Building projects is one of the most effective ways to thoroughly learn a concept and develop essential skills.
Projects immerse you in real-world problem-solving, solidifying your knowledge and cultivating critical thinking, adaptability, and project management expertise.
This guide will walk you through building a movie recommendation system that's tailored to user preferences. We'll leverage a vast 10,000-movie dataset as our foundation.
While the approach is intentionally simple, it establishes the core building blocks common to the most sophisticated recommendation engines in the industry.
Your Toolkit: Python, pandas, and scikit-learn
We'll harness the power and versatility of Python to manipulate and analyze our data.
The pandas library will streamline data preparation, and scikit-learn will provide robust machine learning algorithms like CountVectorizer and cosine similarity.
User experience is key, so we'll design an intuitive web application for effortless movie selection and recommendation display.
What You'll Achieve:
- Develop a data-driven mindset: Understand the essential steps in building a recommendation system.
- Master core techniques: Dive into data manipulation, feature engineering, and machine learning for recommendations.
- Create a user-centric solution: Deliver a seamless experience for personalized movie suggestions.
Let's get started!
Table of Contents
- What Are Our Goals?
- Importance of Machine Learning in Movie Recommendations
- Data Collection and Preprocessing
- Feature Selection and Engineering
- CountVectorizer for Text Pre-Processing
- Machine Learning for Recommendation
- Framework Selection
- Data Splitting: Training, Testing, and Validation
- Walkthrough of the Python Code
- Full Jupyter Notebook Code
- Insights and Outcomes
- Challenges and Solutions in Machine Learning-based Recommendations
- Limitations and Areas for Future Improvement

What Are Our Goals?
Imagine a movie recommendation system that understands your unique taste, consistently suggesting films you'll genuinely enjoy. With the right strategy, you can turn this vision into reality.
Master Data Preparation
Here, you'll learn how to unlock the power of your movie data. Using Python's pandas library, you'll focus on key details like movie ID, title, overview, and genre.
By strategically combining 'overview' and 'genre' into 'tags,' you'll create a powerful foundation for personalized recommendations.
Harness Machine Learning for Tailored Suggestions
Machine learning is your secret weapon. You'll learn how to use the CountVectorizer to transform movie descriptions into data your system can analyze. Then, you'll leverage cosine similarity to pinpoint movies that align with your preferences.
These techniques enable your recommender system to learn and adapt with each movie you watch.
Build a Dynamic Recommendation Function
You'll build a function that expertly analyzes movie indices and similarity scores, ensuring your recommendations accurately reflect your evolving tastes.
This function is where the magic happens, transforming your past choices into a custom-curated list of must-watch movies.
Ensure Consistency Through Serialization
To maintain the accuracy of your recommendations over time, you'll embrace serialization using the pickle library. Think of this as your system's memory, preserving its insightful personalization features for future sessions.
Craft an Intuitive User Interface
A well-designed interface is vital. Streamlit empowers you to build a user-friendly experience with elements like dropdown lists that further refine recommendations based on your input.
In this guide, you'll learn to prioritize intuitive navigation and design to ensure a seamless, enjoyable interaction with your system.
Approach Overview
This chapter briefly explains the core steps of building a movie recommendation engine using Python. You'll create a system that delivers personalized suggestions and fosters an intuitive, engaging user experience.
Step 1: Data Preparation
The foundation of any successful recommendation system lies in well-prepared data.
You'll start by importing your movie dataset (consider Kaggle for a robust source) using Python's pandas library. You'll focus on understanding the structure of your data, addressing missing values, and ensuring it's ready for analysis.
Step 2: Feature Engineering for Insight
Isolate the most impactful features – movie ID, title, overview, and genre are strong contenders.
The key to unlocking personalization is a 'tags' column. You'll learn how to merge 'overview' and 'genre' to capture both textual descriptions and categories. This unified approach enhances your system's ability to zero in on movies your users will enjoy.
Step 3: Choose Your Algorithm Wisely
CountVectorizer from scikit-learn is a powerful tool for transforming your 'tags' into usable numerical data. You'll learn how to apply cosine similarity to calculate how closely movies align based on these features.
Experts often favor cosine similarity for its ability to accurately measure relationships between items, especially when multiple dimensions are involved.
Step 4: Build Your Recommendation Engine
You'll design a function that intelligently analyzes movie indices and similarity scores.
This is the heart of your system, where you'll generate tailored recommendations that reflect user preferences. You'll want to prioritize a straightforward approach for initial success – you can always introduce complexity later.
Step 5: Enhance the Experience
Serialization (using Python's pickle module) ensures your system 'remembers' past choices for consistent recommendations.
You'll use Streamlit to build a user-friendly interface with minimal coding effort. Features like dropdown lists promote seamless interaction and further personalize results.
Step 6: Go the Extra Mile
Then you'll integrate APIs like TMDb to dynamically display movie posters, adding a rich visual element to your recommendations. Consider features like image carousels for a dynamic and immersive interface.
This foundational system can act as a launchpad for exploring more advanced techniques and features to continuously optimize your recommendations.
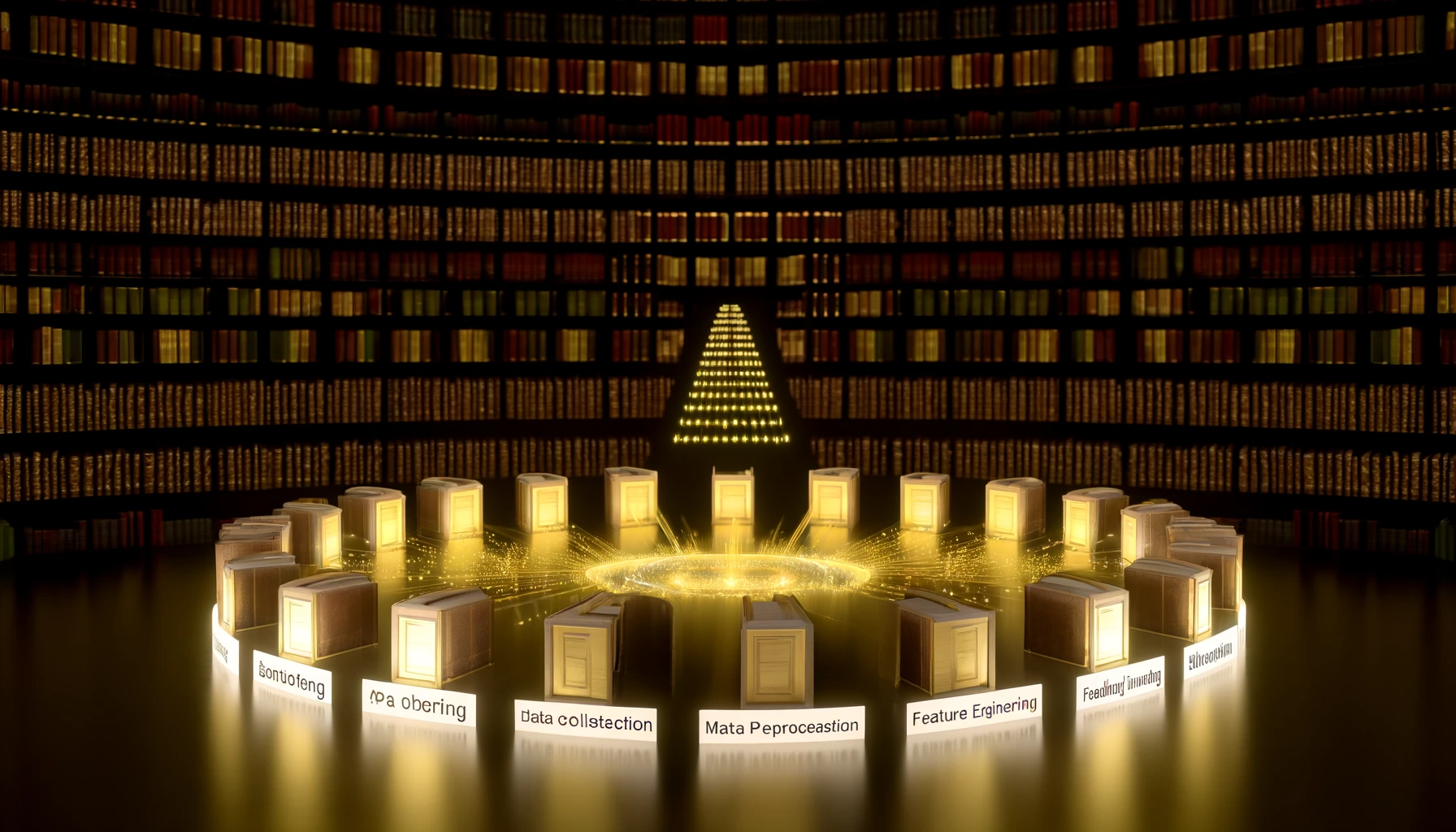
Importance of Machine Learning in Movie Recommendations
Let's delve into how machine learning revolutionizes movie recommendations, delivering personalized experiences that keep users engaged.
Supervised Learning: Predicting User Preferences
Supervised learning is the foundation for predicting what movies a user will enjoy. It analyzes historical data like past ratings and viewing history to uncover patterns.
For example, if a user loves sci-fi films, a supervised model trained on this data will intelligently recommend other sci-fi movies.
Unsupervised Learning: Finding Hidden Connections
Unsupervised learning excels at finding similarities between movies based on features like genre, director, or plot – even when direct user feedback is limited. It might cluster movies into groups like "action," "romantic comedy," or "horror," offering a powerful tool for recommendations.
