In this article, I'm going to explain to you how the DOM works so that you have a clear picture of why JavaScript is needed to develop a web application.
First, let's recap what we know about HTML, CSS, and JavaScript.
How HTML, CSS, and JavaScript work
When you want to build a web application, you create an HTML document filled with HTML, CSS, and JavaScript.
These three technologies are used together like a team, each playing a different role in building what you see in your web browser.
HTML is a markup language used to define the structure and content of the page. The tags we use like <head>
, <body>
, and <button>
have meanings, and the browser will process these tags, rendering the content of the document to the screen
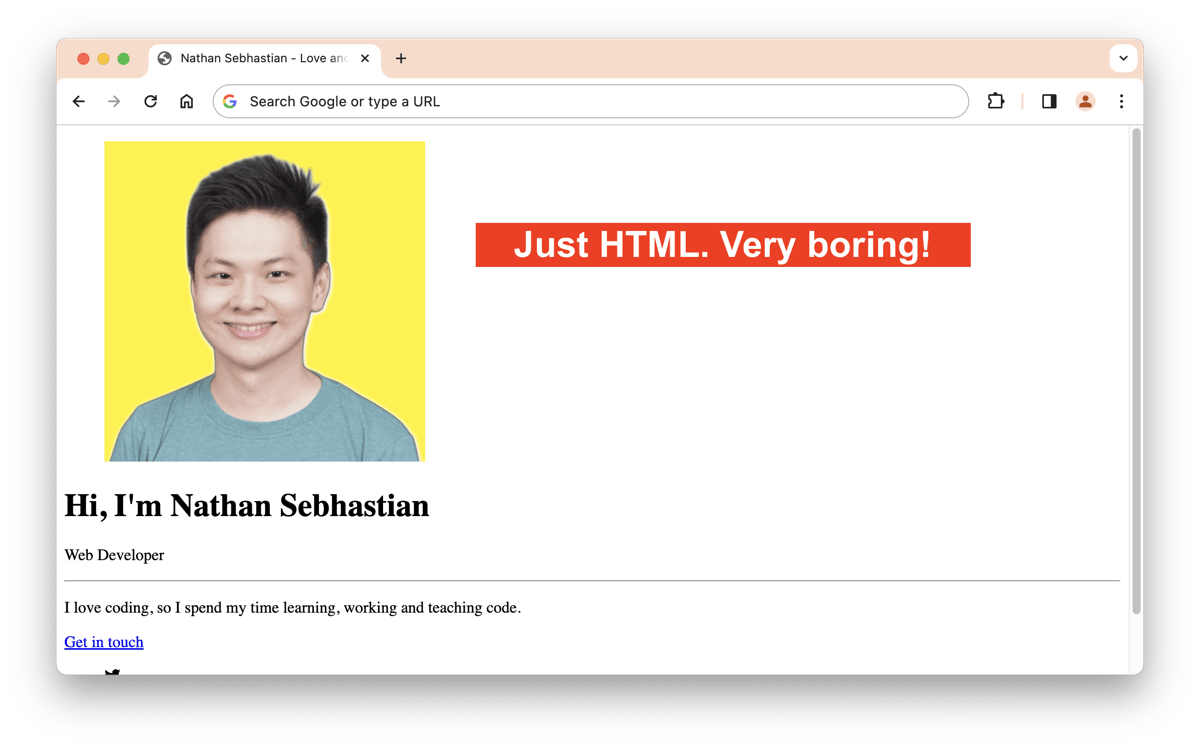
But the HTML alone produces a very boring and generic-looking page. CSS was created to enable us to change the way the HTML tags are represented on the browser.
By using CSS, you can make a button displayed in a color of your choice, or a text input box with a rounded border, making them more appealing and modern.

HTML and CSS are great partners, but even then, a web page is still just a passive thing. Once the browser processes the content and renders it, you can’t edit the page in any way.
You can’t change the background and text color of the page (like switching to dark mode, for example). You can’t create cool animations, turn on the microphone or webcam, or retrieve data from another website to display on the page after a click.
JavaScript is the programming language that’s supported by the browser, and it fills this gap left open by HTML and CSS.
