Helper functions in React.js are like your trusty assistants, and they play a crucial role in simplifying complex tasks. When these functions are well-structured, they make your code easier to read, maintain, and reuse.
In this article, we'll explore the significance of helper functions in React.js projects. We'll also see how their organized use can enhance your code's clarity, make it easier to manage over time, and allow you to recycle your code for efficiency.
Prerequisites:
Before diving into helper functions, you should have a fundamental grasp of JavaScript and React.js concepts, including components, state, and props. This knowledge will provide a solid foundation for understanding and implementing helper functions effectively.
The Role of Helper Functions in React.js
In this section, we'll explore the fundamental role of helper functions in React.js and why they are indispensable for efficient development.
We'll delve into the everyday scenarios where these functions can make complex tasks much simpler. We'll also explore how using helper functions not only improves the organization of your code but also eases the debugging process.
What Are Helper Functions?
Helper functions are small, reusable JavaScript functions that assist your React components in performing specific tasks. They are like specialized tools that simplify your code by breaking down complex operations into manageable steps.
These functions are often separate from your main components and can be called whenever needed.
// Example of a simple helper function
function calculateTotalPrice(quantity, price) {
return quantity * price;
}
In React development, you often encounter tasks that require multiple steps or computations. Helper functions shine in these situations.
For instance, you might need to format dates, validate user input, or fetch data from an API. By creating helper functions for these tasks, you can write cleaner and more concise code.
// Example of a helper function to format a date
function formatDate(date) {
return new Date(date).toLocaleDateString();
}
Benefits of Helper Functions
Using helper functions offers several advantages, such as better code organization and more straightforward debugging.
When your code is organized into small, focused functions, it becomes easier to understand, update, and maintain. In addition, debugging becomes less daunting because you can isolate issues to specific helper functions, making it easier to identify and fix problems.
How to Plan Your Helper Functions
This section will emphasize the crucial step of planning before creating helper functions in a React project.
We'll explore why this preparatory stage is essential and how to identify tasks that can be abstracted into these functions. You'll also see some real-world examples of scenarios where helper functions prove their worth.
The Importance of Planning
Planning is the foundation of effective helper functions. Before creating them, you should take a moment to outline the tasks and challenges your project involves.
This planning process helps you anticipate the need for helper functions, ensuring that they align with your project's goals.
Criteria for Abstraction
To identify tasks that can be abstracted into helper functions, consider functions that are reusable, have a specific purpose, and can be logically isolated from your main components.
For instance, data validation, formatting, or calculations are prime candidates.
// Example: A task like validating an email address can be abstracted into a helper function.
function validateEmail(email) {
// Add validation logic here
return isValid;
}
When Helper Functions are Useful
Helper functions shine in situations where you need to perform similar operations in different parts of your application.
For example, consider a shopping cart application where you want to calculate the total price of items in multiple places. A helper function can help you do this consistently and without duplicating code.
// Example: Calculating the total price of items in a shopping cart
function calculateTotalPrice(cart) {
let totalPrice = 0;
for (const item of cart) {
totalPrice += item.price * item.quantity;
}
return totalPrice;
}
How to Write Helper Functions
In this section, we'll cover the best practices for writing helper functions in a way that makes your code clean and easy to work with.
Naming Conventions
Choose clear and descriptive names for your helper functions. A good name should indicate what the function does. Here's an example:
// Example: Naming a function that capitalizes the first letter of a string
function capitalizeFirstLetter(str) {
// Function logic here
}
Function Parameters and Return Values
Design your functions to accept necessary parameters and return meaningful values. This helps keep your functions focused and reusable.
// Example: A function that adds two numbers
function addNumbers(a, b) {
return a + b;
}
How to Avoid Side Effects
Try to avoid changing data outside of the function's scope. This makes your functions predictable and easier to reason about.
// Example: A function that doesn't have side effects
function doubleArrayValues(arr) {
const doubled = arr.map((item) => item * 2);
return doubled;
}
By following these best practices, you'll create helper functions that are easy to understand and integrate into your React project, enhancing its readability and maintainability.
How to Manage Helper Function Dependencies
In this section, we'll cover how to manage dependencies for your helper functions, including React components or external libraries.
How to Handle Dependencies
When your helper functions rely on other parts of your application, like React components or external libraries, make sure to import them at the beginning of your file. This way, your helper functions have access to what they need.
Here's what I mean:
// Example: Importing a React component and using it in a helper function
import React, { useState } from 'react';
function useCounter() {
const [count, setCount] = useState(0);
// Function logic here
}
Pros and Cons of Dependency Management
There are different strategies for managing dependencies. A pro of importing dependencies at the beginning of your file is that it's clear what your helper function relies on. But a con is that it can make your code file longer if you have many dependencies.
Another approach is to pass dependencies as function parameters, which can make your functions more modular.
