JavaScript
is a widely used programming language that has ruled the software development technology stack for over a decade.
You can find JavaScript in web applications, mobile applications, or apps for your desktop.
According to a survey done by Statista
in 2022, JavaScript developer communities worldwide have about 17.4 million members, and they're growing.
There are also a number of JavaScript frameworks and libraries, like React, Angular, and Vue, that are solving varied use cases and customer problems. And there are many resources available online and in textbooks to learn JavaScript.
Despite the language being so useful and well-known, many JavaScript developers still need help to learn the language confidently. They might be able to complete their day-to-day development tasks using JavaScript with some help, but may need clarification on the underlying concepts. And they may struggle to debug issues and solve customer problems on time.
This article doesn't aim to teach you JavaScript at all. It would be wonderful if you could actually learn JavaScript from such a short article, right.
Instead, this article aims to teach you How to learn JavaScript
to help you become a confident JavaScript developer. I'll talk about strategies and concepts that are essential to understand before you learn JavaScript to make your learning more effective.
If you like to learn from video content as well, this article is also available as a video tutorial here: 🙂
Let's Go Back to Kindergarten
In your early years, when you were learning a spoken or written language (like English, Spanish, Hindi, and others), did you start forming sentences without knowing about the words? Did your teacher try to make you spell words without teaching you the alphabet? Probably not!
The same goes for any programming language as well. You must know how to learn it progressively. How do things work under the hood? Why do certain concepts exist? What do you not need to focus on? In a nutshell, you need to understand the fundamental concepts
.
Just as you need to understand basic grammatical and spelling concepts to be able to correctly form sentences in your own language, programming languages also need "something" to run correctly. The rules and grammar of a programming language are called syntax
.
By the way, fun fact: if you think the word Kindergarten
in the heading is a misspelling, you are mistaken, like I was:
Confession:
— Tapas Adhikary (@tapasadhikary) February 13, 2023
I didn't know that "Kindergarden" is not a correct spelling. It's "Kindergarten". 😲
Google it if you find it surprising too.
I'm pointing this out to emphasize that syntax
is as important as concepts
!
With all this in mind, let's focus on learning JavaScript.
How to Use JavaScript Topic Bubbles
Undoubtedly, JavaScript is vast! As a programming language, it covers all essential aspects of software development:
- Scope
- Functional Programming
- Data Types
- Data Structures
- Operations
- Expressions
- Memory Management
- Reflection
- OOP
- Web APIs
- Asynchronous Programming
- Exception Handling
- Events
- Immutability
- DOM
- Many much more
The list will grow if we continue, but our objective is different. You just need to realize that the number of topics you may have to learn to master the language is quite large. But I do not intend to scare you!
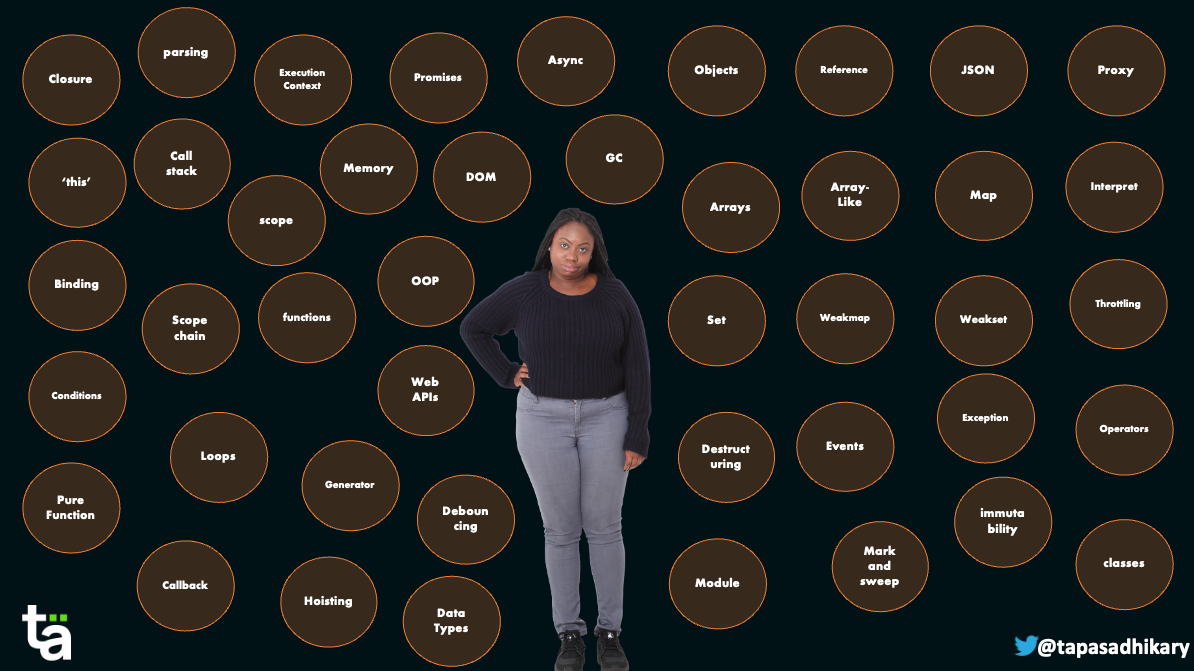
If you're a beginner JavaScript developer, you might be wondering:
- Can I do this?