In short, JavaScript breathes life into static websites, turning them dynamic. It allows a website to interact with the user, respond to their actions, and dynamically update the content without requiring a page reload.
The DOM Explained
So how come JavaScript can do things that HTML and CSS can’t do? Well, the answer is that JavaScript can manipulate the Document Object Model, or the DOM for short.
The DOM is a hierarchical structure composed of objects that make up a web page. Web browsers then expose this DOM so that you can change the page structure, style, and content using JavaScript.
For example, suppose you have the following HTML content in your document:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<h1>Hello World!</h1>
<p>This is a paragraph element</p>
</body>
</html>
The DOM graph generated by the HTML document above is as follows. I’m going to explain what the graph means below:

The DOM looks like a tree structure with a set of connected nodes. These nodes are objects that you can access using JavaScript. The html
node and its children are the content of your document.
For now, let’s focus on the window
and document
objects.
The Window Object
The window
object is the root of the DOM tree, and this object is used to instruct the browser to do tasks like:
- Displaying an alert box or a prompt
- Log messages or errors to the console
- Access the browser’s local storage
- Access the document object
You can access the window object from the Console by typing window
and pressing Enter. The browser will respond by showing you the methods and properties of the object as follows:
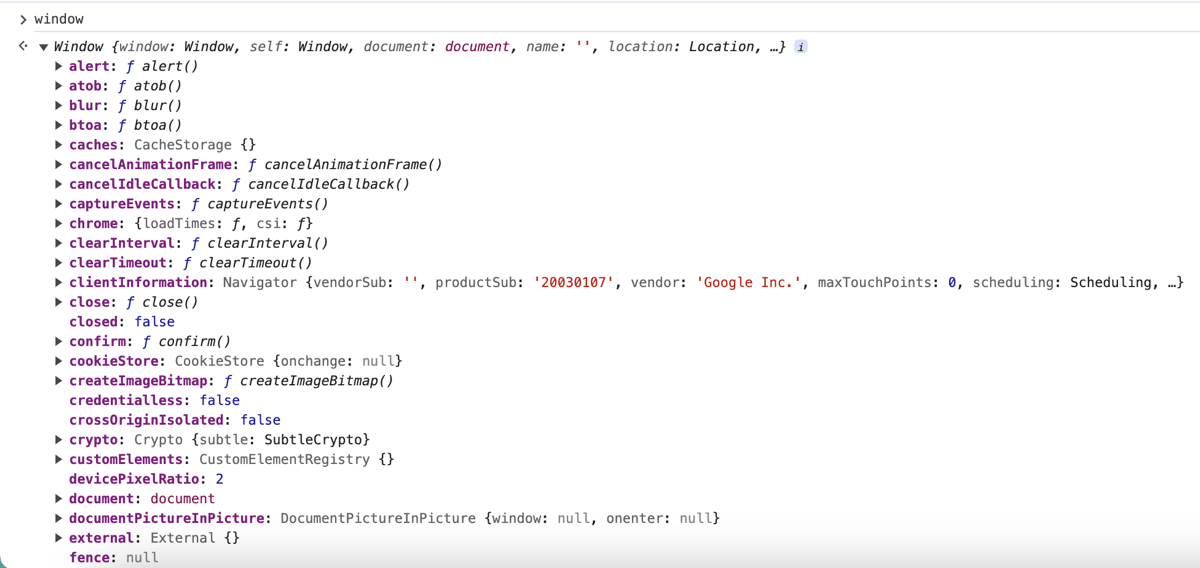
The window
object provides both child objects and methods that you’re going to access to manipulate a web page.
For example, the window
has the console
object that you can use to log a message to the console.
Type the code below to the console and hit Enter:
window.console.log('Hello World!');
You’ll see the string 'Hello World!' logged into the console.
The window
object is a global object, so you can omit it when calling its child object. To access the console
object, just type console as follows:
console.log('Hello World!');
The same goes for when you access the document
object. You can write document.propertyOrMethod
instead of window.document.propertyOrMethod
.
I won’t go too deep on the window
object, because as a web developer, you’re going to interact more with the document
object in your daily routine.
The Document Object
The document
object is the entry point for all HTML elements that you write in your document. This object is also what gives the Document Object Model its name.
