by Arun Mathew Kurian
A Quick Look at Action Text for Rails 6.0
Rails 6.0 is almost here. The stable version will be released on April 30. The Rails 6.0 beta1 was released on January 15. As Rails releases always are, Rails 6.0 is also action-packed. There are two major frameworks newly introduced, Action Mailbox and Action Text. In this post, let's take a quick look at Action Text by using it in a small app.
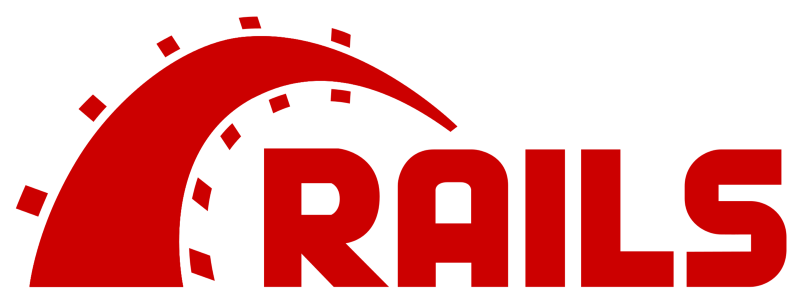
Action Text
Action Text allows us to bring rich text content and editing to Rails. This means we can perform operations like formatting text, embedding images, formatting links, adding lists and other editor-like feature to a text field.
This is done by including the Trix editor into the framework. The RichText content generated by the Trix editor is saved in its own RichText model that’s associated with any existing Active Record model in the application. All embedded images or other attachments are automatically stored using Active Storage.
Let us start building our Rails app which will be a blogger app. The app is created in Rails 6.0, so the ruby version must be >2.5.
In the terminal type
rails new blog --edge
The -- edge flag fetches the latest rails version or edge version of the rails.
Action Text expects web packer and ActiveStorage to be installed. The active storage is already present in the Rails app. So we need
gem “image_processing”, “~> 1.2” #uncomment from Gemfilegem ‘webpacker’
in the gem file.
Now run
bundle install.
Next, we need to create a config/webpacker.yml:
bundle exec rails webpacker:install
Now let us start our Rails Server.
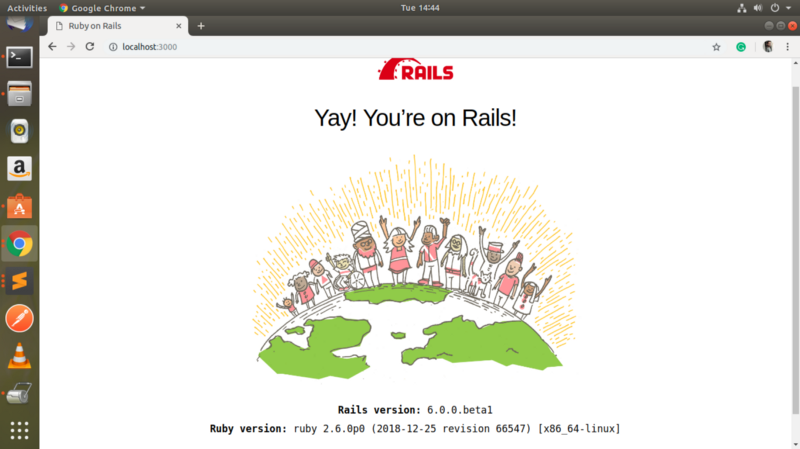
Great, let’s quickly build our app. The app will have only one model Article.
Let us create a controller for articles:
rails g controller Articles index new create show edit update destroy — no-helper — no-test-frameworks
And then configure our routes:
Rails.application.routes.draw do resources :articlesend
Next, we need to create our model. Our Articles model will have two fields: they are title and text. text must be the field that accepts Rich Text Format. So in the migration, we need to add only the title field. The text field will be handled by ActionText.
Let’s generate the model
rails g model Articles title:string — no-test-framework
and run the migrations:
rails db:migrate
Now let us add ActionText part. For that first run
rails action_text:install
This will add all the dependencies required by Action Text. Most notably, this will add a new file javascript/packs/application.js and two action-storage migrations.
