Today I'm gonna show you how you can center and align your content with CSS. Along the way, we'll look at various alignment techniques. So, let's get started! π₯
Table of Contents ->
- How to Use Flexbox to
- How to Use Grid to
- The Transform & position property
- The Margin Property
- Additional resources
- Conclusion
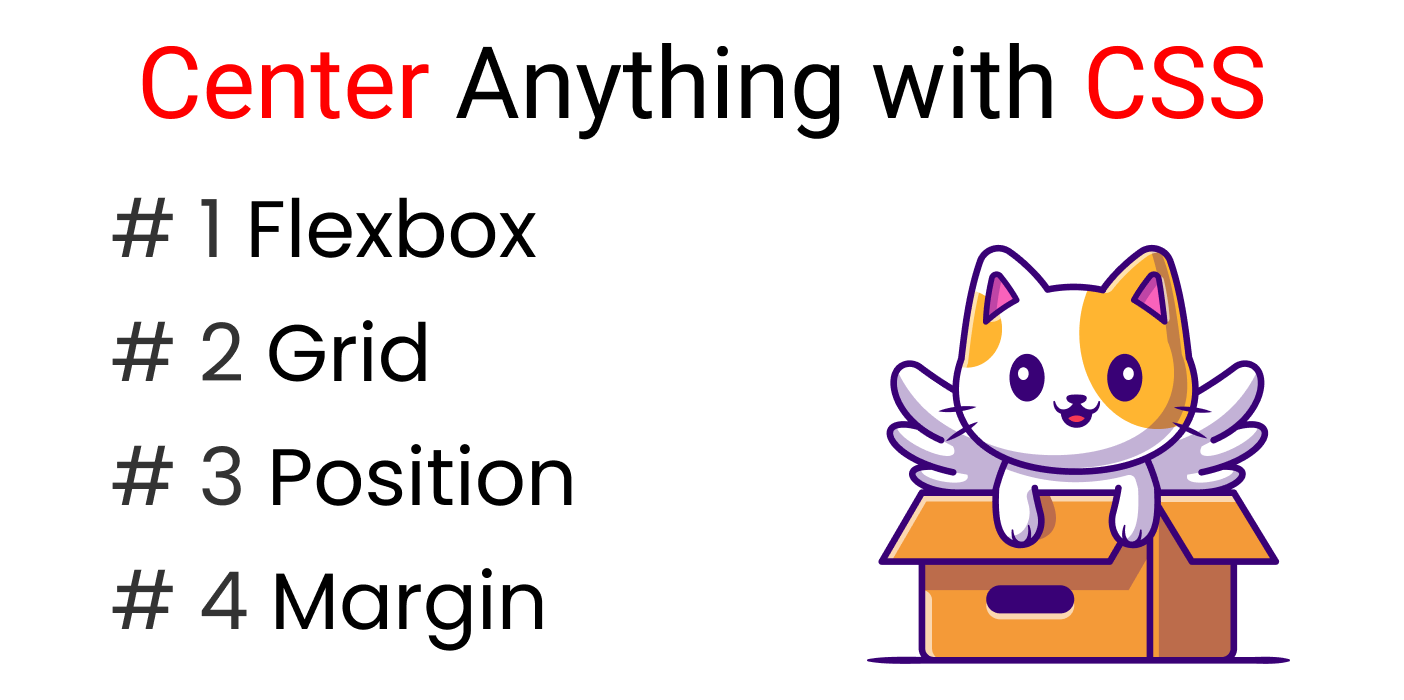
You can watch this tutorial on YouTube as well if you like:
But.... Wait A Minute!
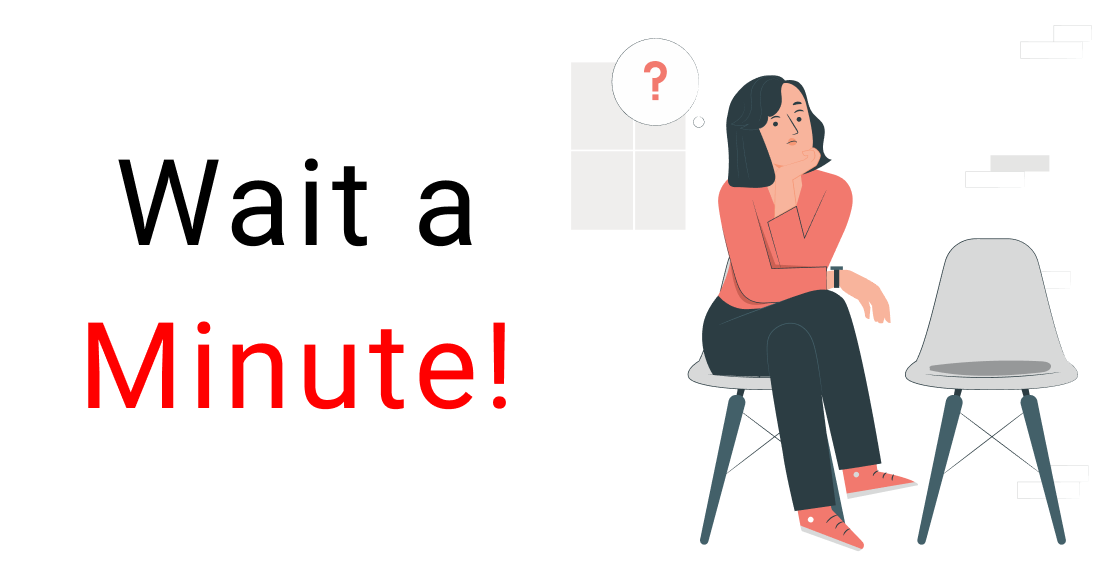
First of all, let's understand:
- Main Axis
- Cross Axis
What is the Main Axis in CSS?
