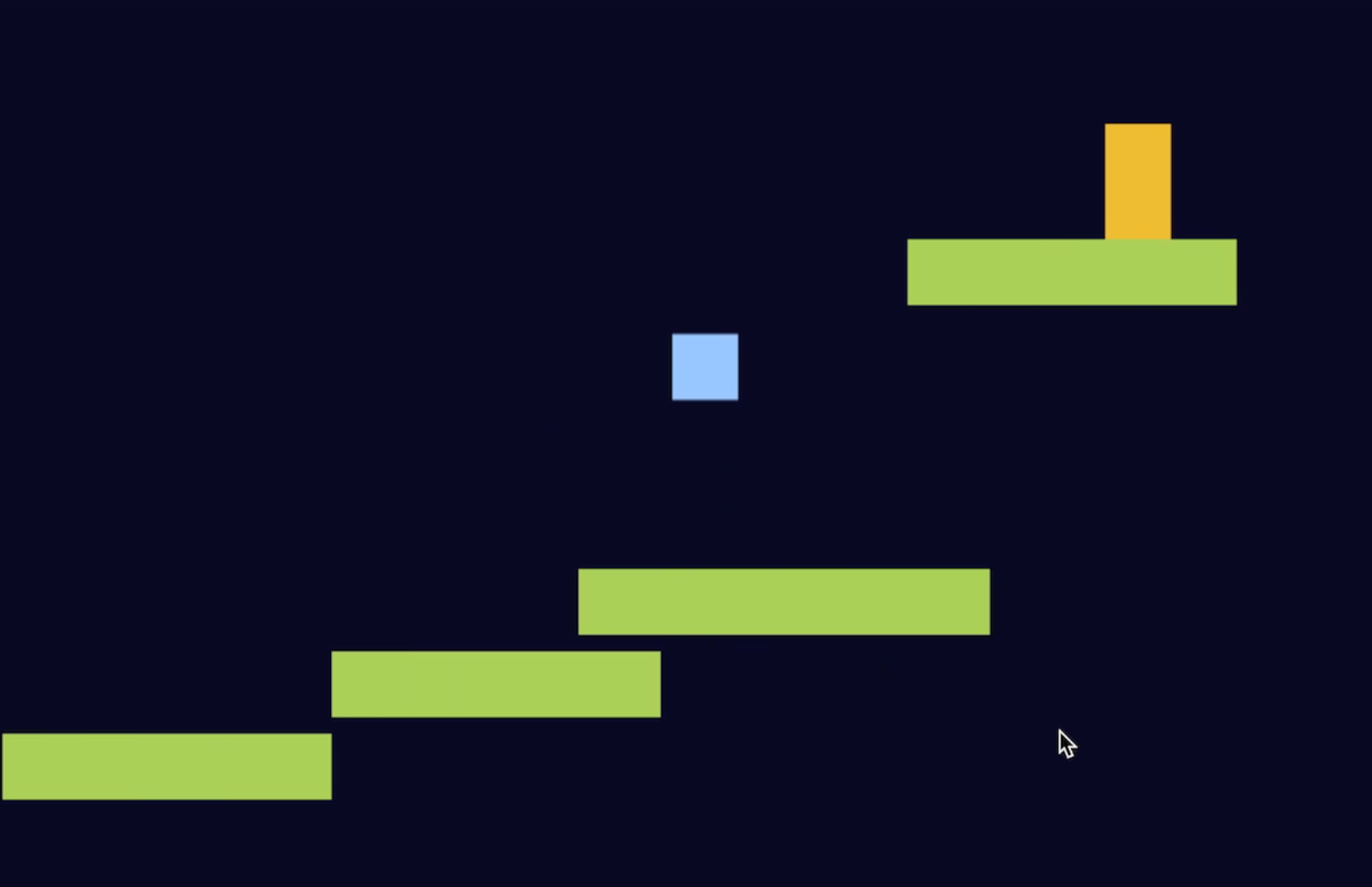
In this platformer game project, you’ll continue to learn about classes, objects, inheritance, and encapsulation. You’ll also learn how to design and organize game elements efficiently and gain insights into problem-solving and code reusability.