- How many courses and tutorials will I need to go through?
- Isn't it too much?
- How do I remember everything that I am learning?
- Overall, how can I actually learn JavaScript?
Let's find the answers together as we read further.
Introducing a JavaScript Learning Model
Let me introduce a conceptual model for learning JavaScript. The model consists of strategies and concepts you must embrace to learn JavaScript effectively.

The model is summarized in these six points, which I will expand on soon.
✅ First, you need to learn Programming Concepts
.
✅ Concepts >>>
Syntax.
✅ Create a mind map
of related topics.
✅ Practice incrementally
.
✅ You don't have to worry about most JavaScript weird things
.
✅ Build projects
that connect the concepts.
Programming Concepts vs JavaScript in Particular
To begin with, separate the basic programming concepts from JavaScript specifically. These basic programming concepts are common among most programming languages.
Learning them well will help you to pick up any programming languages easily. Some fundamental programming concepts are:
- Variables: In programming, variables are the human-readable names you provide to a memory location that stores a value. For example,
car
,students
, andoranges
are variable names. - Values: A value is something that we usually manipulate, change, or use to create other values. We assign values to variables. For example,
Honda
can be the value of thecar
variable. - Data Types: Data types indicate the particular type of a value like number, string, boolean, and others.
- Operators: Operators in programming are symbols that help us perform operations like addition, subtraction, multiplication, concatenation, and so on.
- Conditions and Control Flow: Conditions in programming are statements that result in some actions. It's like if this happens, then do that, otherwise do some other thing.
- Loops: Loops in programming are about repeating a bunch of tasks in the same sequence under a specific condition. There are different loops available in programming languages, and some common ones are a for-loop, while-loop, and do-while-loop.
- Functions: Functions are a block of code you want to execute over and over again rather than repeating it everywhere. You create a function by giving it a name and a bunch of instructions to execute when you invoke it.
- Environment: The programming environment allows developers to code, test, build, and deploy. The base principle remains the same for each aspect of the environment, but the technology may differ for different programming languages.
- Debugging: Debugging is the art of finding and fixing problems in your code. You must know how to debug code irrespective of the language in which the code has been written.
All the points mentioned above are basic programming concepts. As a JavaScript developer, you must be familiar with these concepts.
Concepts >>> Syntax
We discussed the differences between programming concepts and programming syntax. They both are important. But, understanding concepts helps us better than memorizing syntax.
❌ Memorizing Syntaxes.
— Tapas Adhikary (@tapasadhikary) January 30, 2023
✅ Understanding Concepts.
Let's take an example problem statement that we might solve using coding.
The problem statement: If the colour variable has the value "red", return a red ball. Otherwise, return a green ball.
The JavaScript code to address this problem statement is pretty straightforward:
if (color === "red") {
// return a red ball
} else if(color === "green") {
// return a green ball
}
But if you memorize the above syntax and do not try to understand the concepts behind it, you may have problems in the future. If someone tweaks the problem statement a bit, your memorized code syntax may not work to produce the expected results.