You can also call it the:
- X-Axis
- Main Axis
- Horizontal Line
The line from left to right is the Main-Axis.

What is the Cross Axis in CSS?
You can also call it the:
- Y-Axis
- Cross Axis
- Vertical Line
The line from top to bottom is the Cross-Axis.
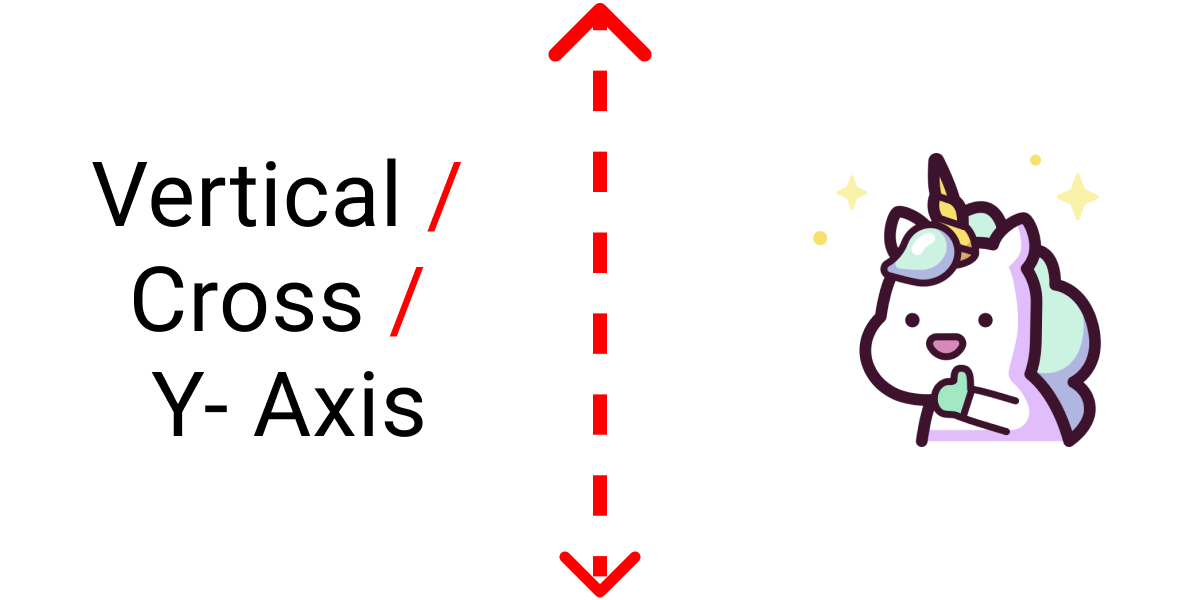
Project Setup
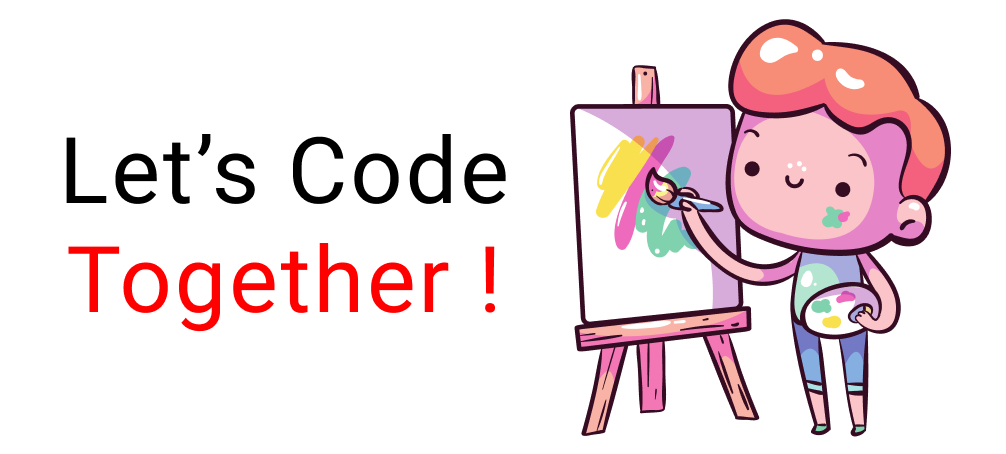
To experiment with all the properties and values, write the following code in your code editor.
