JavaScript is the most widely used scripting language on Earth. And it has the largest library ecosystem of any programming language.
JavaScript is the core language of the web, and the only programming language that can run in all major web browsers.
Notably, JavaScript has no relation to Java. Check out JavaScript: The World’s Most Misunderstood Programming Language.
The official name of JavaScript is ECMAScript defined under Standard ECMA-262.
If you want to learn more about the JavaScript language, and why it’s so widely used, read Quincy Larson’s article - Which programming language should I learn first? - or watch this inspiring video from Preethi Kasireddy.
freeCodeCamp has an in-depth JavaScript tutorial on YouTube that will teach you all the fundamentals in just 3 hours.
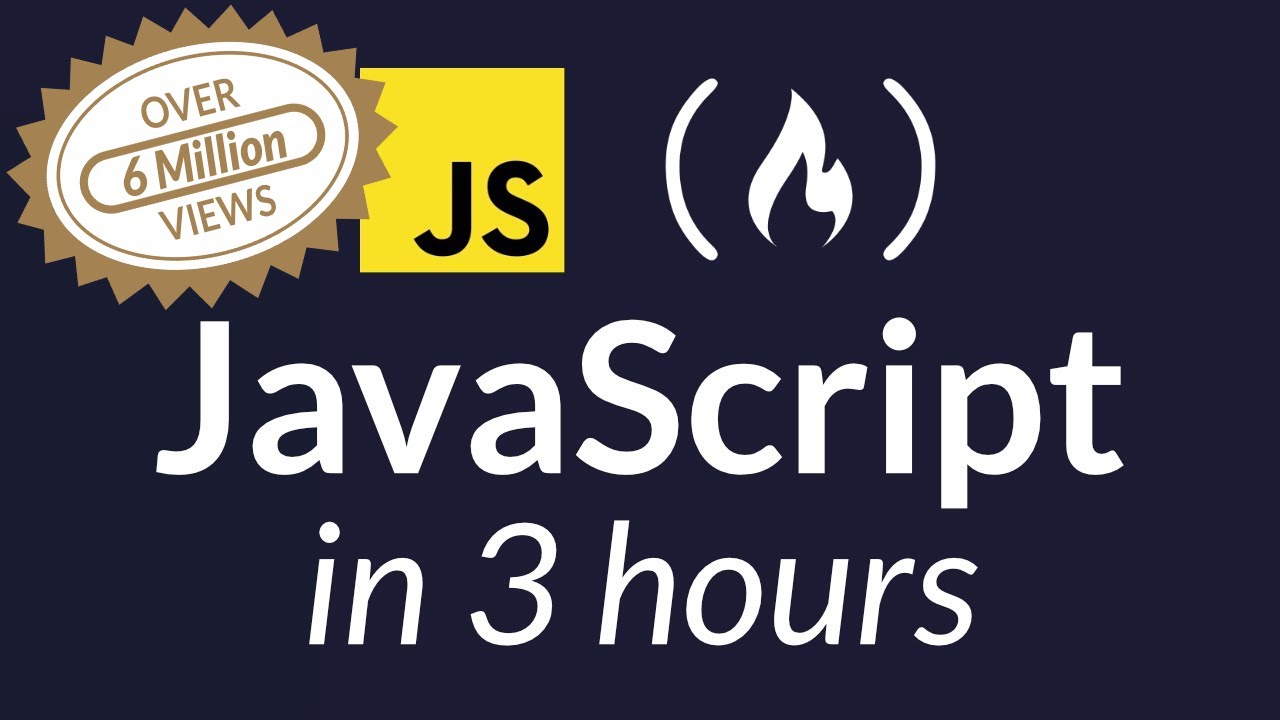
Some other good JavaScript tutorials:
- JavaScript for Cats
- The Modern JavaScript Tutorial
- Professor Frisby’s Mostly Adequate Guide to Functional Programming
- Eloquent Javascript (annotated)
- Speaking Javascript
- Exploring ES6
- Udemy - Javascript Understanding the Weird Parts (first 3.5 hrs)
- Functional programming in JavaScript
- Introduction to JavaScript: First Steps
- Douglas Crockford’s Videos
- Modern JS Cheatsheet
- The 50 Best Websites to Learn JavaScript
- Codementor JavaScript tutorial
- You Might Not Need jQuery
References
Quick JavaScript
- REPL (node)
- JSBin
- JSFiddle
- CodePen
- CoderPad (pair programming)
- C9 (IDE, pair programming)
- Object Playground (visualize objects)
- Plunker
Challenges
Tutorials
Exercises
Editors
Blogs
Podcasts
Video Tutorials
Books
- Secrets of the JavaScript Ninja
- Programming JavaScript Applications
- Maintainable JavaScript
- Learning JavaScript Design Patterns
- Airbnb JavaScript Style Guide
- JSDoc
- Javascript Allonge Six
- You Don’t Know JS
6 books on JavaScript by Kyle Simpson, from beginner to advanced.
Fantastic, thorough introduction to the basics and features of JavaScript, complete with in-browser interactive code.
Quite in-depth guide to Functional Programming in JavaScript
Standalone JavaScript engines
Mozilla’s SpiderMonkey, the first JavaScript engine ever written, currently used in Mozilla Firefox.
V8, Google’s JavaScript engine, used in Google Chrome.
Google Apps Script, a cloud-based/server-side interpreter that provides programmatic “macro-like” control of Google Apps services and documents.