It’s not called the Window Object Model because we’re mostly going to work with the Document object instead of the Window object.
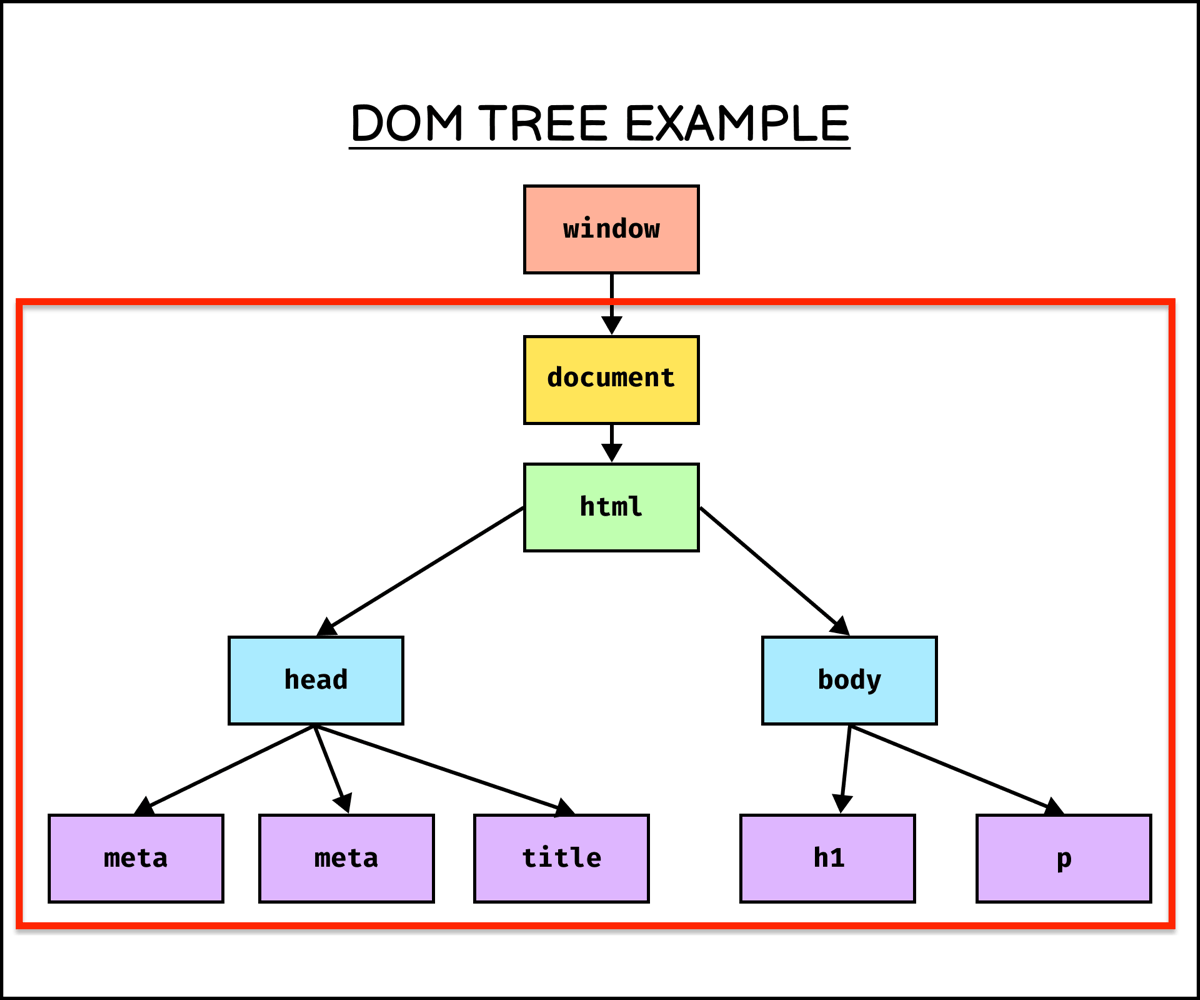
From the document object, you can retrieve any data related to the HTML content using JavaScript.