Learn Intermediate Algorithmic Thinking by Building a Dice Game
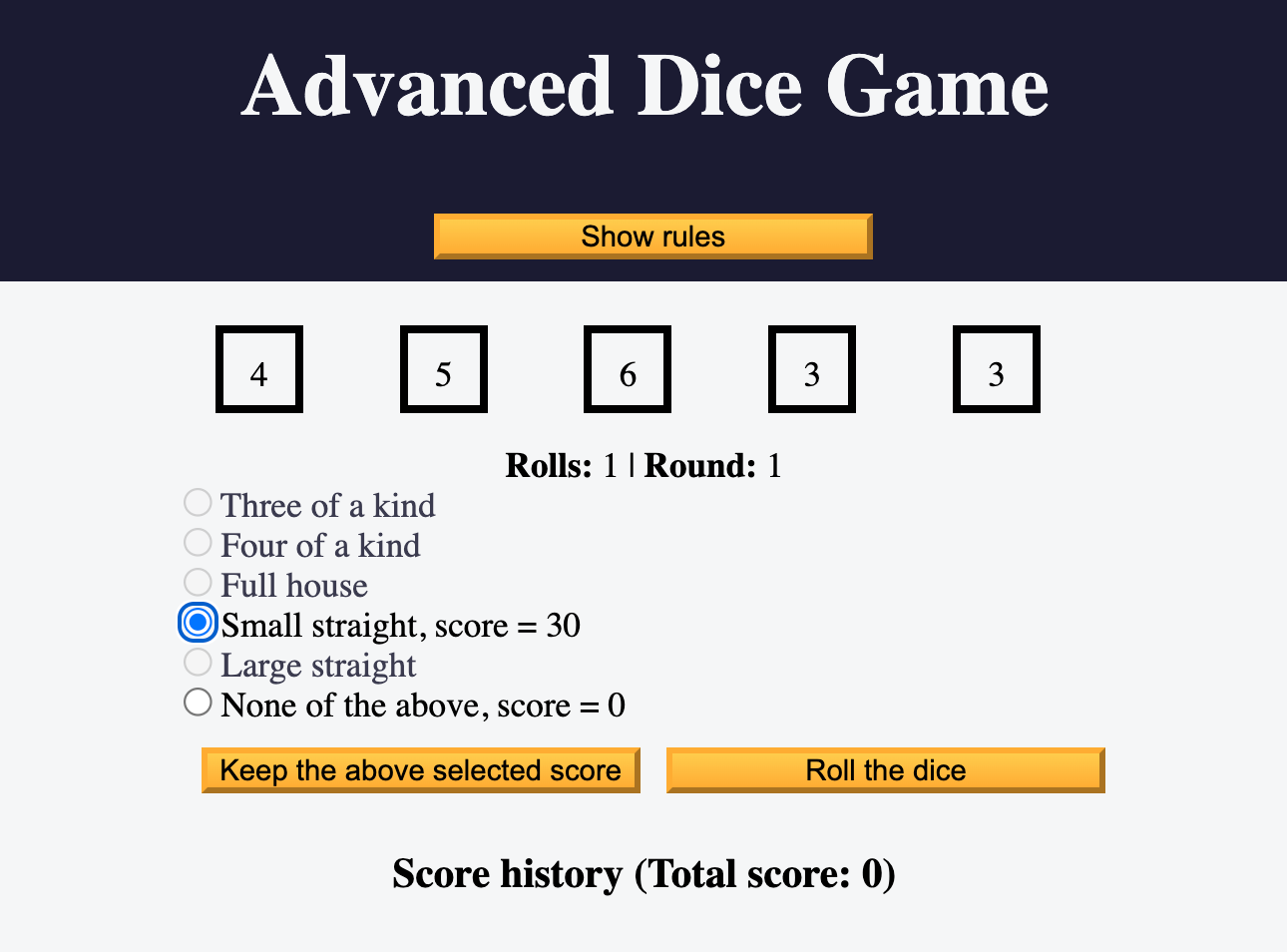
Algorithmic thinking involves the ability to break down complex problems into a sequence of well-defined, step-by-step instructions.
In this Dice game project, you’ll learn how to manage game state, implement game logic for rolling dice, keeping score, and applying rules for various combinations.
This project covers concepts such as event handling, array manipulation, conditional logic, and updating the user interface dynamically based on game state.