Node.js, built on top of V8, a platform which enables server-side applications to be written in JavaScript.
Windows includes JScript, a JavaScript variant in Windows Script Host.
Chakra, a fork of Jscript, is developed by Microsoft and used in their Edge browser.
Mozilla also offers Rhino, an implementation of JavaScript built in Java, typically embedded into Java applications to provide scripting to end users.
WebKit (except for the Chromium project) implements the JavaScriptCore engine.
JavaScript Frameworks
The most frequently used JavaScript Frameworks are React JS, Angular JS, jQuery, and NodeJS. For more details follow this link.
Advantages and Disadvantages of JavaScript
Like with all programming languages, JavaScript has certain advantages and disadvantages to consider. Many of these are related to the way JavaScript is often executed directly in a client's browser. But there are other ways to use JavaScript now that allow it to have the same benefits of server-side languages.
Advantages of JavaScript
- Speed - JavaScript tends to be very fast because it is often run immediately within the client's browser. So long as it doesn't require outside resources, JavaScript isn't slowed down by calls to a backend server. Also, major browsers all support JIT (just in time) compilation for JavaScript, meaning that there's no need to compile the code before running it.
- Simplicity - JavaScript's syntax was inspired by Java's and is relatively easy to learn compared to other popular languages like C++.
- Popularity - JavaScript is everywhere on the web, and with the advent of Node.js, is increasingly used on the backend. There are countless resources to learn JavaScript. Both StackOverflow and GitHub show an increasing amount of projects that use JavaScript, and the traction it's gained in recent years is only expected to increase.
- Interoperability - Unlike PHP or other scripting languages, JavaScript can be inserted into any web page. JavaScript can be used in many different kinds of applications because of support in other languages like Pearl and PHP.
- Server Load - JavaScript is client-side, so it reduces the demand on servers overall, and simple applications may not need a server at all.
- Rich interfaces - JavaScript can be used to create features like drag and drop and components such as sliders, all of which greatly enhance the user interface and experience of a site.
- Extended Functionality - Developers can extend the functionality of web pages by writing snippets of JavaScript for third party add-ons like Greasemonkey.
- Versatility - There are many ways to use JavaScript through Node.js servers. If you were to bootstrap Node.js with Express, use a document database like MongoDB, and use JavaScript on the frontend for clients, it is possible to develop an entire JavaScript app from front to back using only JavaScript.
- Updates - Since the advent of ECMAScript 5 (the scripting specification that JavaScript relies on), ECMA International has been dedicated to updating JavaScript annually. So far, we have received browser support for ES6 in 2017 and look forward to ES7 being supported in the future.
Disadvantages of JavaScript
- Client-Side Security - Since JavaScript code is executed on the client-side, bugs and oversights can sometimes be exploited for malicious purposes. Because of this, some people choose to disable JavaScript entirely.
- Browser Support - While server-side scripts always produce the same output, different browsers sometimes interpret JavaScript code differently. These days the differences are minimal, and you shouldn't have to worry about it as long as you test your script in all major browsers.
ES6
The 6th edition of ECMAScript is called ES6.
It is also know as ES2015.
The changes add a lot of syntactic sugar that allow developers to create applications in an object oriented style.
ES5 example:
var User = function () {
function User(name) {
this._name = name;
}
User.prototype.getName = function getName(x) {
return 'Mr./Mrs. ' + this._name;
};
return User;
}();
ES6 example:
class User {
constructor(name) {
this._name = name
}
getName() {
return `Mr./Mrs. ${this._name}`
}
}
A lot of new syntax features were introduced including:
- classes
- modules
- templating
- for/of loops
- generator expressions
- arrow functions
- collections
- promises
Nowadays most of the features are available in all popular browsers. The compatibility table contains all information about feature availability of all modern browsers.
Frequently, new features arrive that are part of the successor ES7. A common way is to translate modern JavaScript (ES6, ES7 and other experimental proposals) to ES5. This makes sure that also old browsers can execute the code. There are tools like Babel that transforms new JavaScript to ES5.
Besides syntactic sugar coming from ECMAScript standards there are features that require a Polyfill. Usually they are necessary because whole class/method implementations were added to the standard.
Object Instantiation
In JavaScript and most other languages, an object contains a series of properties, which are a key, value pair. There are multiple options available to you when you need to construct an object.
Initialize an object variable
You can create an object with pre-defined properties like so:
let myObject = {
name: "Dave",
age: 33
}
Creating an empty object
This creates an empty object inside our myObject variable:
let myObject = new Object();
When you wish to add properties to your object, you simply use either dot notation or bracket notation with the property name of your choice:
myObject.name = "Johnny Mnemonic"
myObject["age"] = 55
Using a constructor function
You can define a constructor function that you can use to create your objects:
function Kitten(name, cute, color) {
this.name = name,
this.cute = cute,
this.color = color
}
You can define a variable containing an instantiation of this object by calling the constructor function:
let myKitten = new Kitten("Nibbles", true, "white")
Object.create()
The Object.create() method (first defined in ECMAScript 5.1) allows you to create objects. it allows you to choose the prototype object for your new object without needing to define a constructor function beforehand.
// Our pre-defined object
let kitten = {
name: "Fluff",
cute: true,
color: "gray"
}
// Create a new object using Object.create(). kitten is used as the prototype
let newKitten = Object.create(kitten)
console.log(newKitten.name) // Will output "Fluff"