When you see the % symbol, you may think "percent". But in Python, as well as most other programming languages, it means something different.
The %
symbol in Python is called the Modulo Operator. It returns the remainder of dividing the left hand operand by right hand operand. It's used to get the remainder of a division problem.
The modulo operator is considered an arithmetic operation, along with +
, -
, /
, *
, **
, //
.
The basic syntax is:
a % b
In the previous example a
is divided by b
, and the remainder is returned. Let's see an example with numbers.
7 % 2
The result of the previous example is one. Two goes into seven three times and there is one left over.
The diagram below shows a visual representation of 7 / 2
and 7 % 2
(The "R" stands for "remainder"). The single logo on the right side (with the green arrow pointing at it) is the remainder from the division problem. It is also the answer to 7 % 2
.
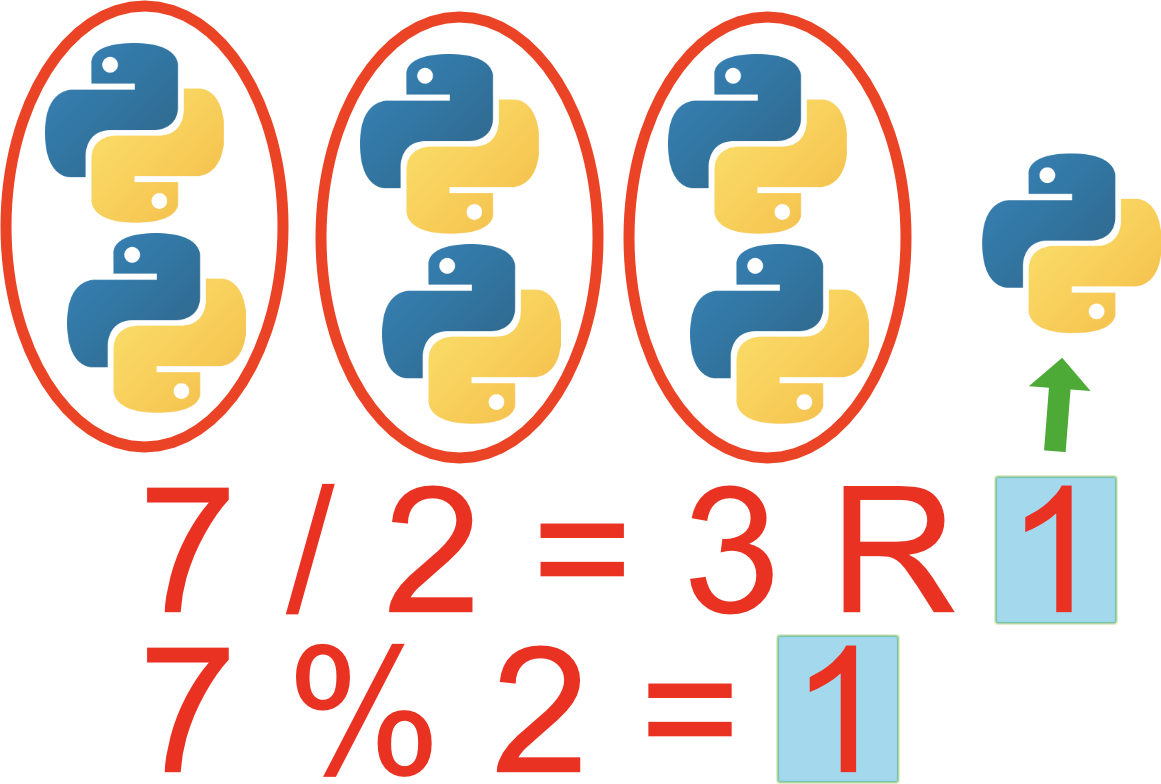
Here is another example:
3 % 4
This will result in three. Four does not go into three any times so the original three is still left over. The diagram below shows what is happening. Remember, the modulo operator returns the remainder after performing division. The remainder is three.
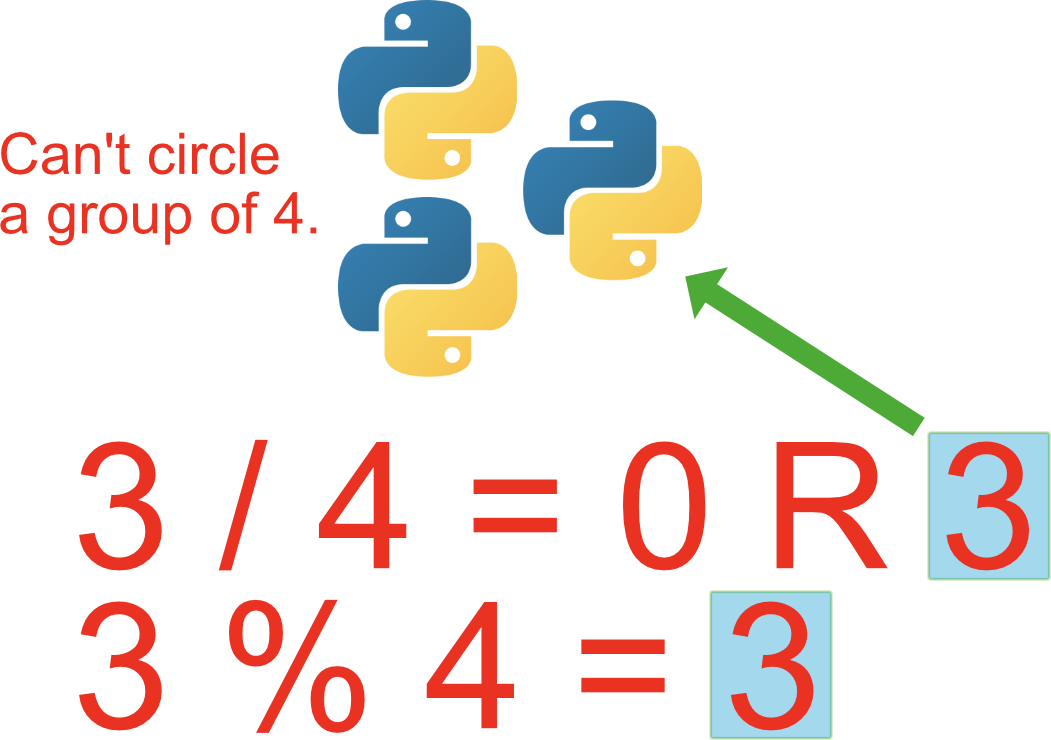
Example using the Modulo Operator
One common use for the Modulo Operator is to find even or odd numbers. The code below uses the modulo operator to print all odd numbers between 0 and 10.
for number in range(1, 10):
if(number % 2 != 0):
print(number)
Result:
1
3
5
7
9