For example, you can get the Document title using the document.title
property, and the URL using document.URL
. The referrer is available in document.referrer
, the domain in document.domain
.
For the HTML document itself, you can access the whole HTML Element node using document.documentElement
, the document head using the document.head
property, or the body using document.body
You can try to access these elements from the console as shown below:
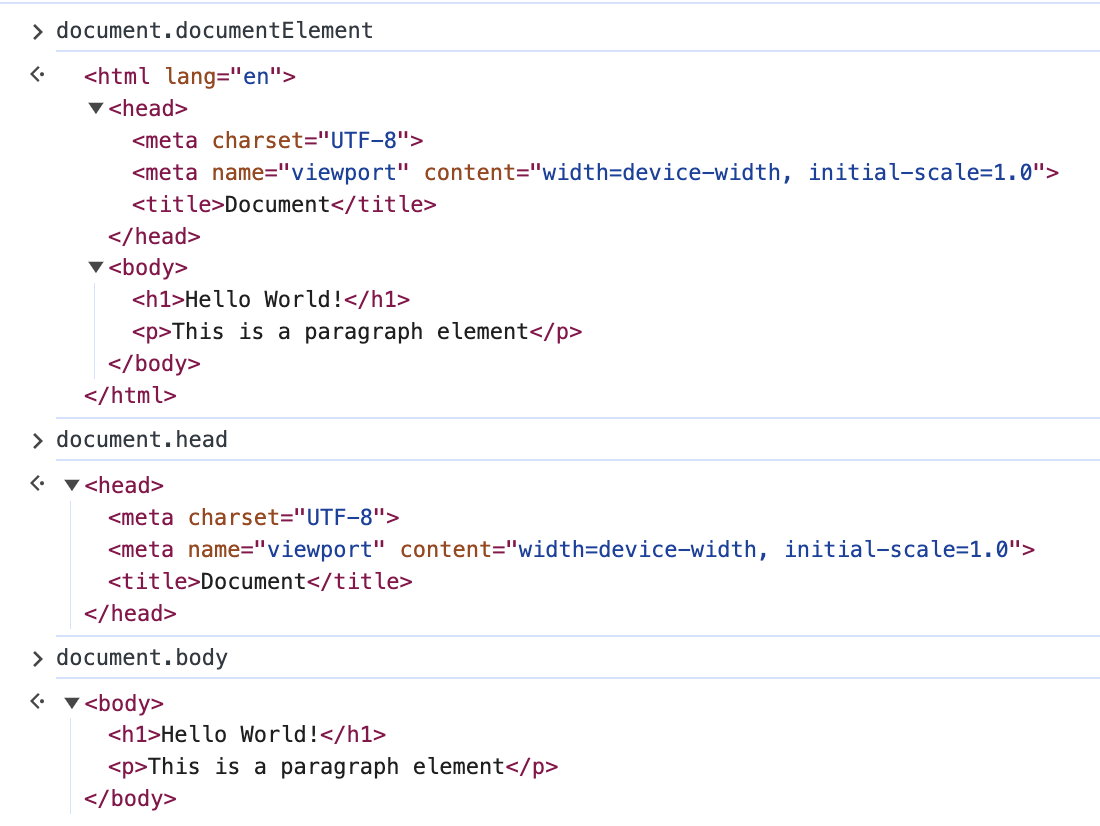
You can see the documentation of all the properties and methods of the document object here (note that it might be overwhelming at first).
Of course, you can do much more than just read your document data. I've written another article that shows you how to manipulate the DOM using JavaScript.
Summary
The DOM is a tree-like structure generated from the HTML file that we created and loaded into the browser.
The browser then exposes this DOM as a set of JavaScript objects that we can access, such as the window
and document
objects.
It’s an important piece of technology that allows you to program the web browser to do specific tasks or change your HTML content.
If you enjoyed this article and want to take your JavaScript skills to the next level, I recommend you check out my new book Beginning Modern JavaScript here.
The book is designed to be easy for beginners and accessible to anyone looking to learn JavaScript. It provides a step-by-step gentle guide that will help you understand how to use JavaScript to create a dynamic web application.
Here's my promise: You will actually feel like you understand what you're doing with JavaScript.
Until next time!