In this problem statement, the programming concept used is flow control
. So if you draw the flow control as a diagram and associate it with your code syntax, you will imprint the logic on your brain more permanently. Here's what it would look like:
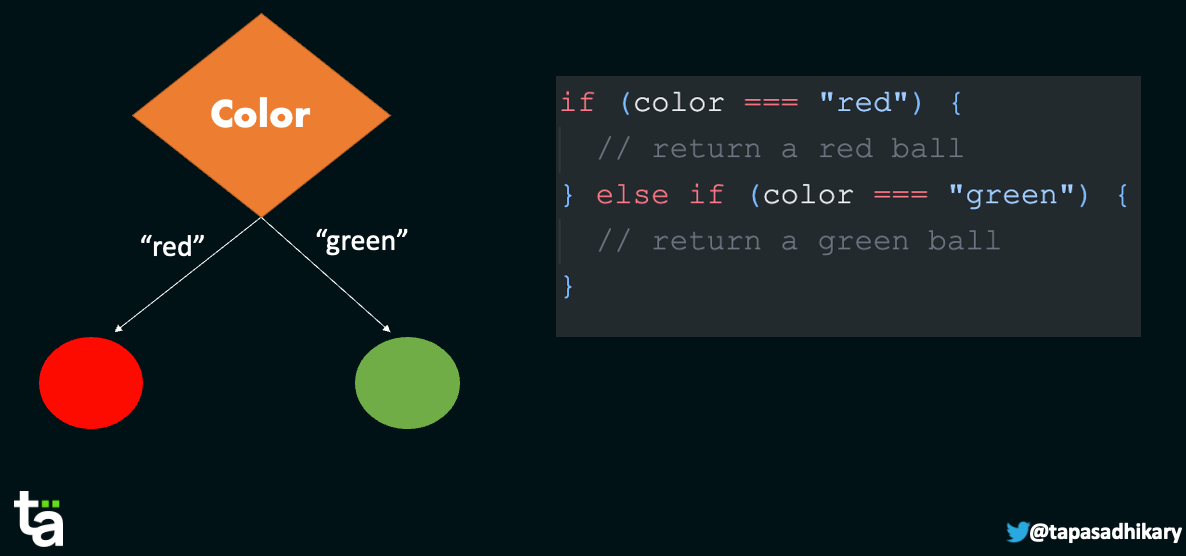
If the problem statement changes, you change the flow, re-draw it, and map it back to your code.
The JavaScript Topics Mind Map
When you pick up a JavaScript topic to learn, you start by going over any resources that you find helpful. It's an excellent first step, but more is needed for effective learning
.
The effective way is to create a mind map of JavaScript topics and guide your learning. It will help you to know the concept well and familiarise yourself with related concepts. Another advantage of mind maps is with multiple topics, you form an intersection of common sub-topics to go over multiple times.
Here is an example of a mind map to learn the JavaScript this
keyword. As you can see, the flow of topics moves logically through progressive concepts to help you get a foundational understanding of the this
keyword in JavaScript.

Another example could be understanding JavaScript promises. It would help if you started with functions, then the call stack and execution context, then learned about task and job queues and finally promises.
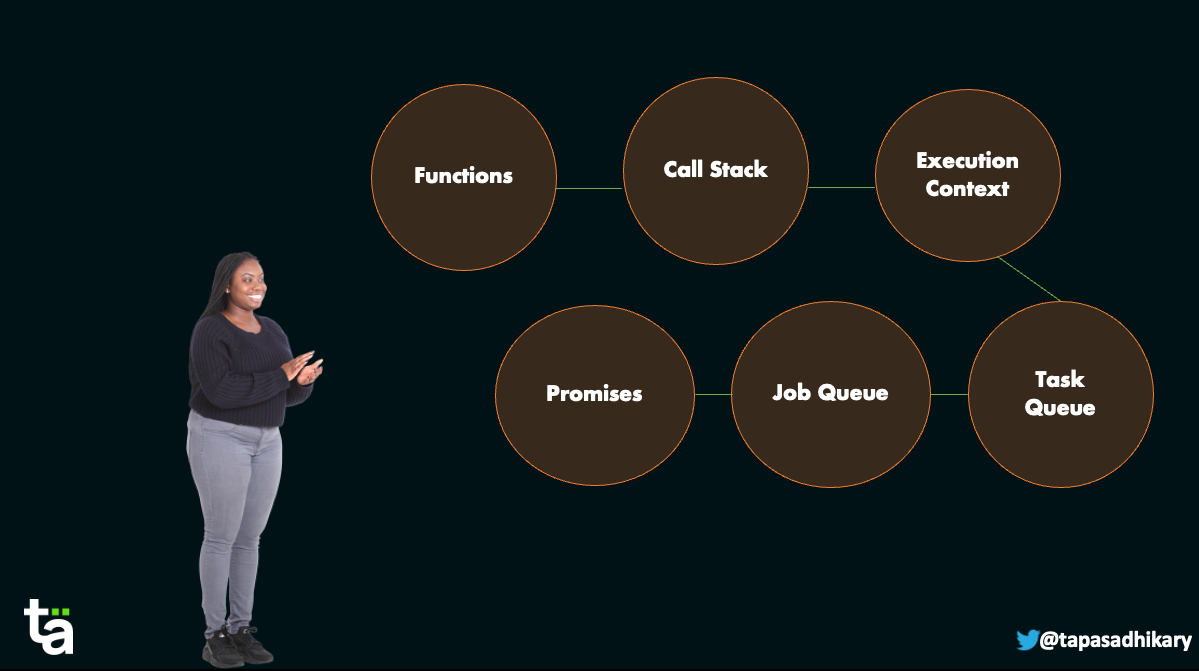
It may sound like a time-consuming process, but following the mind map approach to learning fundamentals will save you lots of time in the long run.