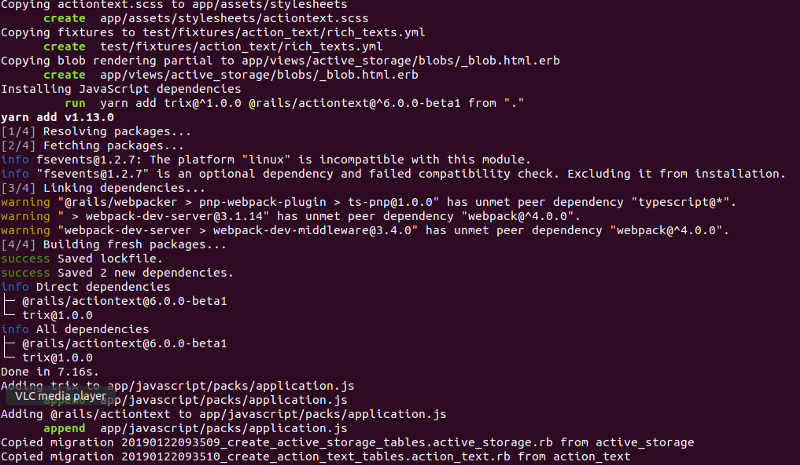
Run the migrations again using
rails db:migrate
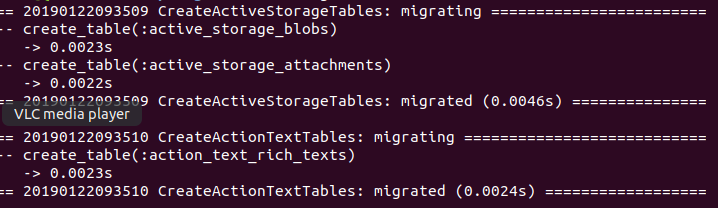
Now we can add the text part of our model. Go to app/models/article.rb and add the following line
has_rich_text :text
text is the field name we are providing. It can be anything like body or content etc.
Now our model becomes
class Article < ApplicationRecord has_rich_text :textend
Before we build our form, let’s create our controller logic for the creation of articles:
class ArticlesController < ApplicationController def create @article = Article.new(article_params) @article.save redirect_to @article end
private def article_params params.require(:article).permit(:title, :text) end
end
We can now create the form for the blog. In app/views/articles/new.rb add the following form code:
<%= form_with scope: :article, url: articles_path, local: true do |form| %>
<p> <%= form.label :title %><br> <%= form.text_field :title %> </p> <p> <%= form.label :text %><br> <%= form.rich_text_area :text %> </p> <p> <%= form.submit %> </p><% end %>
Notice that for text field we are using form.rich_text_area which is provided by Action Text.
Let us render our page:
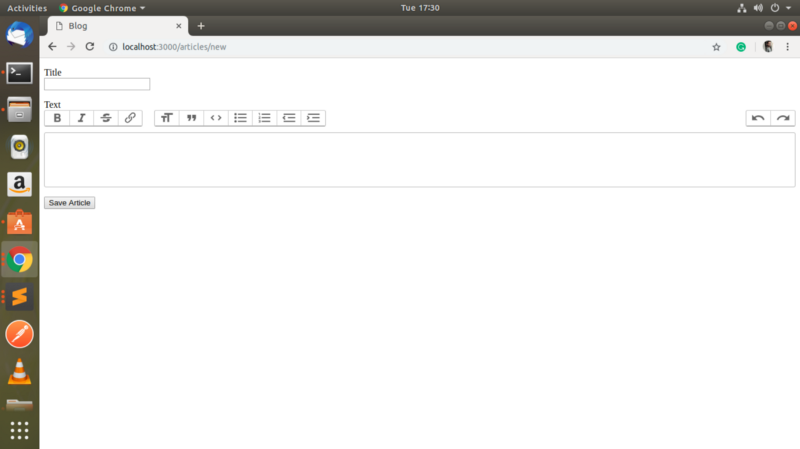
Awesome!!
