In this tutorial, we’ll learn how to find the number of vowels in a string with JavaScript. This is a problem you might be asked in junior developer job interviews, and it’s also a CodeWars problem.
Before we get started coding, let’s read over the problem description in full:
Return the number (count) of vowels in a given string. We will consider a, e, i, o and u as vowels, but not y. The input string will only consist of lower case letters and/or spaces.
Step 1: Make a plan to solve the problem
For this problem, we’ll create a function, called getCount
, which takes as input a string and returns as output the count of how many vowels are in that string.
Let’s go over some examples.
With the first example, we see that our function returns 5, which is how many times a vowel appears in the string abracadabra
. With the string abc
, only 1 is returned, as only one vowel (a) appears.
To solve this problem, we’ll create a vowelsCount
variable that will keep track of how many vowels are in the string.
We’ll also create an array, vowels, that holds all of our vowels. We’ll go through each character in our string. If the character is a vowel, we’ll increase our vowelsCount
variable.
Finally, we’ll return the vowelsCount
variable.
Let’s get started!
Step 2: Write the code to solve the problem
First we write our function, getCount
. Next we’ll create a variable, vowelsCount
, and set it to 0
.
We’ll create our vowels array next. This allows us to have every vowel in one place, and we can use this array later.
Now we need to go through every character in our input string, str
. We need to go through or look at every character in our string so that we can determine whether or not it is a vowel.
To do this, we can use the for...of
statement that works on strings. You can read more on it here.
Now inside our for loop, we have the ability to look at and go over each character in our string.
Next, we want to check whether or not each character is a vowel.
To do this, we can use the includes
method. The includes()
method determines whether an array includes a certain value among its entries. It returns true if so, and false if not.
Using includes
, we’ll check if our vowels array contains the character we’re currently iterating over in our loop.
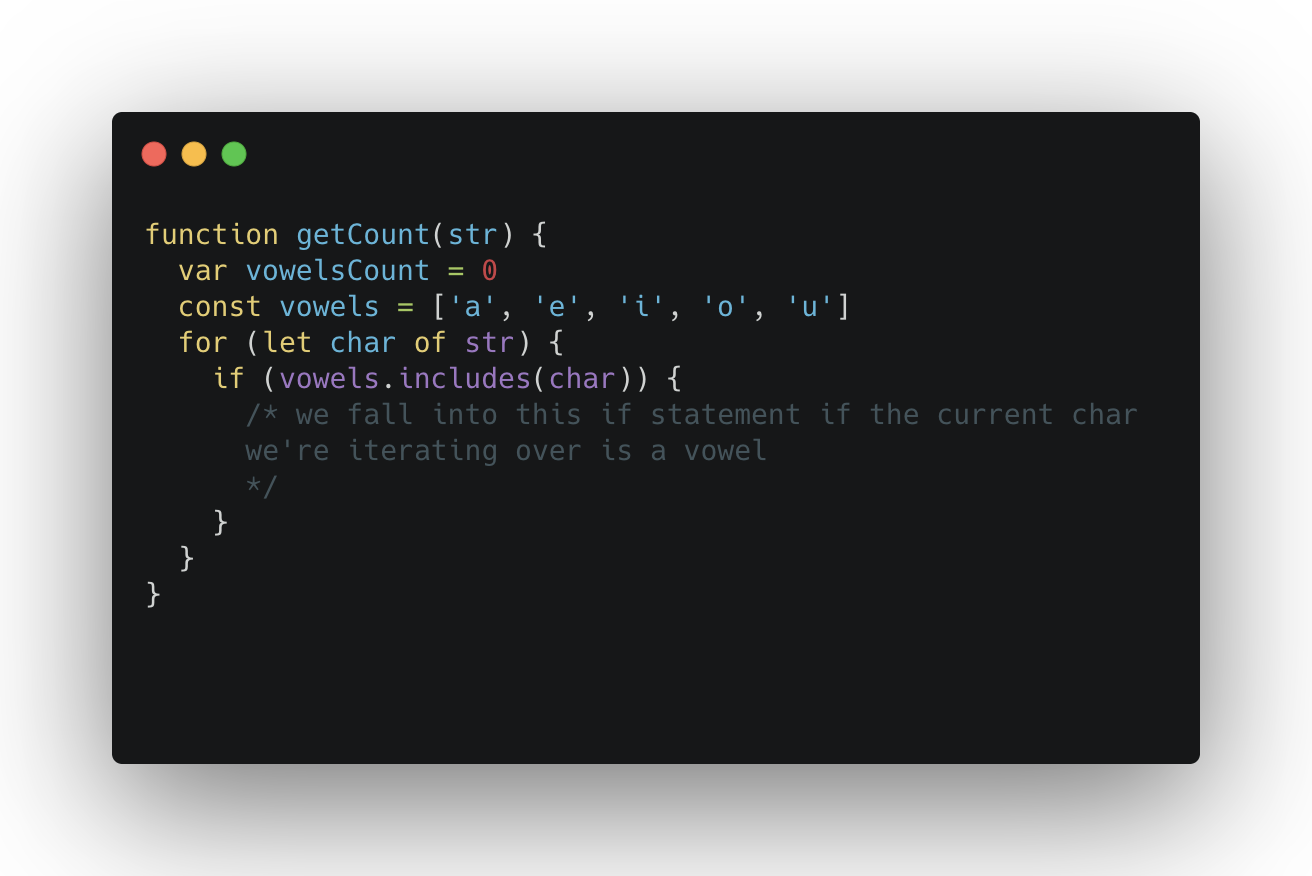
We’ve created our if statement
to check whether the current character is a vowel. If the character is a vowel, then we want to increase our vowelsCount
variable. To do this, we can use the increment operator in JavaScript:
At this point in our code, we’ve looked at each character in the string, determined whether it was a vowel or not, and increased the number we stored in vowelsCount
if it was.
Lastly, all we need to do is have our function return our vowelsCount
variable. We can do this by returning the variable outside of our loop.
There we have it.
That's it!
We’ve now written a function that will take as input a string and return as output the number of times a vowel appeared in the string.