Reinforcement Learning: Continuously Improving
Reinforcement learning takes personalization to the next level. The system makes a recommendation, analyzes the user's response (rating, watch/no-watch), and constantly adapts. This dynamic approach ensures recommendations stay relevant to changing user preferences.
How to Choose Your Approach
- Supervised Learning: Best when you have abundant user ratings/viewing history.
- Unsupervised Learning Uncovers patterns in movie data, even with limited feedback.
- Reinforcement Learning: Ideal for real-time adaptation and maximizing user satisfaction long-term.
The Best Systems Use All Three
It's often the best idea to combine these techniques strategically! Supervised learning predicts ratings, unsupervised learning finds hidden movie connections, and reinforcement learning adapts to real-time interactions.
This blend maximizes recommendation accuracy and user satisfaction.
Data is the Key to Success
Diverse, high-quality data is crucial for success:
- Movie Basics: Titles, genres.
- User Insights: Demographics, viewing history, social media activity.
- Data Transformation: Normalization ensures unbiased treatment by your model. TF-IDF converts text data (descriptions, reviews) into usable numbers.
Preprocessing is Essential
Clean data is paramount. Handle missing values, remove duplicates, and filter out anything that might skew results. This might feel secondary, but it has a significant impact on recommendation accuracy.
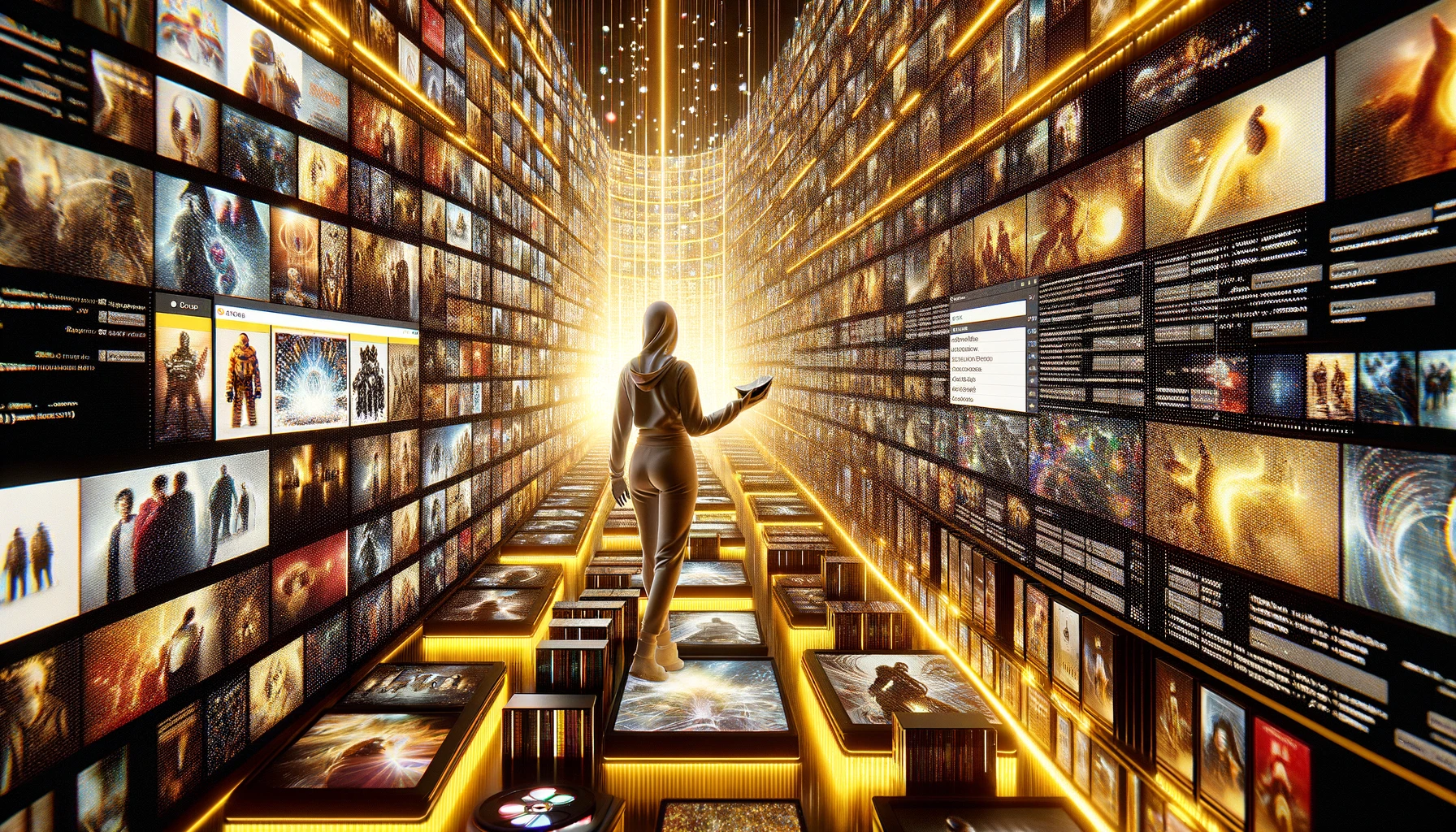
Data Collection and Preprocessing
Alright, now you're almost ready to build a system that understands your movie preferences. I'll outline the key steps here, empowering you to analyze movie data and deliver recommendations that hit the mark.
Your Development Toolkit
- Python Power: Harness pandas for data manipulation and scikit-learn for machine learning magic. We will
- Coding Environments: Choose your weapon – Jupyter Notebook for interactive exploration, VS Code for development, or Google Colab for cloud-based collaboration.
Data Collection
- Find Your Dataset: Start with Kaggle's datasets or explore alternatives like TMDb. Aim for a rich dataset with movie titles, descriptions, genres, and ideally, user ratings.
- Load it Up:
pd.read_csv()
brings your dataset into a pandas DataFrame, ready for analysis.
Understand Your Data
- First Look:
movies.head(10)
reveals your data's structure. - Statistics:
movies.describe()
uncovers summary statistics. - Missing Values:
movies.isnull().sum()
flags data gaps – clean-up is crucial!
Feature Engineering
- Focus Your Engine: Target essential features – ID, title, overview, and genre.
- The 'Tags' Advantage: Combine 'overview' and 'genre' for a richer representation of each movie.
- Streamline: Drop redundant columns to maximize efficiency.
Prepare Your Text Data: Machines 'Read' Differently
- CountVectorizer: Your text-to-numbers transformer. Experiment with parameters for optimal results.
- Transform 'Tags': Convert your rich descriptions into a format your model can understand.
Measure Similarity: The Key to Relevant Recommendations
- Cosine Similarity: The industry standard for comparing text-based features. Dig deeper to understand how it works!
- The Similarity Matrix: This output is your recommendation engine's heart.
Build Your Recommendation Function
- Logic is Key: Design a function that analyzes movie indices, similarity scores, and delivers targeted suggestions.
- Test Drive: Try
recommend("Iron Man")
– did you get results that make sense?
Save Your Progress
- Pickle Power: Serialize your processed data and similarity model for quick restarts and future improvements.
Feature Selection and Engineering
Feature selection and engineering are where the magic happens in movie recommendation systems. By crafting the perfect set of data points, you empower your model to deliver recommendations that feel tailor-made for each user. Let's unlock the full potential of this process:
Harness the Power of Text Data with TF-IDF (and Beyond!)
- Text as Treasure: Movie descriptions, reviews, and social media chatter hold immense value if you know how to extract it. TF-IDF is a powerful starting point, but it's not the only tool in your arsenal.
- Sentiment Analysis: Tools that analyze the tone or emotional content of text can reveal another layer of preferences ("dark and suspenseful" vs. "lighthearted and fun").
- Topic Modeling: Identify underlying themes and recurring concepts in descriptions or reviews. This is especially useful for finding movies that share a similar vibe, even if the genres are different.
Manage Feature Correlation: Efficiency and Hidden Insights
- Correlation as a Roadmap: Analyze how features relate to each other. Strong correlations suggest redundancy, while weak correlations might point to untapped areas of user preference.
- Data Doesn't Lie: Don't make assumptions! Let your data analysis reveal unexpected connections between features. Maybe users who enjoy historical dramas also have a surprising affinity for quirky sci-fi comedies.
- Streamline and Optimize: Use your correlation insights to eliminate unnecessary features, boosting your model's efficiency and avoiding overfitting.
Feature Engineering: Real-World Examples (Expanded)
- Go Beyond Genres: Break down broad categories into granular subgenres or niche interests for unparalleled personalization.
- User Feedback Goldmine: Don't just track ratings. Analyze completion rates, watch time, search patterns, and even comments for subtle preference indicators.
- Embrace the Unexpected: Create features based on director, cast, cinematographer, or even soundtrack style. These can cater to users with specific tastes.
- Tagging is Your Friend: Let users generate their own tags or use natural language processing to extract key themes from movie descriptions for highly tailored recommendations.
Level Up: Advanced Strategies
- Embrace the Dynamic: Consider time of day, location, current mood, or recent watch history for real-time personalization.
- Metadata Matters: Movie release year, awards, or even critical reception can add valuable predictive power, especially when combined with other features.
- Experiment and Learn: Start with a solid foundation, then test different feature combinations. Monitor your model's accuracy metrics to guide your refinement process. Don't be afraid to try something outside the box!