We now have an awesome text editor for creating our article.
Before we start playing with the editor, let’s quickly implement the show functionality of the blog, so that we can see the articles we have created.
In app/controllers/articles_controller.rb add the following function:
def show @article = Article.find(params[:id]) end
Also, we need to create a view for this.
Create the file app/views/articles/show.html.erb and add the following code:
<p>Article Title:<strong><%= @article.title %></strong></p><p>Article Text:<strong><%= @article.text %></strong></p>
<%= link_to ‘Create new’,new_article_path %>
That's it. Our app is done. Now let's check the various features available in the text editor provided by ActionText.
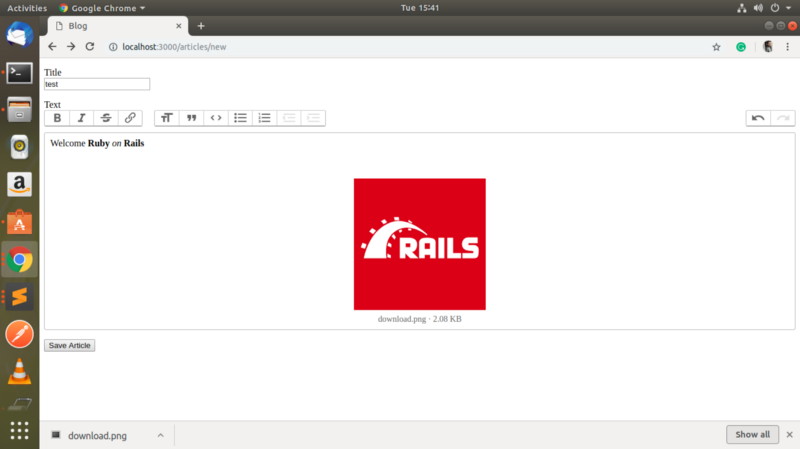
We can see that ActionText provides almost all the functionalities of a normal rich text-editor like formatting the text as bold, italic, adding strike-throughs, quotes, links, dragging and dropping images, etc.
After saving this, we can see the saved post from the show page.
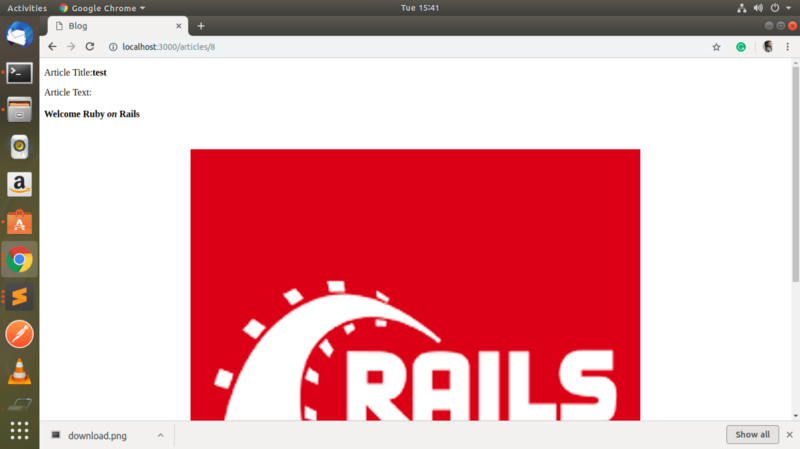
Great!
This is a very small example that displays the potential of ActionText. Hope it was helpful. Do give it a try.
A vast majority of web apps deal with rich content in some way. So I believe this new feature of Rails can make the lives of many developers easier.
Kudos to DHH and all the awesome people behind this.
https://github.com/amkurian/Rails-6.0_action_text_demo
Some Useful Links:
Action Text Overview - Ruby on Rails Guides
Action Text OverviewThis guide provides you with all you need to get started in handling rich text content.After…edgeguides.rubyonrails.org