Imagine you're trying to find a needle in a haystack, but the haystack is the size of a mountain. That's what it can feel like to search for specific items in a massive dataset using Python.
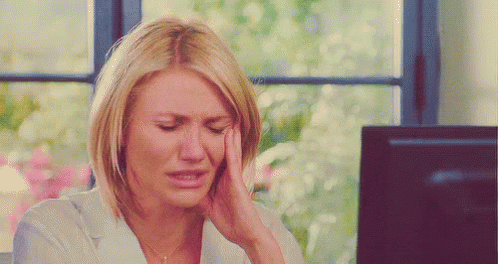
But fear not! With the right techniques, you can efficiently search and lookup information in large datasets without feeling like you're climbing Everest.
In this article, I'll show you how to take the pain out of search operations in Python. We'll explore a range of techniques, from using the built-in bisect module to performing a binary search, and we'll even throw in some fun with sets and dictionaries.
So buckle up and get ready to optimize your search operations on large datasets. Let's go!
Method 1: Linear Search in Python
The simplest way to search for an item in a list is to perform a linear search. This involves iterating through the list one element at a time until the desired item is found. Here is an example of a linear search:
def linear_search(arr, x):
for i in range(len(arr)):
if arr[i] == x:
return i
return -1
In the code above, we define the function linear search, which accepts two inputs: a list arr and a single item x. The function loops through the list, iterating through each element and comparing it to the desired item x. The function returns the item's index in the list if a match is found. In the absence of a match, the method returns -1.
Linear search has an O(n) time complexity, where n is the list length. This indicates that the time needed to conduct a linear search will increase proportionally as the size of the list grows.
Method 2: Binary Search in Python
If the list is sorted, we can perform a binary search to find the target item more efficiently. Binary search works by repeatedly dividing the search interval in half until the target item is found. Here is an example of a binary search:
def binary_search(arr, x):
low = 0
high = len(arr) - 1
while low <= high:
mid = (low + high) // 2
if arr[mid] < x:
low = mid + 1
elif arr[mid] > x:
high = mid - 1
else:
return mid
return -1
In the code above, we define the function binary search, which accepts as inputs a sorted list arr and a target item x. The low and high indices are used by the function to maintain a search interval.
A comparison between the target item x and the middle element of the search interval is performed by the function on each iteration of the loop.
The modified search interval omits the bottom half of the list if the middle element is less than x. The search interval is modified to omit the top half of the list if the middle element is greater than x. The function provides the item's index in the list if the middle element equals x.
If the desired item cannot be located, the function returns -1. Binary search has an O(log n) time complexity, where n is the list length. This means that, especially for big lists, binary search is substantially more effective than linear search.
Method 3: Search Using Sets in Python
If the order of the list is not important, we can convert the list to a set and use the in
operator to check whether an item is present in the set. Here is an example:
my_list = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
my_set = set(my_list)
if 5 in my_set:
print("5 is in the list")
else:
print("5 is not in the list")
In the above code, we define a list my_list
and convert it to a set my_set
. We then use the in
operator to check whether item 5 is present in the set. If the item is present, we print a message indicating that it is in the list. If the item is not present, we print a message indicating that it is not in the list.
Using sets for search operations can be very efficient for large lists, especially if you need to perform multiple lookups, as sets have an average time complexity of O(1) for the in
operator. But sets do not preserve the order of the elements, and converting a list to a set incurs an additional cost.
Method 4: Search Using Dictionaries in Python
If you need to associate each item in the list with a value or some other piece of information, you can use a dictionary to store the data. Dictionaries provide a fast way to look up a value based on a key. Here is an example:
students = {
"John": 85,
"Lisa": 90,
"Mike": 76,
"Sara": 92,
"David": 87
}
if "Lisa" in students:
print(f"Lisa's grade is {students['Lisa']}")
else:
print("Lisa is not in the class")
In the above code, we define a dictionary students
that associates the name of each student with their grade. We then use the in
operator to check whether the name "Lisa" is in the dictionary, and if so, we print her grade.
Dictionaries provide an average time complexity of O(1) for lookups based on the key, which makes them very efficient for large datasets. But, dictionaries do not preserve the order of the items, and there is an additional cost associated with creating the dictionary.
Conclusion
Searching and looking up info in large datasets can be a daunting task, but with the right tools and techniques, it doesn't have to be. By applying the methods we've covered in this article, you can efficiently navigate massive datasets with ease and precision.
From the built-in bisect module to the powerful capabilities of sets and dictionaries, Python offers a range of efficient and versatile options for finding and retrieving data. By combining these techniques with smart programming practices and optimization strategies, you can create lightning-fast search operations that can handle even the largest datasets.
So don't let big data intimidate you. With a little bit of creativity, a lot of perseverance, and the techniques we've explored in this article, you can conquer any search challenge and emerge victorious. Happy searching!