// Example: Passing dependencies as function parameters
function useCounter(setCountFunction) {
// Function logic here
}
Choosing the right strategy depends on your project's complexity and your preference.
How to Test Helper Functions
In this section, we'll highlight why testing your helper functions is crucial to ensure they function correctly. We'll also discuss popular testing tools like Jest and React Testing Library, and provide simple code examples for writing tests.
Importance of Testing
Testing your helper functions is vital to make sure they do what you expect. It helps catch bugs early, prevents unexpected behavior, and provides confidence that your code works as intended, even as you make updates.
Testing Tools and Frameworks
Popular tools like Jest and React Testing Library are excellent choices for testing your React code. They provide simple and effective ways to write and run tests.
How to Write Your Tests
Here's a basic example of testing a helper function using Jest. In this case, we're testing the addNumbers
function from the section on "How to Write Helper Functions":
// Import the function you want to test
const { addNumbers } = require('./your-helper-functions');
// Write a test case
test('adds two numbers correctly', () => {
expect(addNumbers(2, 3)).toBe(5); // Check if the function returns the expected result
});
Writing tests like this allows you to confirm that your helper functions work correctly and continue to do so as your project evolves.
Documentation and Comments
In this section, you'll learn why of clear and comprehensive documentation is important for helper functions. I'll also explain how it benefits developers and future maintainers. Then we'll look at some guidelines for writing effective comments and documentation.
Significance of Documentation
Documentation is essential because it helps developers understand how to use your helper functions. Well-documented code serves as a guide, reducing the learning curve for new developers and ensuring that existing developers don't forget how the functions work over time.
Clear documentation benefits developers by providing insights into what a helper function does, what parameters it expects, and what it returns. This leads to faster development, fewer errors, and more maintainable code.
For future maintainers, comprehensive documentation is invaluable for understanding and updating code without breaking existing functionality.
Guidelines for Comments and Documentation
Here are some simple guidelines for writing comments and documentation:
/**
* A helper function that calculates the total price.
*
* @param {number[]} prices - An array of item prices.
* @returns {number} The total price of all items.
*/
function calculateTotalPrice(prices) {
return prices.reduce((acc, price) => acc + price, 0);
}
How to Organize Helper Functions
In this section, we'll discuss strategies for effectively organizing your helper functions within your React project. Proper organization makes your codebase more manageable as it scales.
Strategies for Organization
Organizing your helper functions is crucial to keep your project clean and maintainable.
Consider creating a dedicated directory for your helper functions. You can structure it based on functionality, where related functions are grouped together.
src/
|-- components/
|-- helperFunctions/
|-- dataManipulation/
|-- formatDate.js
|-- calculateTotalPrice.js
|-- validation/
|-- validateEmail.js
|-- validatePassword.js
For smaller projects, you may opt for utility files that contain multiple helper functions in one file. But as your project grows, organizing them into separate files within directories becomes more efficient.
Naming Conventions
Follow clear and consistent naming conventions for your directories and files. This makes it easy for you and other developers to locate specific helper functions.
For instance, use descriptive names like dataManipulation
or validation
for directories and camelCase for file names.
Reusability and Sharing
In this section, we'll delve into the concept of reusability in helper functions and how to make them available for broader use.
Reusability in Helper Functions
Reusability is a key concept in helper functions. These functions are designed to be reused in multiple parts of your project. By writing functions that perform specific, commonly needed tasks, you can avoid duplicating code and simplify maintenance.
How to Make Helper Functions Available Internally
To use helper functions in multiple parts of your project, simply import them as needed.
For instance, if you have a utility file with helper functions, import those functions into various components or modules where they are required.
import { formatDate, calculateTotalPrice } from './helperFunctions';
How to Share Helper Functions Externally
If you want to share your helper functions with others or use them in different projects, you can package them as an npm module or publish them on a code-sharing platform like GitHub. This way, they become accessible to the open-source community and can be easily integrated into various projects.
By focusing on reusability and sharing, you maximize the value of your helper functions, making them available for use in multiple parts of your project and beyond. This promotes code efficiency and collaboration with others in the
Performance Considerations
In this section, we'll discuss essential performance considerations when working with helper functions.
How to Write Efficient Helper Functions
When writing helper functions, consider potential performance bottlenecks. In situations where your functions are called frequently or work with large datasets, inefficient code can slow down your application. Optimize algorithms and data structures to improve performance.
Profiling and Measuring
Profiling and measuring are essential techniques to ensure that your helper functions and overall codebase perform optimally.
Profiling helps you analyze how different parts of your code consume resources, allowing you to pinpoint performance bottlenecks and focus your optimization efforts where they are needed most. Measuring involves quantifying the time taken by specific operations.
Here's a simplified explanation and a basic example of how you can profile your code using the Chrome DevTools performance tab:
- Open Chrome DevTools by right-clicking on your web page and selecting "Inspect."