Expert Tip: Remember, even with advanced techniques, some of the most powerful features can be surprisingly simple. A user's most-watched director or favorite actor might be all you need for a spot-on suggestion.
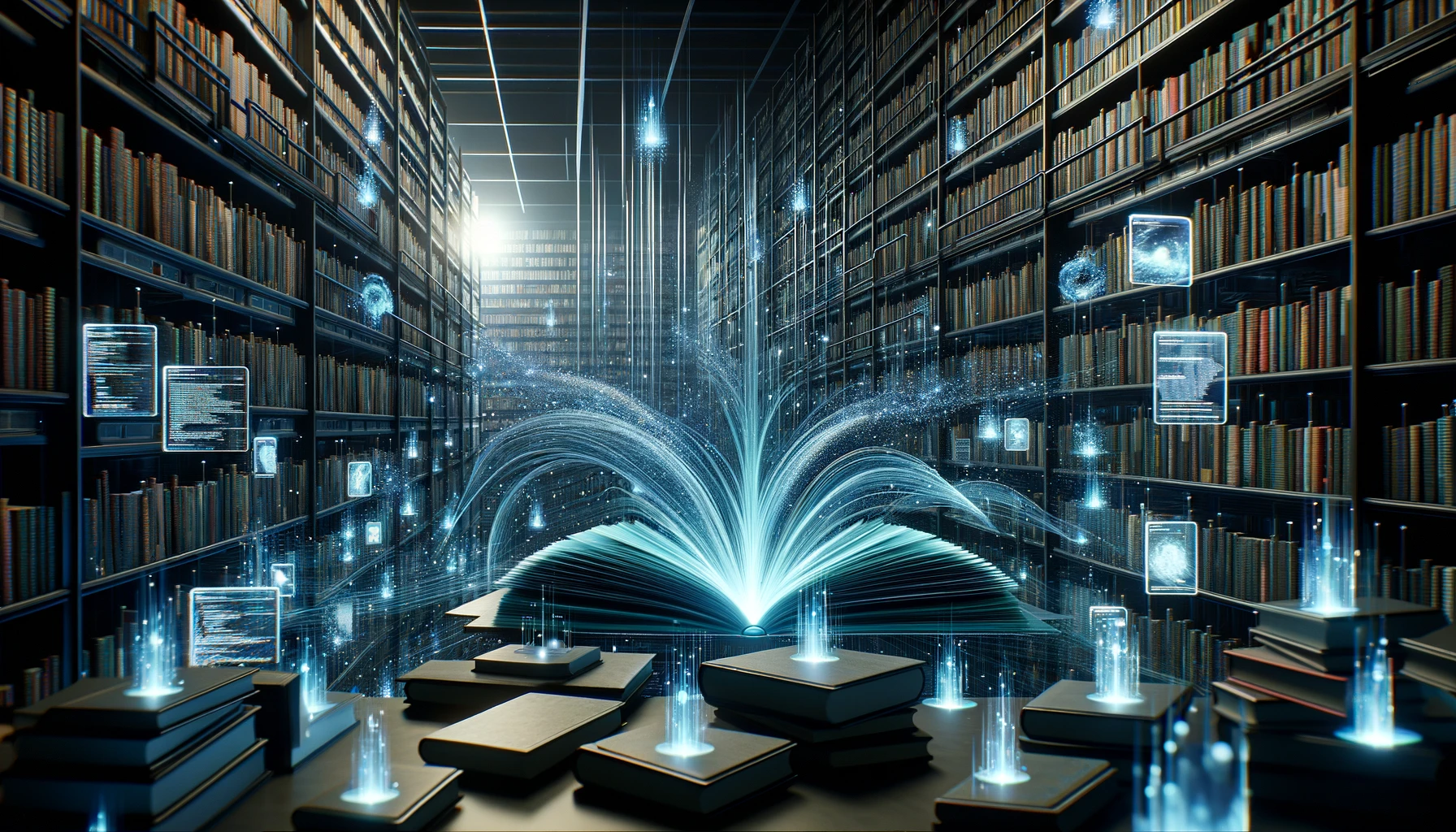
CountVectorizer for Text Pre-Processing
Text vectorization is a crucial process in machine learning as it converts text data into a numerical format that algorithms can process effectively. This transformation is especially significant when dealing with large volumes of text data.
CountVectorizer, a widely-used tool in natural language processing (NLP), plays a key role in text data processing. It converts text data into a structured form, specifically a sparse matrix of token counts, which is highly suitable for machine learning models.
In our movie recommendation system, CountVectorizer is essential in quantifying movie descriptions. By quantifying the descriptions, the system can accurately compare and recommend movies based on textual similarities.
To implement CountVectorizer in Python, you can use the following example code:
# Import CountVectorizer again (redundant import)
from sklearn.feature_extraction.text import CountVectorizer
# Initialize a CountVectorizer object with a maximum of 10,000 features and English stop words
cv = CountVectorizer(max_features=10000, stop_words='english')
# Fit the CountVectorizer to the 'tags_clean' column and transform the text data into a numerical vector representation
vector = cv.fit_transform(new_data['tags_clean'].values.astype('U')).toarray()
Remember to customize CountVectorizer for movie data by adjusting its parameters to capture unique aspects of movie descriptions, such as genre-specific terms.
It is important to strike a balance between accuracy and efficiency in vectorization. Finding the right level of depth in vectorization while maintaining computational efficiency is crucial for a responsive and accurate recommendation system.
Also, for large movie datasets, implementing practices like dimensionality reduction and regular updates to the word dictionary can optimize the performance of CountVectorizer.
Basics of Text Data in Recommendation Systems
Text data in movies, including descriptions and genres, is inherently unstructured and diverse, presenting a challenge in standardizing the data for recommendation algorithms.
But analyzing text data accurately plays a crucial role in enhancing movie recommendations. By deeply understanding the content of movies through text data analysis, the recommendation system can provide more precise and personalized recommendations.
When dealing with unstructured text in movie descriptions, it is essential to address the nuances of natural language, such as idioms, context, and sentiment. These elements are critical in comprehending movie content and ensuring accurate recommendations.
To address these challenges, you'll need to do some text preprocessing to clean and prepare the data. This involves removing irrelevant characters, correcting typos, and standardizing text formats for consistency.
Tokenization, the process of breaking down text into individual words or tokens, makes analysis and quantification easier. Also, removing common stop words and applying stemming, which reduces words to their root form, helps focus on the most meaningful elements of the text.
Text Preprocessing for Vectorization
Text data preprocessing is an essential step in preparing data for analysis in the field of Natural Language Processing (NLP). It involves cleaning and standardizing the data to ensure consistency. This includes removing irrelevant characters, correcting typos, and standardizing text formats.
For example, you can use string manipulation functions in Python to remove special characters and regular expressions to correct common typos.
Tokenization is another crucial step in text data preprocessing. It involves breaking down the text into individual words or tokens, making it easier to analyze and quantify.
In Python, you can use various libraries such as NLTK or spaCy to tokenize text data. For example, NLTK provides a word_tokenize()
function that splits text into words.
Removing stop words and applying stemming are also important steps in text preprocessing.
Stop words are commonly used words that do not carry much meaning, such as "the," "and," or "is." Removing these words helps to focus on the most meaningful elements of the text.
Stemming reduces words to their root form, which can help in capturing the essence of the word. Libraries like NLTK provide built-in stop word lists and stemming algorithms, such as the Porter stemming algorithm.
To implement these preprocessing steps in Python, you can use various libraries and functions. For example, NLTK provides functions for removing stop words and applying stemming. Here is an example code snippet:
# Importing necessary modules from NLTK for text preprocessing.
import nltk
import re
from nltk.corpus import stopwords
from nltk.stem import WordNetLemmatizer
from nltk.tokenize import word_tokenize
# Downloading NLTK resources necessary for text processing.
nltk.download('punkt')
nltk.download('wordnet')
nltk.download('stopwords')
Introduction to CountVectorizer
CountVectorizer is a powerful tool in the field of Natural Language Processing (NLP) that converts text data into a matrix of token counts. It provides a simple yet effective way to analyze text by counting the frequency of each word in the text. This approach is particularly useful for basic NLP tasks and is widely used in machine learning and data science.
To understand how CountVectorizer works, let's dive into the mathematics behind it. The algorithm begins by creating a dictionary of all unique words present in the text. It then counts the occurrences of each word in each document, resulting in a matrix representation of the text data. This matrix captures the frequency of each word, allowing for further analysis and comparison.
When compared to other text vectorization techniques like TF-IDF, CountVectorizer focuses solely on word frequencies, making it simpler and more straightforward. This simplicity is advantageous for basic NLP tasks and provides a solid foundation for further analysis.
To implement CountVectorizer in Python, you can follow these steps:
# Importing CountVectorizer from scikit-learn to convert text documents to a matrix of token counts.
from sklearn.feature_extraction.text import CountVectorizer
# Installing scikit-learn if not already installed.
pip install scikit-learn
# Initializing CountVectorizer with a limit on the maximum number of features and excluding common English stop words.
cv = CountVectorizer(max_features=10000, stop_words='english')
# Fitting the CountVectorizer to the cleaned tags and transforming the text data into a numerical array.
vector = cv.fit_transform(new_data['tags_clean'].values.astype('U')).toarray()
In this code snippet, we import the CountVectorizer class from the sklearn library. We then create an instance of CountVectorizer and fit it to the text data. Finally, we transform the text data into a word frequency matrix using the transform()
method.