Certification Project: Build a Cash Register
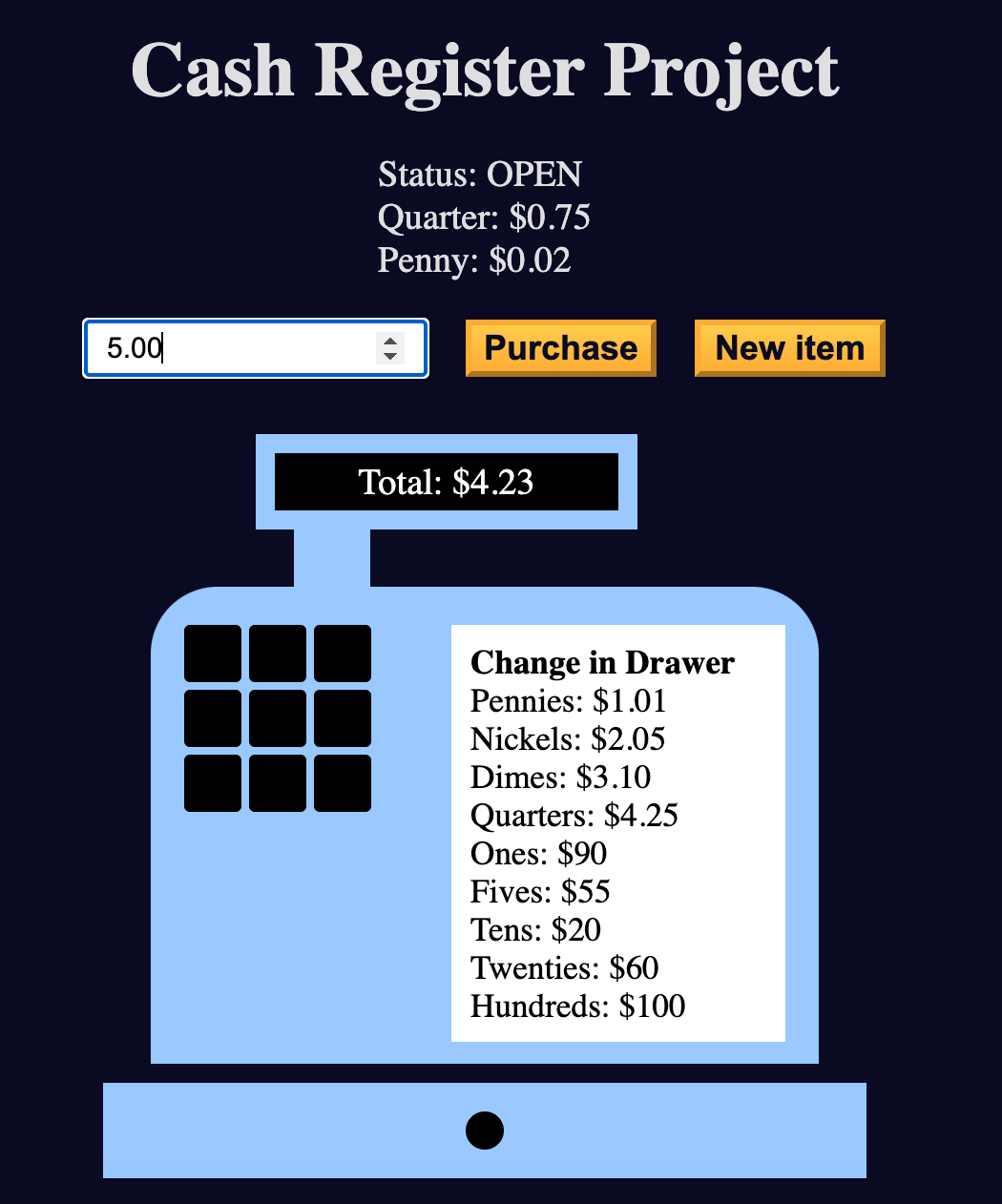
For this certification project, you will build out a functioning cash register. A user should be able to input cash and the register should output if any change is due and what amount.
This is one of the five certification projects that count towards earning the JavaScript Algorithms and Data Structures Certification.
Learn Fetch and Promises by Building an fCC Authors Page

One common aspect of web development is learning how to fetch data from an external API, then work with asynchronous JavaScript.
This freeCodeCamp authors page project will show you how to use the fetch method, then dynamically update the DOM to display the fetched data. This project will also teach you how to paginate your data so you can load results in batches.