- Go to the "Performance" tab.
- Start recording the performance by clicking the record button.
- Interact with your application and perform the actions you want to profile.
- Stop recording.
- Review the performance analysis report to identify bottlenecks and areas that need optimization.
Check out this link below for more on using chrome dev tools for analysis:
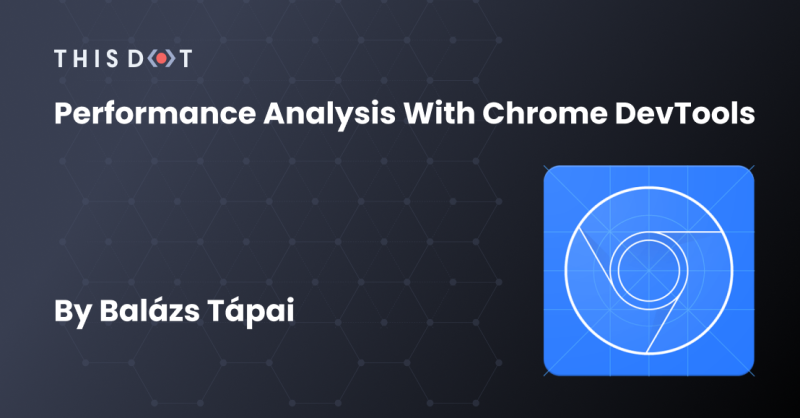
You can identify the performance bottlenecks, such as functions consuming excessive CPU or causing re-renders.
Common profiling and measuring tools include:
- Chrome DevTools: Built into Google Chrome, DevTools provides detailed insights into JavaScript and rendering performance.
- React DevTools: A browser extension for profiling React applications specifically.
- Lighthouse: An open-source tool for auditing web page performance and generating performance reports.
- Webpack Bundle Analyzer: For visualizing the size and composition of your application bundles.
- Jest and React Testing Library: These testing tools can measure the performance of your unit and integration tests.
These tools help you dig deeper into your code's performance and identify areas where optimization can yield the most significant benefits.
The Importance of Optimization
Efficient helper functions not only improve performance but also enhance the overall user experience. In a React project, fast and responsive applications are crucial.
Optimizing data and algorithms is crucial for improving the efficiency and performance of your applications. The best approach involves a number of key strategies:
- Analyze and Profile: Begin by measuring and profiling your code to identify performance bottlenecks. Tools like Chrome DevTools can help you pinpoint areas that need optimization.
- Select Efficient Data Structures: Choose the right data structures for your specific use case. For example, use maps for fast key-based access or arrays for indexed data.
- Algorithms Matter: Ensure that the algorithms you use are well-suited to the problem you're solving. Sometimes, a more efficient algorithm can drastically improve performance.
- Minimize Unnecessary Work: Avoid redundant calculations or unnecessary data processing. Cache results when appropriate to prevent recomputation.
- Batch Operations: Instead of processing items one by one, consider batch operations. For example, use the
map
function instead of afor
loop for operations on arrays. - Lazy Loading: Load data and perform computations only when they are needed, rather than preloading everything upfront.
- Use Memoization: Implement memoization for expensive function calls. This technique stores previously computed results and returns them if the same input is encountered again.
- Minimize Re-renders: In a React project, optimize component rendering to reduce unnecessary re-renders. Use React's
memo
orPureComponent
to prevent re-renders when props and state haven't changed. - Asynchronous Operations: Move time-consuming operations to web workers or use asynchronous processing to prevent blocking the main thread.
- Testing and Benchmarking: Continuously test and benchmark your code to ensure that optimizations don't introduce new issues or regressions.
- Prioritize Based on Impact: Focus your optimization efforts on the most critical and frequently used parts of your application. Don't spend excessive time optimizing code with minimal impact on performance.
- Documentation and Comments: Document your optimization efforts, including reasons and changes made, to help other developers understand and maintain the code.
Optimizing data and algorithms is an ongoing process that requires a balance between readability and performance. Regularly measuring and profiling your code, along with following best practices, will help you achieve optimal performance without sacrificing maintainability and readability.
By addressing performance considerations, you ensure that your helper functions do their job effectively without negatively impacting the user's interaction with your app.
Addressing performance considerations and optimizing your helper functions are essential steps to deliver a high-quality user experience and maintain the responsiveness of your React project.
Conclusion
Well-structured and well-documented helper functions are the unsung heroes of efficient React.js development. They simplify complex tasks, enhance code readability, and promote maintainability.
By following best practices, including clear documentation, organized organization, and thoughtful testing, you not only make your code easier to work with but also improve your development workflow.
Embrace the power of helper functions in your React.js projects, and you'll discover how these small yet mighty tools can make your coding life easier and more enjoyable while delivering robust and performant applications.