To optimize CountVectorizer for your specific needs, it's important to customize its parameters. For example, you can adjust the max_features
parameter to limit the number of features in the matrix, or use the ngram_range
parameter to consider phrases of multiple words. You can also provide a custom list of stop_words
to exclude commonly used words that may not carry much meaning.
It's worth noting that while CountVectorizer is a powerful tool, striking a balance between accuracy and efficiency is crucial. For large datasets, implementing practices like dimensionality reduction and regular updates to the word dictionary can optimize performance.
How to Implement CountVectorizer in Python
Implementing CountVectorizer in Python is a straightforward process. First, import the CountVectorizer class from the sklearn library. Then, create an instance of CountVectorizer, like cv = CountVectorizer()
. Next, fit the CountVectorizer to your text data using the fit_transform()
method, which converts the data into a word frequency matrix.
Here's an example code snippet:
# Initializing CountVectorizer with a limit on the maximum number of features and excluding common English stop words.
cv = CountVectorizer(max_features=10000, stop_words='english')
# Fitting the CountVectorizer to the cleaned tags and transforming the text data into a numerical array.
vector = cv.fit_transform(new_data['tags_clean'].values.astype('U')).toarray()
When customizing CountVectorizer for movie data, consider adjusting parameters to capture unique aspects of movie descriptions.
For example, you can set the max_features
parameter to limit the number of features in the matrix or use the ngram_range
parameter to analyze phrases of multiple words. Also, providing a custom list of stop_words
can exclude commonly used words that may not carry much meaning.
Finding the right balance between accuracy and efficiency is crucial in vectorization. For large movie datasets, consider implementing practices like dimensionality reduction and regular updates to the word dictionary. These practices optimize CountVectorizer's performance and ensure a responsive recommendation system.
CountVectorizer in the Movie Recommendation System
CountVectorizer plays a crucial role in converting movie descriptions into a numerically analyzable format.
For example, if we have a movie description like "An epic adventure in space," CountVectorizer would first tokenize this sentence into individual words ["An", "epic", "adventure", "in", "space"]. Then, it removes common stop words (in this case, "An" and "in") which do not contribute significantly to the overall meaning. Finally, it counts the occurrence of the remaining meaningful words.
This process turns a piece of text into a numerical vector, making it accessible for mathematical algorithms.
The next step involves utilizing the vectorized data to create a similarity matrix. This matrix is critical for identifying which movies are similar to one another.
For instance, if our dataset contains the vectorized descriptions of movies A and B, the cosine similarity metric is used to compute the similarity score between them. The score quantifies how similar the contents of the two movies are, based on their description vectors.
The structured approach of CountVectorizer allows for an objective and precise comparison of movie content.
For instance, if a user enjoys "Iron Man," the system can find other movies with similar themes by comparing their vectorized descriptions. This leads to more accurate and personalized recommendations.
Suppose "Iron Man" is vectorized as [0, 1, 2] and another movie "The Avengers" is vectorized as [1, 1, 1], the similarity score calculated will help in determining how close "The Avengers" is to "Iron Man" in terms of content.
These examples illustrate the practical application of CountVectorizer and cosine similarity in enhancing the functionality of a movie recommendation system. They show the transition from raw text to vectorized formats and how these vectors are used to find similar movies, providing a comprehensive understanding of the underlying processes.
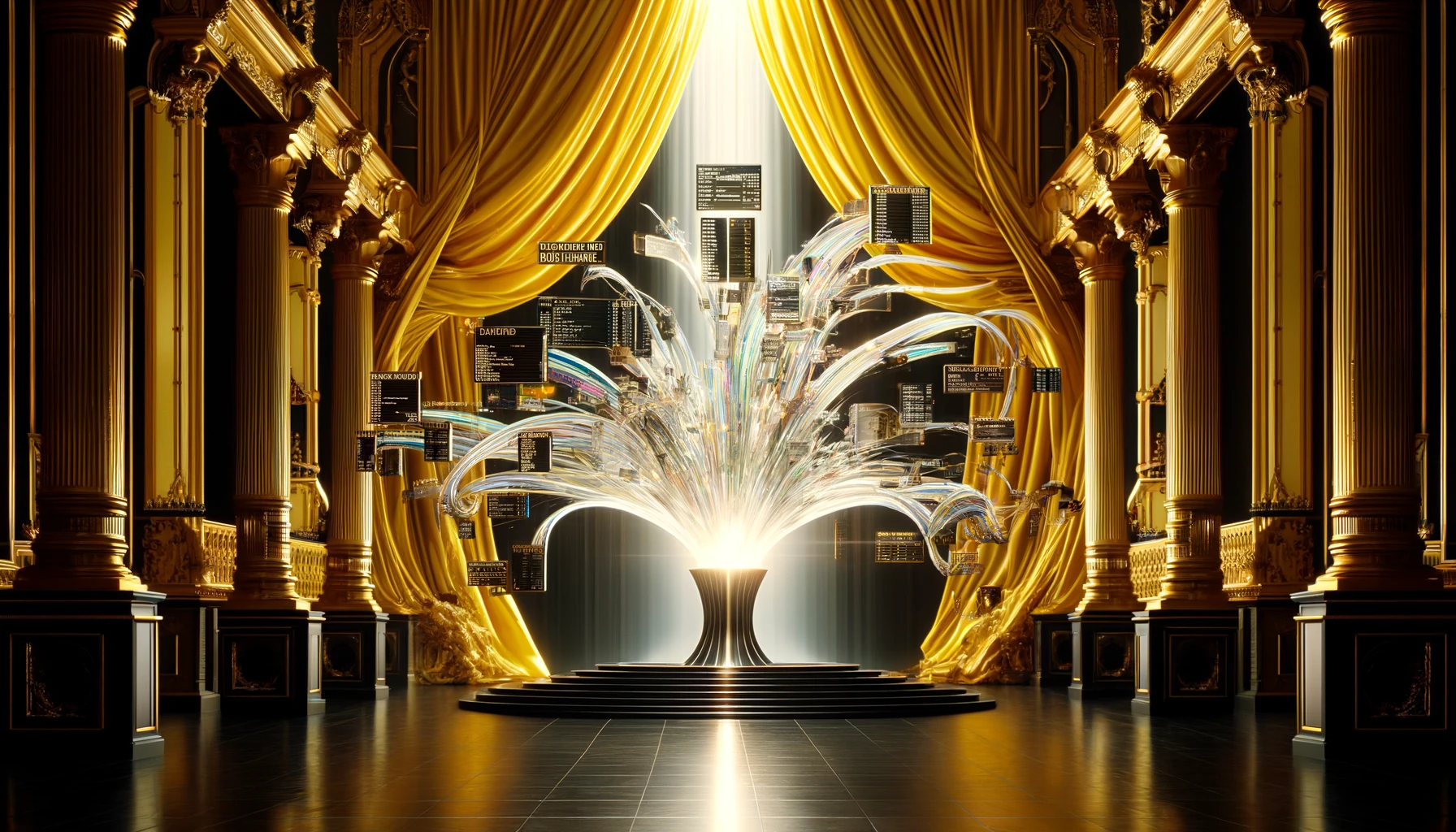
Machine Learning for Recommendation
Understanding the strengths and nuances of each approach is key to designing a recommendation system that truly caters to your users.
Content-Based Filtering: The Power of Targeted Recommendations
- "Made for You" Recommendations: This method excels at matching a user's known preferences with movies that possess similar core attributes. Analyzing genre, cast, director, plot themes, and even stylistic touches from movie descriptions lets you find highly targeted recommendations.
- New Discoveries, Your Way: Uncover hidden gems. If you loved a recent film's visual storytelling or offbeat humor, content-based algorithms can surface lesser-known films that share those qualities. This delights even the most discerning cinephiles.
Potential Pitfalls: Over-reliance can lead to predictable recommendations. Mitigate this proactively with these techniques:
- Diverse Recommendations: Intentionally recommend movies from genres the user hasn't explored recently.
- Explorative Features: Allow users to control the balance between relevance and novelty. Maybe they want familiar favorites one day and unexpected discoveries the next.
- Explicit Feedback: Let users flag recommendations as "spot-on" or "not my style" to refine the system's understanding of their unique tastes.
Collaborative Filtering: Recommendations Inspired by Your Community
- Leveraging Collective Wisdom: Taps into the aggregated ratings and viewing habits of users with similar tastes. This strategy unlocks the power of serendipitous discovery – it's like having a community of expert movie-watchers guiding you.
- Dynamic and Adaptable: Recommendations naturally evolve alongside your users' preferences. This adaptability keeps them engaged, always feeling like the system 'gets them.'
Overcoming Challenges:
- "Cold Starts": For new movies or users without history, content-based filtering offers a starting point. You can also incentivize new users to provide basic preferences upon signup.
- Scalability: Big datasets demand specialized algorithms. Explore options like matrix factorization for efficient handling of sparse data.
Hybrid Systems: The Best of Both Worlds
- Winning Combo: Seamlessly blend these techniques for maximum personalization and the potential for delightful surprises. This approach puts user experience front and center.
- Strategic Implementation: Start with a robust content-based system for immediate value, even with limited user data. Add collaborative filtering as your user base and computational resources grow.
- Fine-Tune for Success: Monitor these success metrics:
- Accuracy: Do recommendations consistently hit the mark?