The great thing about understanding fundamentals is you save lots of time in the long run.
— Tapas Adhikary (@tapasadhikary) February 11, 2023
Practice Incrementally
Practice makes you a better programmer. As you start understanding JavaScript concepts, you need to practice them. Practice incrementally to make your learning engaging and continuous. What does incremental practice mean, though?
In an incremental practice strategy, you start with a practice code example of a concept. When you go to the next concept in the mind map, you build your next practice code example on top of the previous example.
Let's say you are learning about JavaScript functions and practising by creating code snippets. Next, when you learn about scope or the scope chain, extend the same example you used for functions and inject the scope and scope chain concepts into it.
Say, you are learning to write a function that adds two numbers. You may come up with a sum()
function like this:
var sum = function(a,b) {
return a + b;
}
By creating this function, you would have learned:
- How to create a function
- How to pass parameters to a function
- How to return from a function
- How to use function as an expressions
Next, a logical step will be to call/invoke the function.
sum(2,3); // produces 5 as result
Great! Now when you learn about scope, you can use the same function to practice functional scope.
Take a look into the example below. Here the calc()
function uses the same sum()
we learned a while back.
function calc() {
var extra = 5;
var sum = function(a,b) {
return a + b;
}
let total = extra + sum(2,3);
}
console.log(extra); // is extra accessible here?
calc();
console.log(total); // is total accessible here?
In the calc()
function we are trying to experiment with the scope of a couple of variables. In the same way, now you can write an example of a scope chain by using the same calc()
function (and I am leaving that to your creativity to try out).
This strategy has a couple of advantages:
- As your concepts are connected in a mind map, your examples are also connected topic by topic.
- In the future, when you refresh your memory by looking back at any of the examples, it will be easier for you to traverse back and forth to connect the dots.
Connect Concepts to Projects
While you practice the concepts incrementally, it is essential to create projects. When you follow a course or tutorial, you get to practice the exact project your instructor will teach. This is good, but can be better.
After you follow the instructor and complete the project, try to pause. Think, what else can you build with the concept you learned just now? You are on the right track if you can think of an idea or use case to build using the concept. Next, try implementing it.
You may get stuck in the implementation phase. Go back to your topic mind maps. Find which concepts you need to brush up on to remove the blockers. Read up on those concepts, practice them incrementally, and start back on the project. That's how you learn effectively!
Here are some ideas of projects to build to get you started as you're learning:
Is JavaScript Weird?
The rumors are that JavaScript is weird
! Well, it can be, especially when you see code like these examples:
+[] == +![]; // returns true
Number([]); // returns 0
null >= 0; // returns true
0.1 + 0.2 == 0.3 // Who could believe, it returns fasle!
[10, 1, 3].sort() // Returns, [1, 10, 3]
How can you explain these? The only way you can explain these quirks is by explaining their underlying concepts. Besides a few tiny weird things (for example, the type of null
is still an object
in JavaScript), you can explain most syntax by understanding the underlying concepts. You'll need to try doing that to figure out these weird things.
So, What's Next?
I hope this article provides insights and a less daunting path forward for learning JavaScript effectively
. I've been working on JavaScript and frameworks/libraries based on it for over a decade. Everything I shared here is from my experience in learning the language deeply.
Try following the six points
you have learned about the JavaScript learning model
. Feel free to tweak them based on your needs. If you have any further queries, feel free to connect.
Let's connect.
- SUBSCRIBE to my YouTube channel if you want to learn JavaScript, ReactJS, Node.js, Git, and all about Web Development in a practical way.
- Follow on Twitter and LinkedIn if you don't want to miss the daily dose of Web Development and Programming Tips.
- Follow on Showwcase for community-based learning.
- You can connect with me on a call.
See you soon with my next article. Until then, please take care of yourself, and stay happy.