In JavaScript, every
and some
help you test if something is true for every element or some elements of an array.
In this article, I'll show you how to use these helpful array methods.
Table of Contents
- 1How
every()
andsome()
Work – an Overview - 2Parameters of
every
andsome
- 3Edge cases for
every
andsome
- 4A challenge for you
1How every()
and some()
Work – an Overview#
First we need some data to test. For simplicity let's consider an array of numbers:
const nums = [34, 2, 48, 91, 12, 32];
Now let's say we want to test if every number in the array is less than 100
. Using every
we can easily test it like below:
nums.every(n => n < 100);
// true
Short and sweet! You can think about what happens here like this:
every
loops over the array elements left to right.- For each iteration, it calls the given function with the current array element as its 1st argument.
- The loop continues until the function returns a falsy value. And in that case
every
returnsfalse
– otherwise it returnstrue
.
some
also works very similarly to every
:
some
loops over the array elements left to right.- For each iteration, it calls the given function with the current array element as its 1st argument.
- The loop continues until the function returns a truthy value. And in that case
some
returnstrue
– otherwise it returnsfalse
.
Now let's use some
to test if some number in the array is odd:
nums.some(n => n % 2 == 1);
// true
That's really true! 91
is odd.
But this is not the end of the story. These methods have some more depth. Let's dig in.
2Parameters of every
and some
#
The way to use every
and some
array methods is exactly the same. They have the same set of parameters and those parameters also mean identical things. So it's very easy to learn them at once.
We have already worked with first parameter of these methods which is a function. We call this function predicate.
In computer science, a predicate is a function of a set of parameters that returns a boolean as an answer. JavaScript treats the function we give toevery
/some
as a predicate. We can return any type of value we wish, but those are treated as a Boolean, so it's common to call this function a predicate.
They also have an optional 2nd parameter to control this
inside of non-arrow predicates. We call it thisArg
.
So you can call these methods in the following ways:
arr.every(predicate)
arr.every(predicate, thisArg)
arr.some(predicate)
arr.some(predicate, thisArg)
Let's see the predicate
and the optional thisArg
in detail below.
2.1predicate
#
Through the predicate
, every
/some
not only gives us access to the current array element but also its index and the original array through its parameters like below:
- 1st parameter: The current array element.
- 2nd parameter: The index of the current element.
- 3rd parameter: The array itself on which
every
/some
is called.
We have only seen the first parameter in action in earlier examples. Let's catch the index and the array by defining two more parameters. This time, let's say we have some T-Shirt data to test if all of them have freeCodeCampe
logo:
let tshirts = [
{ size: "S", color: "black", logo: "freeCodeCamp" },
{ size: "S", color: "white", logo: "freeCodeCamp" },
{ size: "S", color: "teal", logo: "freeCodeCamp" },
{ size: "M", color: "black", logo: "freeCodeCamp" },
{ size: "M", color: "white", logo: "freeCodeCamp" },
{ size: "M", color: "teal", logo: "freeCodeCamp" },
{ size: "L", color: "black", logo: "freeCodeCamp" },
{ size: "L", color: "white", logo: "freeCodeCamp" },
{ size: "L", color: "teal", logo: "freeCodeCamp" },
];
tshirts.every((item, i, arr) => {
console.log(i);
console.log(arr);
return item.logo == "freeCodeCamp";
})
Try this out in your console to see the output. And don't forget to play around with some
too.
2.2Optional thisArg
#
If in any case you need to have a particular this
value inside your predicate, you can set that with thisArg
. Note that is only applicable for non-arrow predicates because arrow functions have no this
bindings.
If you omit this argument, this
inside the predicate (non-arrow function) works as usual, that is:
- In strict mode
this
isundefined
. - In sloppy mode
this
is the global object which iswindow
in browser andglobal
in Node.
I can't think of any good use case of thisArg
. But I think it's good that it exists because now you have control over this
inside your predicate. So even if someday there is a need for it you will know that there is a way.
If you have any good ideas for uses of thisArg
, please let me know on Twitter :)
3Edge cases for every
and some
#
3.1What happens when every
and some
is called on an empty array?#
Sometimes the array you want to test might be empty. For example, you fetched an array from an API and it can have an arbitrary number of elements at different times including zero.
For the case of every
a true
return value can mean two things:
- If the array has more than zero elements, then all elements of the array satisfies the predicate.
- The array has no elements.
So if we want we can do crazy things inside the predicate like below:
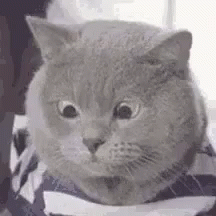
const myCatsBankAccounts = [];
myCatsBankAccounts.every(account => account.balance > elonMusk.totalWealth)
And still get true
as the return value!
If the array is empty, JavaScript directly returns true
without any calls to the predicate.
It's because in logic, you can say anything about the elements of an empty set and that is regarded as true or more precisely vacuously true. Such logic might seem nonsense in everyday usage but it's how logic works. Read the wiki page linked above to know more about it.
So if you get true
as the the return value of every
you should be aware that the array could be empty.
some
on the other hand, directly returns false
on empty arrays without any calls to predicate
and without any weirdness.
3.2Non-existing elements are ignored#
If your array has holes in it like below, they are ignored by every
/some
:
const myUntiddyArry = [1,,,3,,42];
3.3Mutating the array in the predicate#
I will not discuss this case here, because mutating the original array in most cases just complicates things and makes more room for bugs.
If you really need to or are interested, please read the note in the spec for details.
4A challenge for you#
Express every
with some
and some
with every
in JavaScript.
I hope you will also feel the immense joy and wonder that I got when I discovered this relationship!
Solution
Let's do it step by step. First let's try to express every
with some
:
- For every element of the array, the predicate is true.
- It's not true that for some elements of the array the predicate is not true.
We can translate that into JavaScript like below:
const myEvery = (arr, predicate) => !arr.some(e => !predicate(e));
Now let's express some
with every
. It's almost the same as before. Just some
is replaced by every
. Try to understand what is going on:
- For some elements of the array, the predicate is true.
- It's not true that for every element of the array the predicate is not true.
In JavaScript:
const mySome = (arr, predicate) => !arr.every(e => !predicate(e));
Note that the above implementations also work when arr
is empty. And for simplicity, I've excluded other parameters of the predicate
and thisArg
. Try to add these details yourself, if you are interested. In this process, you might learn one or a few things!
Thanks for reading! I hope this article was helpful. Check out my other articles here. Let's connect on Twitter.