- Diversity: Does the system avoid pigeonholing users?
- User Satisfaction: Direct feedback (surveys, interaction data) reveals what's truly working.
Taking it to the Next Level: Advanced Considerations
- Beyond Just Ratings: Incorporate implicit feedback like:
- Watch time: Did they finish the film? Rewatch parts?
- Navigation Patterns: What they search for, hover over, or add to watchlists.
- Engagement: Reviews, shares – especially helpful for new content without ratings.
- Context-Aware Recommendations: Make your system hyper-relevant:
1. Time Matters: A lighthearted comedy might be perfect for weeknights, but a gripping drama is better for weekend viewing.
2. Mood Matchmaking: Suggest something upbeat if the user seems bored, or offer a comforting classic if they seem down.
3. Location-Based: Highlight new releases playing in their local theater.
Data Excellence: The Foundation for Accurate Recommendations
- Dataset Selection: Prioritize reputable sources like TMDb and Kaggle for comprehensive movie data. To achieve deeper personalization, explore additional datasets incorporating user interactions (ratings, reviews), social signals, or external critical reception.
- Data Integrity: Dedicate significant effort to cleaning and preprocessing. Use pandas for data manipulation and validation. Address missing values, outliers, and inconsistencies strategically to avoid model bias and ensure recommendation accuracy.
- Feature Engineering: Consider incorporating these features to enrich your recommendations:
1. Release Date: Target recent releases, classics, or specific eras.
2. Cast, Crew, Production: Appeal to niche interests and stylistic preferences.
3. Awards and Nominations: May signal higher production value or critical acclaim.
Algorithm Selection & Optimization for Enhanced Performance
- Align Strategy with Goals: Content-based filtering is ideal for initial personalization, but consider hybrid approaches as your dataset and computational resources grow. Collaborative filtering can unlock serendipity and scalability.
- Iterative Refinement: Explore diverse text analysis techniques (topic modeling, sentiment analysis) to uncover hidden preferences. Continuously experiment, track key metrics, and optimize algorithm configurations to improve recommendation quality over time.
- Metrics That Matter: Focus on both precision (how relevant are recommendations) and recall (is the system offering diverse suggestions?). For large datasets, consider Normalized Discounted Cumulative Gain (NDCG) to measure your model's ability to rank the most relevant options at the top.
- Scaling Success: Proactively research matrix factorization and graph-based algorithms for efficient performance with vast datasets and complex user-movie relationships.
Prioritize User Experience for Engagement and Loyalty
- Balancing Power with Usability: While Streamlit is effective for prototyping, consider Flask or Django for full-fledged web applications. These frameworks offer flexibility for features like user accounts, advanced filtering, and persistent watchlists.
- Visual Appeal: Invest in visually engaging design. Incorporate movie posters, trailers (via API integrations), and intuitive carousels to drive user interaction and discovery.
- Feedback Loops: Implement robust rating systems and qualitative feedback mechanisms. Analyze this data to pinpoint areas for improvement, understand user decision-making, and continually refine your recommendation process.
Expert Guidance: Strategies for Future Refinement
- Real-Time Adaptation: Explore reinforcement learning to dynamically adjust recommendations based on immediate user feedback (clicks, watch time, and so on).
- Context-Aware Recommendations: Factor in user location, inferred mood, or social context (solo vs. group watching) for even more tailored suggestions.
- Responsible AI: Proactively address ethical considerations in recommendation systems. Research fairness metrics, bias mitigation techniques, and transparent communication with users about data usage.
Evolving Systems
Machine learning models that continuously adapt are ideal for this dynamic domain. Consider these approaches:
- Reinforcement Learning: Systems that learn by trial and error, adjusting based on immediate user reaction.
- Time-Sensitive Models: Recent preferences often outweigh older ones in the movie world.
Expert Tip: Continual evaluation is your superpower! A/B test different approaches, monitor metrics, and solicit qualitative user feedback to build a truly exceptional system.

Framework Selection
The right algorithm is the heart of a recommendation system that truly understands your users. Let's explore the nuances of each approach, when to combine them, and advanced techniques to take your system to the next level.
Content-Based Filtering: The Power of "Just Like This"
- Targeted Recommendations: This method shines when you need to match a user's known favorites with movies that share similar core attributes – genre, cast, director, visual style, even specific plot elements or themes.
- Beyond the Obvious: Use sophisticated text analysis to uncover unexpected connections. Maybe a user consistently enjoys dark comedies; look for quirky dramas that share the same offbeat humor and complex characters.
- Code in Action: Python's CountVectorizer transforms movie descriptions into numerical data, while cosine similarity quantifies how closely movies align. Experiment with TF-IDF weighting or explore word embeddings for even richer representations.
Ideal Scenarios:
- New users without extensive viewing history.
- Finding niche films outside the mainstream.
- When explainable recommendations are valuable (you can show why a movie was suggested).
Collaborative Filtering: Recommendations Inspired by Your Community
- Harnessing Collective Wisdom: Analyzes patterns across users with similar tastes, allowing you to recommend what others like them have enjoyed. It's like having a network of expert movie-watchers providing personalized suggestions.
- Dynamic and Adaptable: This approach naturally evolves with user preferences, keeping recommendations fresh and relevant.
Overcoming Challenges:
- The Cold Start: Content-based filtering bridges the gap for new users or movies. You could also incentivize users to provide initial preferences during signup.
- Scalability: For massive datasets, specialized techniques like matrix factorization or graph-based algorithms optimize performance.
- Code Example: Python's K-nearest neighbors (KNN) identifies 'neighbors' with similar tastes, while libraries like Surprise offer additional collaborative filtering algorithms.
Hybrid Systems: Unlocking the Full Potential
- Strategic Fusion: Seamlessly blend these techniques to maximize personalization and the potential for delightful surprises. This approach puts user experience front and center.
- Iterative Refinement: Start with a robust content-based system for immediate value, even with limited user data. Gradually layer in collaborative filtering as your dataset and computational resources grow.
- Metrics that Matter: Monitor how recommendations match explicit user tastes (accuracy) while also introducing welcome surprises (serendipity and diversity).
Advanced Considerations: Refine, Adapt, Excel
Go Beyond Explicit Ratings: Track implicit feedback:
- Watch time and completion rates
- Search patterns and hovered-over titles
- Engagement (reviews, shares, watchlist adds) - especially valuable for content without extensive ratings.
Context is King: Tailor recommendations based on:
- Time of day/week (lighthearted vs. immersive)
- Location (emphasize local new releases)
- Inferred Mood (upbeat suggestions vs. comforting classics)
Embrace Change: Utilize reinforcement learning for systems that improve through trial-and-error or implement time-aware models that weight recent preferences more heavily.
Bias Awareness: Critically assess your dataset for potential biases (popularity, gender representation, etc.) Proactively implement strategies to mitigate them for a truly inclusive recommender.
Expert Tip: Continual experimentation is key! A/B test different approaches, track diverse metrics, and actively seek user feedback to build an exceptional system that fosters trust and loyalty.

Data Splitting: Training, Testing, and Validation
Data splitting is your secret weapon for ensuring that your recommendations are accurate and adaptable to the ever-changing world of movie preferences.
Let's dive into the nuances of this process and strategies for taking your system to the next level.
Step 1 - Setup: Harness Python's Power
- Load Your Data:
import pandas as pd
andmovies = pd.read_csv('movies.csv')
. - Dive into Your Dataset: Commands like
movies.head(10)
,movies.info()
, andmovies.describe()
offer a comprehensive understanding of your dataset. Pay close attention to missing values, outliers, potential inconsistencies, and the overall balance of classes (genres, release periods, etc.). These insights will guide later decisions. - Data Transformation: Ensure numerical data for compatibility with machine-learning algorithms. Text fields (descriptions, reviews) may necessitate techniques like CountVectorizer or TF-IDF to convert them into usable formats.
Step 2 - Split and Conquer: The Heart of Reliability
- Avoid Overfitting: A model that learns the training data too well won't be useful when faced with new users or movies. Splitting forces your model to learn general patterns that translate to the real world.
- The Gold Standard: A meticulously held-out test set, unseen during training, is your most reliable benchmark of recommendation accuracy in realistic scenarios.
- Iterate and Improve: The validation set is your playground. Experiment with algorithms, analyze the impact of different features, and fine-tune hyperparameters for the best performance. Re-evaluate on the validation set each time to ensure improvements translate to unseen data.
Step 3: Code in Action
from sklearn.model_selection import train_test_split
is your go-to tool. Consider these factors when deciding on percentages:
- Dataset Size: With massive datasets, smaller test sets might suffice.
- Complexity: Intricate models may benefit from larger validation sets for optimal tuning.
Step 4: Advanced Strategies for Complex Problems
- Stratified Sampling: Maintain the balance of your original dataset within each split. This is vital if you're aiming to make accurate recommendations for even underrepresented categories (niche genres, classic films, etc.).
- K-Fold Cross-Validation: Ideal for limited datasets. This ensures every data point gets used for both training and validation across multiple iterations, providing a more robust evaluation of your model's potential.
- Time-Series Splits: Crucial if analyzing viewing trends across time (users likely watch different types of movies on weekends vs. weekdays). This helps avoid accidentally "predicting" the past based on future data, which wouldn't serve your users. .
- Evaluation Metrics: Accuracy Alone Isn't Enough Consider precision, recall, F1-scores, or even business-specific metrics (did a recommendation lead to a completed viewing? repeat customers?). These offer a holistic assessment of your system.