Learn Asynchronous Programming by Building an fCC Forum Leaderboard
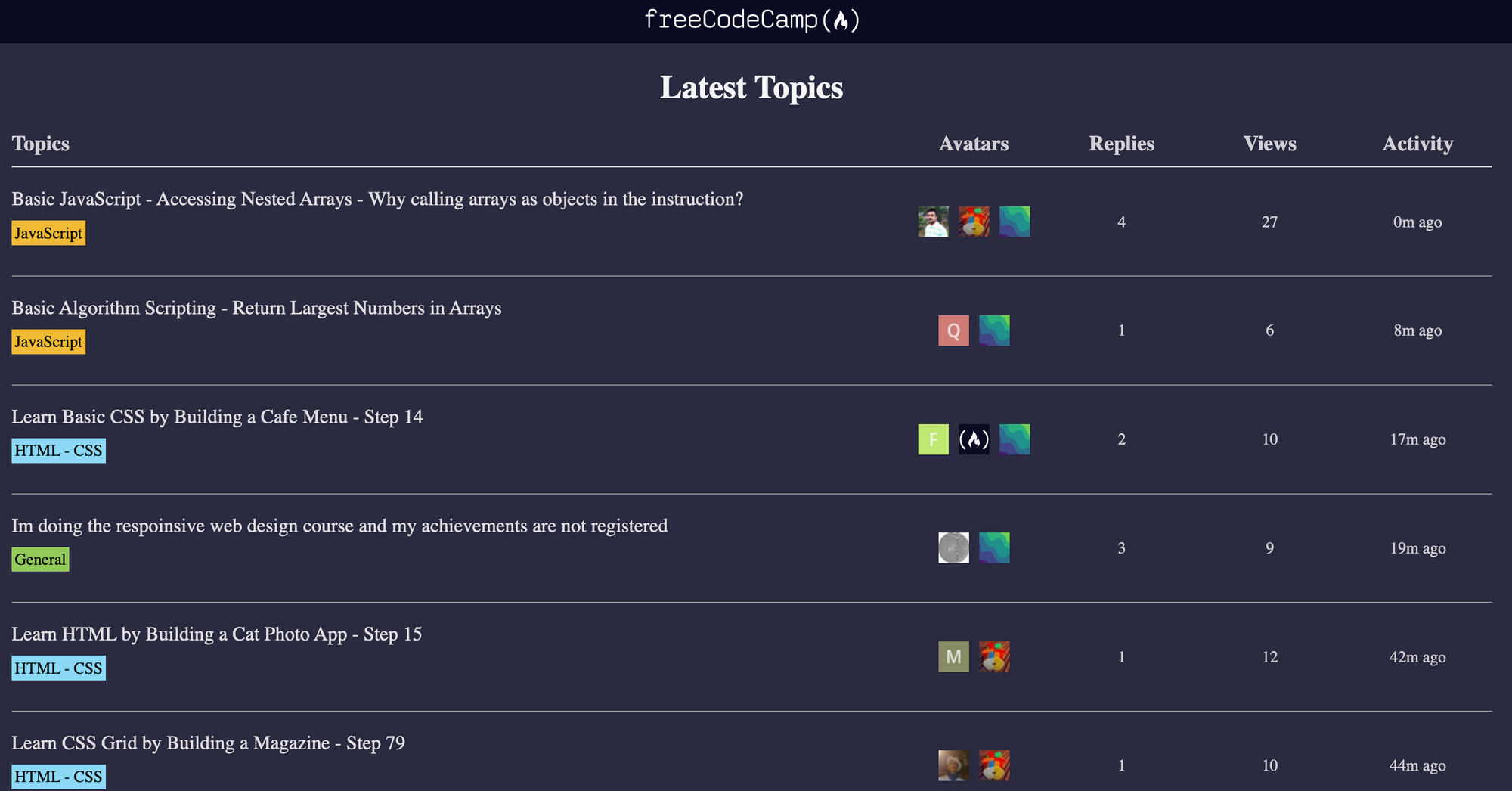
In this project you will gain proficiency in asynchronous concepts by building your own freeCodeCamp forum leaderboard. This project will cover the Fetch API
, promises, Async/Await
, and the try...catch
statement.
