Running HTTPS in development is helpful when you need to consume an API that is also serving requests via HTTPS.
In this article, we will be setting up HTTPS in development for our create-react-app with our own SSL certificate.
This guide is for macOS users and requires that you have brew
installed.
Adding HTTPS
In your package.json
, update the start script to include https:
"scripts": {
"start": "HTTPS=true react-scripts start",
"build": "react-scripts build",
"test": "react-scripts test",
"eject": "react-scripts eject"
},
Running yarn start
after this step will show you this screen in your browser:
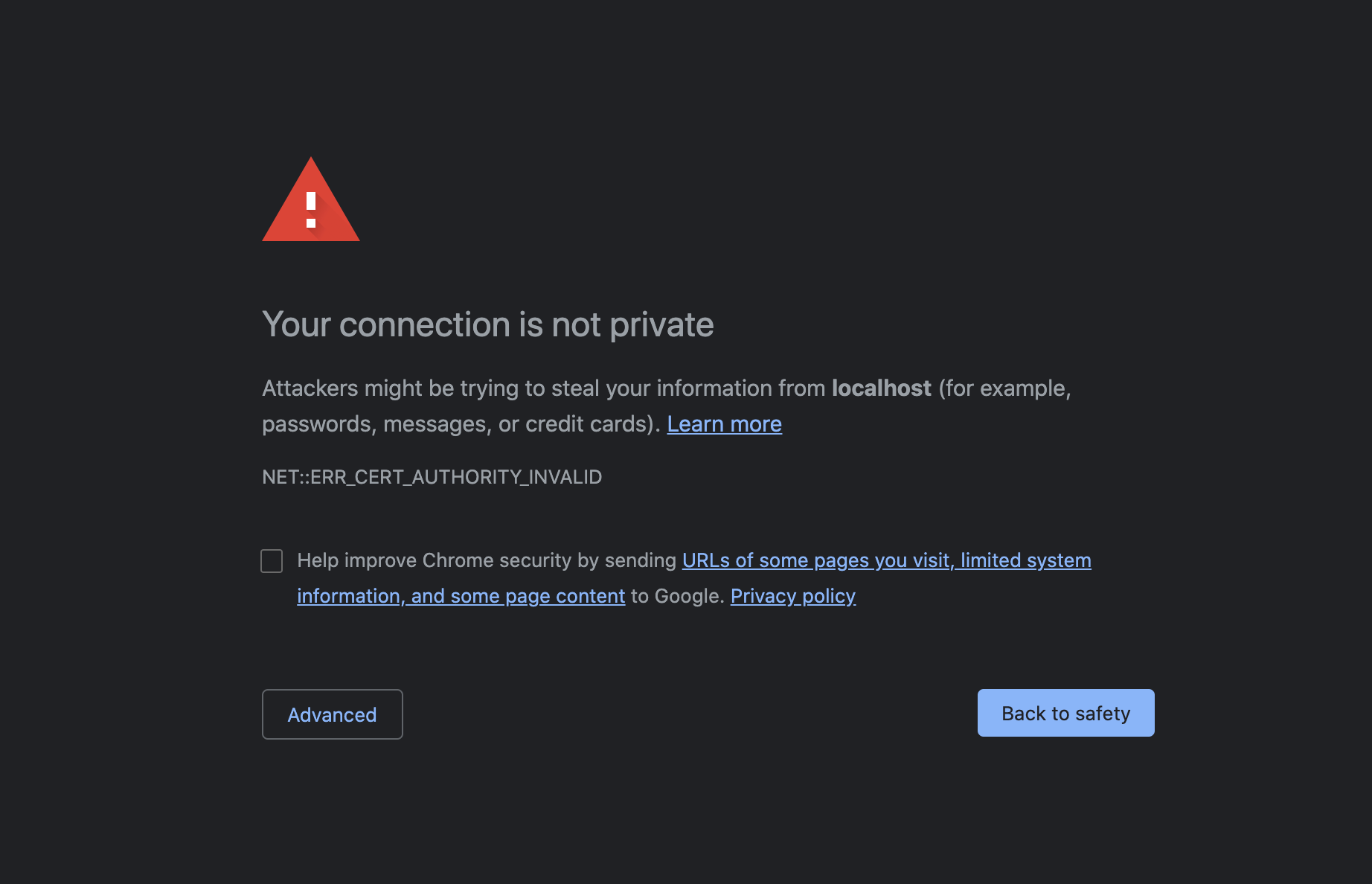
At this stage, you're already set to go with https
. But you don't have a valid certificate, so your connection is assumed to be insecure.
Creating a SSL Certificate
The easiest way to obtain a certificate is via mkcert
.
# Install mkcert tool
brew install mkcert
# Install nss (only needed if you use Firefox)
brew install nss
# Setup mkcert on your machine (creates a CA)
mkcert -install
After running the above commands, you'll have created a certificate authority on your machine which enables you to generate certificates for all of your future projects.
From the root of your create-react-app
project, you should now run:
# Create .cert directory if it doesn't exist
mkdir -p .cert
# Generate the certificate (ran from the root of this project)
mkcert -key-file ./.cert/key.pem -cert-file ./.cert/cert.pem "localhost"
We'll be storing our generated certificates in the .cert
directory. These should not be committed to version control, so you should update your .gitignore
to include the .cert
directory.
Next, we need to update the start
script again to include our newly created certificate:
"scripts": {
"start": "HTTPS=true SSL_CRT_FILE=./.cert/cert.pem SSL_KEY_FILE=./.cert/key.pem react-scripts start",
"build": "react-scripts build",
"test": "react-scripts test",
"eject": "react-scripts eject"
},
When you run yarn start
again, you should now see that your connection is secure.
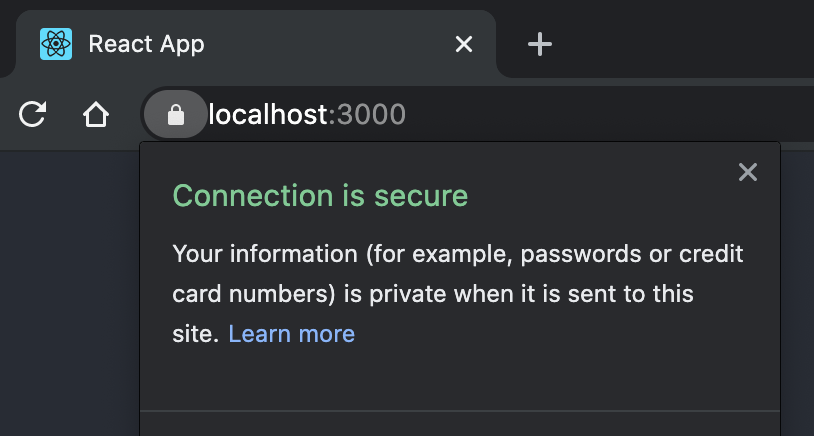
Don't be a stranger! Feel free to write if you have any questions - connect with me on Linkedin or follow me on Twitter.