Step 5: Preserve, Iterate, and Scale
- Saving Your Model's Progress: Serialization (
import pickle
) allows you to reload the model later for deployment, adding new data, or incorporating feedback without starting from scratch. - The Future is Change: Don't treat your system as static. Re-evaluating splits periodically keeps your recommendations fresh. This is especially true as your user base grows and movie trends shift.
Expert Tip: Data splitting decisions should align with your system goals. Aiming for highly-personalized top-5 recommendations demands a different approach than predicting whether a user will broadly like or dislike a movie. Let this guide your strategy!

Walkthrough of the Python Code
Creating a movie recommendation system is a fascinating project that combines data manipulation, machine learning, and user interface design.
In this section, I'll guide you through this process step by step, based on everything you've learned so far. We'll start from the very basics of handling data with Python.
For this project, we'll use the pandas library for data manipulation and analysis. This library is essential for dealing with large datasets in a flexible and intuitive manner.
Let's begin with setting up our environment and preparing our dataset.
Step 1: Set Up the Environment
First, we need to ensure that we have all the necessary tools and libraries installed. We'll be using pandas extensively for data manipulation. Here’s how you start:
# Import the pandas library for data manipulation and analysis
import pandas as pd
# Install pandas library using pip (Python package installer)
%pip install pandas
# Note: The second import statement is redundant if you've already installed and imported pandas once. If that's the case, you can omit it.
Step 2: Import the Dataset
Before you can import the dataset, make sure you've downloaded it from the provided link on Kaggle: Top Rated TMDb Movies - 10k Dataset. This dataset is a rich source of information on movies and will serve as the foundation for our recommendation system.
Once you have the dataset, load it into a pandas DataFrame. Assuming the dataset is in a CSV file, you can use the following code:
# Load the dataset into a pandas DataFrame
movies = pd.read_csv('path/to/your/downloaded/movies.csv')
Replace 'path/to/your/downloaded/movies.csv'
with the actual file path of the downloaded dataset on your system.
Step 3: Understand the Dataset
To get a sense of the data we're working with, let's inspect the names of the columns in our DataFrame. This will help us identify which columns are relevant to our recommendation system.
# Get the names of columns in the DataFrame
print(movies.columns)
Step 4: Select Relevant Features
For our movie recommendation system, we'll focus on a few key features: id
, title
, overview
, and genre
. Here's why we choose these features:
- ID: Essential for uniquely identifying each movie in our dataset.
- Title: Allows users to search and identify movies by their names.
- Overview: Provides a brief description of the movie’s plot, which is crucial for understanding its content and theme.
- Genre: Helps categorize movies into different types, enabling genre-based recommendations and personalization.
By focusing on these features, we can leverage the narrative content and genre information of the movies to find similarities and make recommendations. Let’s select these columns and drop the rest:
# Select and retain only specified columns ('id', 'title', 'overview', 'genre') from the DataFrame
movies = movies[['id', 'title', 'overview', 'genre']]
This step simplifies our dataset, leaving us with only the information we need for building our recommendation system.
Step 5: Combine Overview and Genre
Combining the 'overview' and 'genre' columns will create a more insightful feature vector, significantly improving the system's ability to recommend movies that closely match user preferences. This combination allows us to leverage both textual descriptions and genre categorizations, enriching the dataset with more context for each movie.
# Add a new column 'tags' by concatenating 'overview' and 'genre' columns
movies['tags'] = movies['overview'] + movies['genre']
Step 6: Simplify the Dataset
Now that we have a consolidated 'tags' column that captures the essence of each movie's content and genre, we can simplify our dataset further by removing the now-redundant 'overview' and 'genre' columns. This leaves us with a streamlined dataset focused on the most useful features for our recommendation system.
# Create a new DataFrame 'new_data' by dropping the 'overview' and 'genre' columns from 'movies'
new_data = movies.drop(columns=['overview', 'genre'])
Step 7: Prepare Text Data for Machine Learning
To leverage machine learning algorithms for our recommendation system, we need to convert our textual data into a numerical format.
This is where the CountVectorizer
comes into play. It transforms the text in our 'tags' column into a sparse matrix of token counts, effectively converting words into vectors.
But before we can apply CountVectorizer
, it's crucial to clean the text data to improve the quality of our vectors. This typically involves lowercasing the text, removing punctuation, and applying stemming or lemmatization to reduce words to their base or root form.
While the prompt mentions reapplying a clean_text
function to the 'tags' column, it seems we haven't defined such a function yet. Assuming we have a function to clean our text, it would look something like this:
# Assuming 'clean_text' is a function that cleans the text data
new_data['tags_clean'] = new_data['tags'].apply(clean_text)
Let's proceed with vectorizing our cleaned 'tags' data:
# Import CountVectorizer from scikit-learn for text vectorization
from sklearn.feature_extraction.text import CountVectorizer
# Note: Ensure that scikit-learn is installed. If not, use pip to install it.
# pip install scikit-learn
# Initialize CountVectorizer
cv = CountVectorizer(max_features=5000, stop_words='english')
# Vectorize the cleaned 'tags' text
vectorized_data = cv.fit_transform(new_data['tags_clean']).toarray()
In this code snippet, max_features=5000
limits the number of features (that is, distinct words) to the top 5000, which helps in managing computational complexity. Specifying stop_words='english'
removes common English words that are unlikely to contribute to the uniqueness of the movie descriptions.
Step 8: Calculate Cosine Similarity
With our movie descriptions transformed into numerical vectors, the next critical step is to calculate the cosine similarity between these vectors.
This measurement will allow us to determine how similar any two movies are based on their content. A cosine similarity score of 1 means the movies are very similar, while a score of 0 indicates no similarity.
Import Library and Calculate Similarity
# Import cosine_similarity from scikit-learn for computing similarity between vectors
from sklearn.metrics.pairwise import cosine_similarity
# Calculate the cosine similarity between vectors
similarity = cosine_similarity(vectorized_data)
Here, cosine_similarity
function takes the array of vectors (our vectorized movie tags) as input and returns a matrix of similarity scores between all pairs of movies.
Testing with a Specific Movie
To understand how our recommendation system works in practice, let's find and print out the titles of movies most similar to a specific movie in our dataset. We'll use the first movie as an example:
# Calculate similarity scores for the third movie with all other movies, sort them, and store the result
distance = sorted(list(enumerate(similarity[4])), reverse=True, key=lambda vector: vector[1])
# Print the titles of the first five movies most similar to the third movie
for i in distance[0:5]:
print(new_data.iloc[i[0]].title)
Define a Recommendation Function
To make our system user-friendly, we'll encapsulate the logic for finding similar movies into a function. This function will take a movie title as input and print the titles of the top 5 similar movies:
# Define a function to recommend the top 5 similar movies for a given movie title
def recommend(movies):
# Find the index of the given movie in the DataFrame
index = new_data[new_data['title'] == movies].index[0]
# Calculate similarity scores, sort them, and print titles of the top 5 similar movies
distance = sorted(list(enumerate(similarity[index])), reverse=True, key=lambda vector: vector[1])
for i in distance[1:6]: # start from 1 to skip the movie itself
print(new_data.iloc[i[0]].title)
# Example usage
recommend("Iron Man")
Note: The example provided uses "Iron Man"
as a placeholder. Replace it with an actual movie title from your dataset to test the function.
Step 9: Serialize the Dataframe and Similarity Matrix
To save our progress and easily share or deploy our recommendation system, we'll serialize the cleaned dataset and the similarity matrix using the pickle
module:
import pickle
# Serialize and save the 'new_data' DataFrame and 'similarity' matrix to files
pickle.dump(new_data, open('movies_list.pkl', 'wb'))
pickle.dump(similarity, open('similarity.pkl', 'wb')) # Fixed typo: should use 'wb' for writing
# Optionally, deserialize to verify
# movies_list = pickle.load(open('movies_list.pkl', 'rb'))
# similarity_loaded = pickle.load(open('similarity.pkl', 'rb'))
Verify the Environment
Finally, to understand where our serialized files are saved, or if you're working in an environment where the file path matters (like a server or a deployment scenario), you can print the current working directory:
import os
# Print the current working directory
print(os.getcwd())
This concludes the step-by-step guide on building a basic movie recommendation system. You now have a functional system that can recommend movies based on similarity in content and genre, with the infrastructure to serialize your model for future use or deployment.
In the next chapter, you'll see the full Python code, all put together.