Certification Project: Build a Pokémon Search App
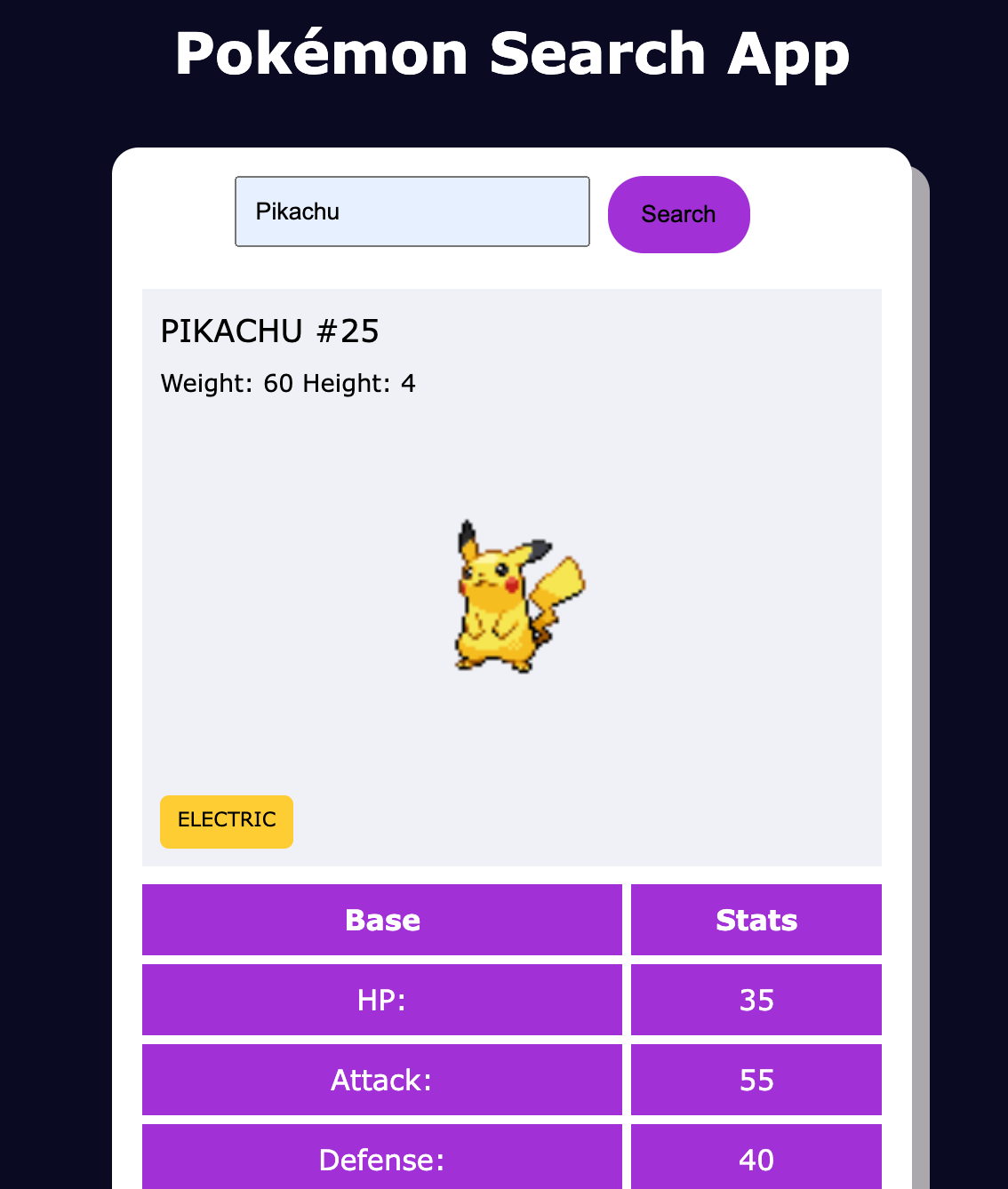
For this certification project, you will build out a Pokémon Search App. A user should be able to enter in the name or id for a Pokémon and your application should return the stats and avatar for that Pokémon.
This is one of the five certification projects that count towards earning the JavaScript Algorithms and Data Structures Certification.
Try the New JavaScript Algorithms and Data Structures Certification
This certification is currently in beta. If you find any issues, please report them to our GitHub repository.
If you need help with any of the projects, reach out on the freeCodeCamp forum.
When you are ready, you can dive right into the new JavaScript Algorithms and Data Structures Certification.
This is only the beginning
We'll be working throughout 2024 and 2025 to help as many developers as possible improve their JavaScript skills.
You can get involved in our open source efforts by becoming a volunteer in the freeCodeCamp community.
And if you want to help us speed up development of our JavaScript curriculum, and the many other initiatives we have underway, you should become a monthly supporter of our charity.
Happy coding. 🏕️