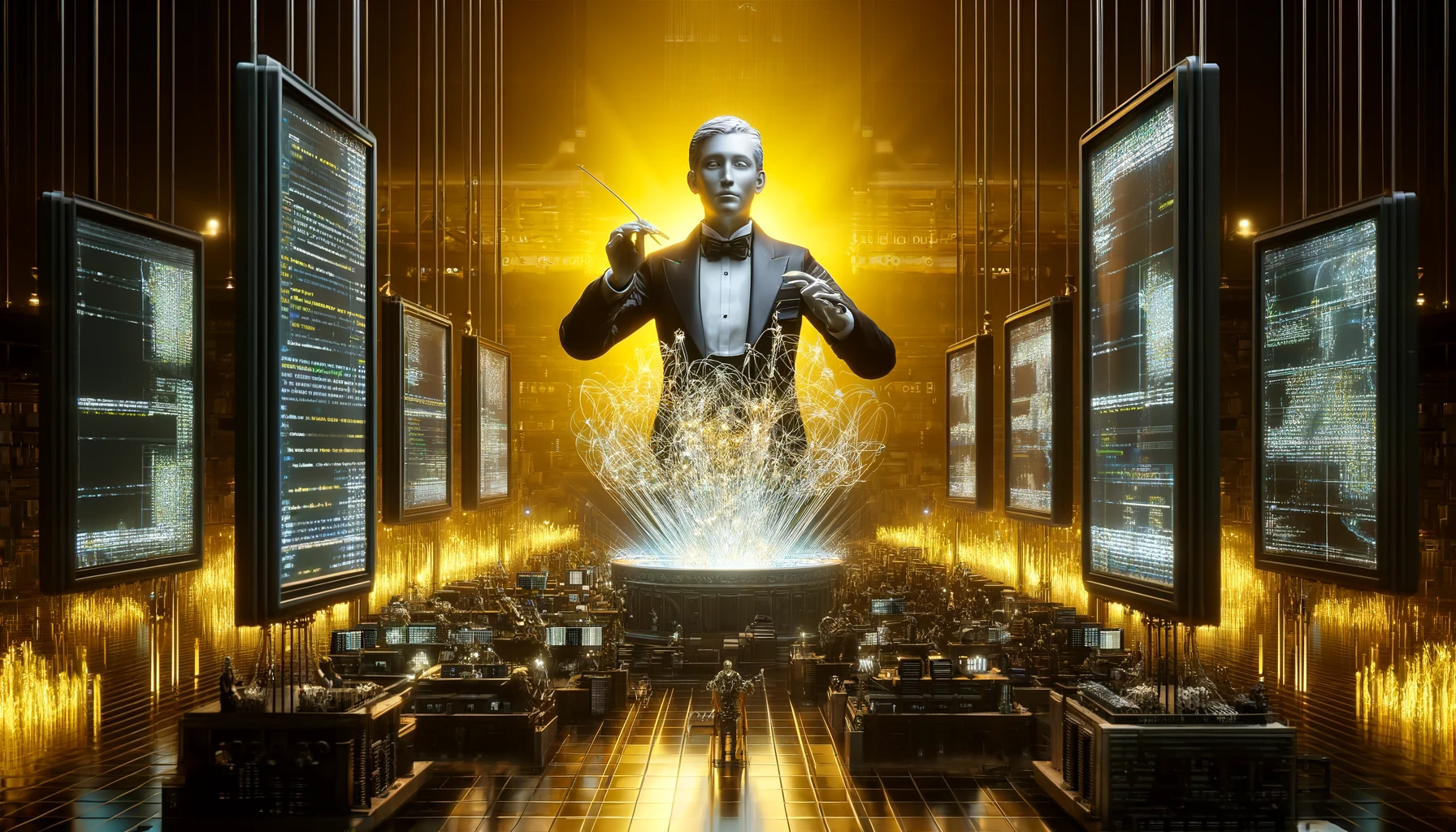
Full JupyterNotebook Code
# Import the pandas library for data manipulation and analysis
import pandas as pd
# Install pandas library using pip (Python package installer)
%pip install pandas
# Import the pandas library again (this line is redundant and can be omitted)
import pandas as pd
# Load a dataset from a CSV file into a pandas DataFrame
movies = pd.read_csv('dataset.csv')
# Display the first 10 rows of the DataFrame
movies.head(10)
# Generate descriptive statistics of the DataFrame
movies.describe()
# Print a concise summary of the DataFrame (information about columns, data types, non-null values, etc.)
movies.info()
# Calculate the sum of null (missing) values for each column in the DataFrame
movies.isnull().sum()
# Get the names of columns in the DataFrame
movies.columns
# Select and retain only specified columns ('id', 'title', 'overview', 'genre') from the DataFrame
movies = movies[['id', 'title', 'overview', 'genre']]
# Add a new column 'tags' by concatenating 'overview' and 'genre' columns
movies['tags'] = movies['overview'] + movies['genre']
# Create a new DataFrame 'new_data' by dropping the 'overview' and 'genre' columns from 'movies'
new_data = movies.drop(columns=['overview', 'genre'])
# Import necessary modules from the NLTK library for text processing
import nltk
import re
from nltk.corpus import stopwords
from nltk.stem import WordNetLemmatizer
from nltk.tokenize import word_tokenize
# Download NLTK resources for tokenization, lemmatization, and stopwords
nltk.download('punkt')
nltk.download('wordnet')
nltk.download('stopwords')
# Define a function for cleaning text data
def clean_text(text):
# Return an empty string if text is not a string
if not isinstance(text, str):
return ""
# Convert text to lowercase
text = text.lower()
# Remove punctuation while retaining words and digits
text = re.sub(r'[^\\w\\s\\d]', '', text)
# Tokenize the text into words
words = word_tokenize(text)
# Define English stopwords
stop_words = set(stopwords.words('english'))
# Remove stopwords from the tokenized words
words = [word for word in words if word not in stop_words]
# Initialize the WordNet lemmatizer
lemmatizer = WordNetLemmatizer()
# Lemmatize each word
words = [lemmatizer.lemmatize(word) for word in words]
# Join the words back into a single string
text = ' '.join(words)
return text
# Apply the clean_text function to the 'tags' column of 'new_data' and store the result in 'tags_clean'
new_data['tags_clean'] = new_data['tags'].apply(clean_text)
# Import CountVectorizer from scikit-learn for text vectorization
from sklearn.feature_extraction.text import CountVectorizer
# Install scikit-learn library using pip
pip install scikit-learn
# Reapply the clean_text function to the 'tags' column (this line seems redundant)
new_data['tags_clean'] = new_data['tags'].apply(clean_text)
# Import train_test_split from scikit-learn for splitting data into training and test sets
from sklearn.model_selection import train_test_split
# Import CountVectorizer again (redundant import)
from sklearn.feature_extraction.text import CountVectorizer
# Initialize a CountVectorizer object with a maximum of 10,000 features and English stop words
cv = CountVectorizer(max_features=10000, stop_words='english')
# Fit the CountVectorizer to the 'tags_clean' column and transform the text data into a numerical vector representation
vector = cv.fit_transform(new_data['tags_clean'].values.astype('U')).toarray()
# Check the shape of the resulting vector
vector.shape
# Import cosine_similarity from scikit-learn for computing similarity between vectors
from sklearn.metrics.pairwise import cosine_similarity
# Calculate the cosine similarity between vectors
similarity = cosine_similarity(vector)
# Print a concise summary of the 'new_data' DataFrame
new_data.info()
# Calculate similarity scores for the third movie with all other movies, sort them, and store the result
distance = sorted(list(enumerate(similarity[2])), reverse=True, key=lambda vector: vector[1])
# Print the titles of the first five movies most similar to the third movie
for i in distance[0:5]:
print(new_data.iloc[i[0]].title)
# Define a function to recommend the top 5 similar movies for a given movie title
def recommend(movies):
# Find the index of the given movie in the DataFrame
index = new_data[new_data['title'] == movies].index[0]
# Calculate similarity scores, sort them, and print titles of the top 5 similar movies
distance = sorted(list(enumerate(similarity[index])), reverse=True, key=lambda vector: vector[1])
for i in distance[0:5]:
print(new_data.iloc[i[0]].title)
# Call the recommend function with "Iron Man" as the argument
recommend("Iron Man")
# Import the pickle module for serializing Python objects
import pickle
# Serialize the 'new_data' DataFrame and save it to a file
pickle.dump(new_data, open('movies_list.pkl', 'wb'))
pickle.dump(new_data, open('similarity.pkl', 'wb'))
# Deserialize the 'movies_list.pkl' file back into a Python object
pickle.load(open('movies_list.pkl', 'rb'))
# Import the os module for interacting with the operating system
import os
# Print the current working directory
print(os.getcwd())

Challenges and Solutions in Machine Learning-based Recommendations
Implementing machine learning in movie recommendations presents distinct challenges such as handling sparse data, which occurs when there's insufficient information about users or movies.
A common instance of this is the 'cold start' problem, where new users or movies have little to no interaction data. Without enough user ratings or movie details, generating accurate recommendations is difficult.
Let's explore strategies to overcome these hurdles and build an adaptable recommendation system.
Strategies for 'Cold Start' Recommendations
- Content is King (At First): Focus on detailed descriptions, genre tags, cast/director popularity, or even visually-derived features (color palettes, cinematography style). Let your system find similar movies.
- The Power of Metadata: Look beyond surface-level similarity. Did the same production company release similar recent hits? Is the director known for a specific mood or filmmaking style?
- 'Exploration' Mode: Dedicate a small portion of recommendations to items with lower popularity scores or minimal user data. Injecting some calculated randomness promotes discovery and helps combat the "echo chamber" effect.
- Embrace Transparency: Communicate to users when recommendations are based on limited data ("We're still learning your taste, but you might enjoy this based on its cast..."). This builds trust and invites engagement.
Optimize & Evolve: Harness User Feedback & Testing
Incorporating your feedback directly into the development and refinement of your movie recommendation system can significantly boost its effectiveness and your satisfaction.
By integrating mechanisms to gather your responses and preferences, your needs are addressed more precisely, leading to more accurate and appreciated movie recommendations.
Here's why leveraging your feedback is pivotal for enhancing the accuracy of your movie recommendation system:
Feedback is Your Goldmine
- Immediate Relevance Feedback: Features like "Did you enjoy this movie?" buttons or options for you to rate recommendations allow the system to adjust its future suggestions in real-time, making them more aligned with your preferences.
- Deep Insight Through Surveys: Optional short surveys after you've watched a movie can provide insights into what specific aspects you liked or disliked (for example plot complexity, character development, genre preferences). This detailed feedback can be used to fine-tune the recommendation algorithms.
- Watchlist Tracking: Seeing which movies you add to your watchlists or what you watch next after a recommendation offers implicit feedback on your preferences, aiding in tailoring future suggestions more accurately.
A/B Test for the Win
- Balancing Safe vs. Exploratory Recommendations: A/B testing different strategies helps find the perfect balance between recommending movies that closely match your known preferences ("safe" choices) and introducing you to new content to expand your horizons (exploratory choices). Monitoring engagement metrics such as clicks, watch time, and ratings lets the system determine which approach keeps you engaged and satisfied over the long term.
Don’t Neglect the Classics
- Inclusive Recommendation Scope: A common pitfall of recommendation systems is focusing too much on new releases, potentially missing out on classics or older gems that might perfectly suit your taste. By incorporating the release year into the algorithms or deliberately reserving specific slots for classic films across various genres, the system can introduce you to a wider range of movies you might love but haven't yet discovered.
Integrating your feedback into a movie recommendation system not only optimizes the accuracy and relevance of the suggestions provided but also creates a dynamic learning environment for the system. It ensures that the recommendations evolve with your changing preferences, leading to a more engaging and satisfying experience for you.
This strategy turns you, the passive viewer, into an active participant in the curation process, significantly enhancing the system's overall effectiveness.
Expert Insight: The best systems proactively address sparse data. Continuously re-evaluate data sources, seek creative ways to gather some initial user preferences, and never assume your work is done.
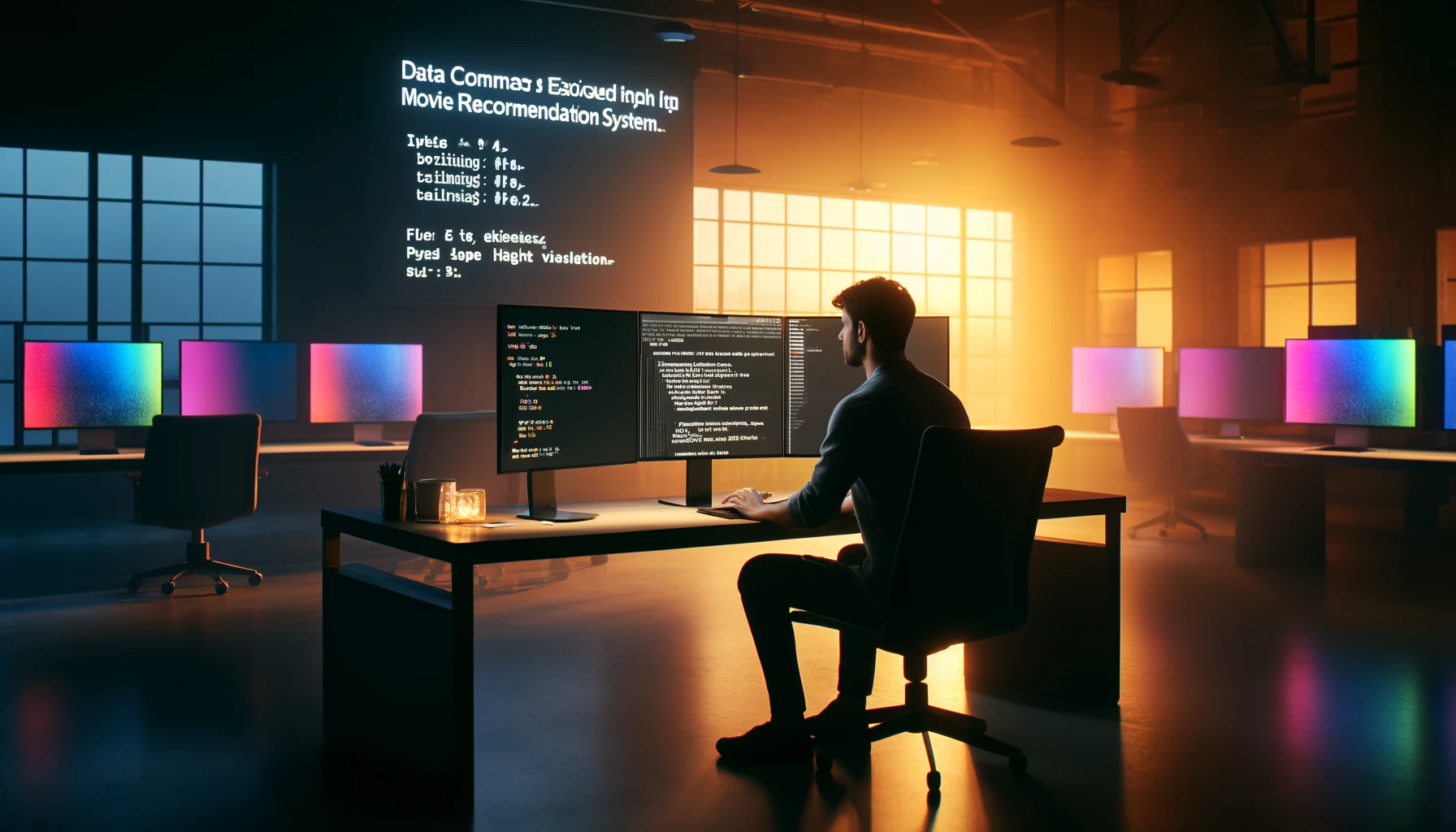
Limitations and Areas for Future Improvement
While the approach outlined for building a comprehensive movie recommendation system is robust and innovative, it's important to acknowledge the inherent limitations due to dataset constraints and the reliance on data from TMDB (The Movie Database).
Here's a clearer explanation of these limitations and their potential impact on the recommendation system:
Dataset Limitations
- Limited Columns and Depth: Our primary dataset, sourced from TMDB, might not have the breadth or depth of metadata needed for more nuanced recommendations. For instance, it may offer basic details like genre, release date, and cast but lack richer content such as detailed user reviews, specific subgenres, or thematic tags that could enhance personalization.
- Static Dataset: The data snapshot from TMDB represents a particular moment in time. As new movies are released and cultural trends shift, the dataset risks becoming outdated, potentially skewing recommendations toward older or less relevant content without regular updates.
Reliance on TMDB
- Data Completeness and Accuracy: While TMDB is a valuable resource, the completeness and accuracy of its data depend on user contributions and can vary. This variability might affect the reliability of the recommendations based on this data, especially for less popular or newer movies.
- Limited User Feedback Loop: TMDB provides a solid foundation for understanding what movies are available and their basic attributes. But it might not capture the full spectrum of user interactions and feedback, such as nuanced sentiment analysis or specific viewing patterns, which are crucial for refining recommendation algorithms.
Strategies to Mitigate Limitations
- Data Enrichment from Diverse Sources: To compensate for the limitations of the TMDB dataset, incorporating additional data sources is crucial. Aggregating ratings and reviews from platforms like Kaggle or MovieLens, mining discussions from Reddit or Letterboxd, and including awards data can enrich the dataset, offering a more rounded view of movies and viewer preferences.
- Advanced Text and Visual Analysis: Utilizing advanced NLP techniques for deeper text analysis and exploring visual cues from movie posters or trailers can add layers of understanding beyond basic metadata, helping to uncover latent connections between movies and viewer preferences.
- Dynamic Algorithm Updates: Regularly updating the recommendation algorithms to integrate new data sources, user feedback, and evolving trends ensures the system remains relevant and effective over time. This includes refining collaborative filtering models to address data sparsity and developing contextual models that reflect current viewing contexts and moods.
Recognizing the limitations of relying primarily on TMDB data and the current dataset's scope underscores the importance of ongoing efforts to enrich and update the dataset and algorithms.
By acknowledging these challenges and actively seeking to mitigate them through strategic data sourcing, advanced analysis, and algorithm evolution, the recommendation system can continue to improve, offering more accurate, personalized, and timely movie suggestions to users.
Thank You for Reading!
As we wrap up this tutorial, I extend my gratitude for your time. This journey of distilling years of professional and academic knowledge into this manual has been a fulfilling endeavor.
Thank you for joining me in this pursuit, and I eagerly anticipate witnessing your growth in the tech sphere.
Resources
If you're keen on mastering data structures, check out LunarTech.AI's Data Structures Mastery Bootcamp. It's perfect for those interested in AI and machine learning, focusing on effective use of data structures in coding.
This comprehensive program covers essential data structures, algorithms, and Python programming, and includes mentorship and career support.
Additionally, for more practice in data structures, explore these resources on our website:
- Java Data Structures Mastery - Ace the Coding Interview: A free eBook to advance your Java skills, focusing on data structures for enhancing interview and professional skills.
- Foundations of Java Data Structures - Your Coding Catalyst: Another free eBook, diving into Java essentials, object-oriented programming, and AI applications.
Visit our website for these resources and more information on the bootcamp.
About the Author
Vahe Aslanyan here, at the nexus of computer science, data science, and AI. Visit vaheaslanyan.com to see a portfolio that's a testament to precision and progress. My experience bridges the gap between full-stack development and AI product optimization, driven by solving problems in new ways.
With a track record that includes launching a leading data science bootcamp and working with industry top-specialists, my focus remains on elevating tech education to universal standards.