HTML
Write this code inside the body tag:
<div class="container">
<div class="box-1"> </div>
</div>
CSS
Clear the default browser styles so that we can work more accurately:
*{
margin: 0px;
padding: 0px;
box-sizing: border-box;
}
Select the .container class and set it to 100vh. Otherwise, we can't see our result on the Vertical Axis:
.container{
height: 100vh;
}
Style the .box-1 class like this:
.box-1{
width: 120px;
height: 120px;
background-color: skyblue;
border: 2px solid black;
}
We're all set, now let's start coding!
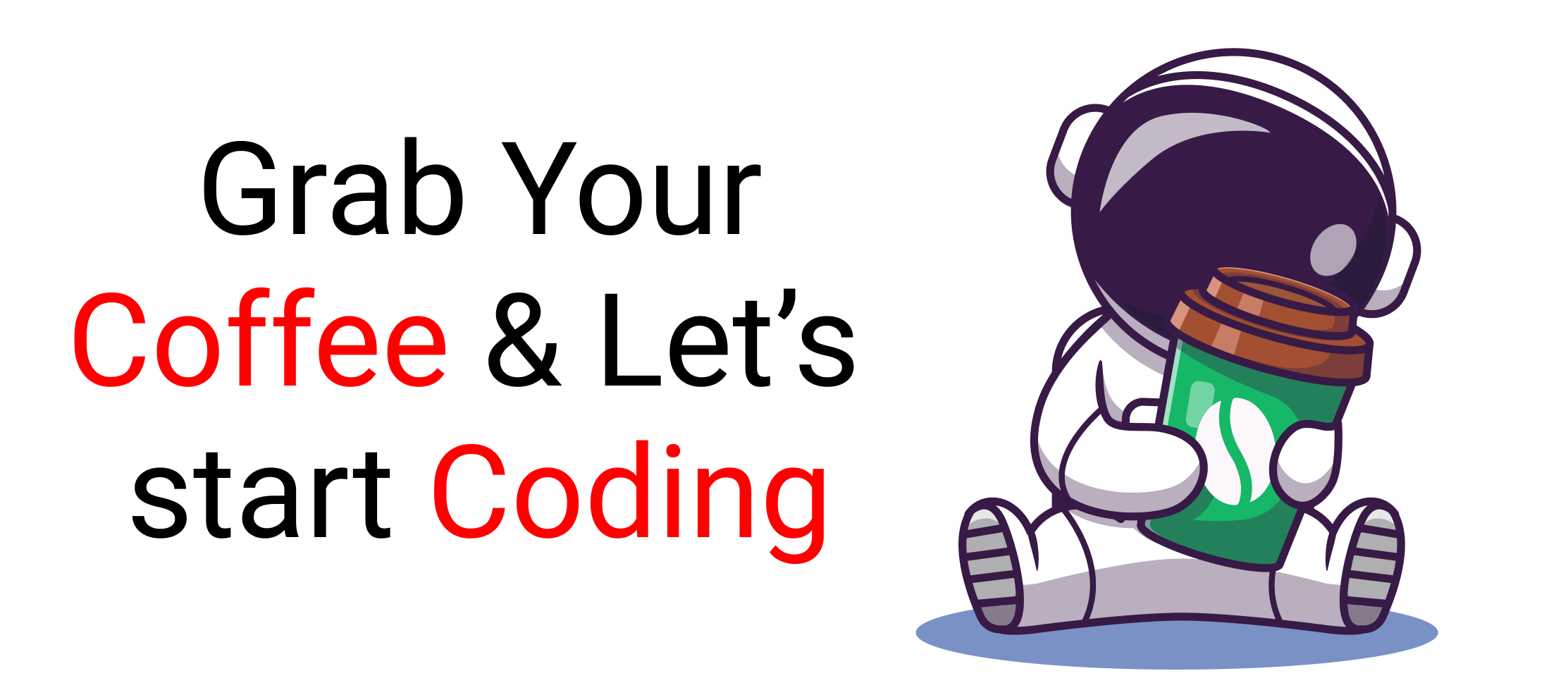
How to use Flexbox to center anything
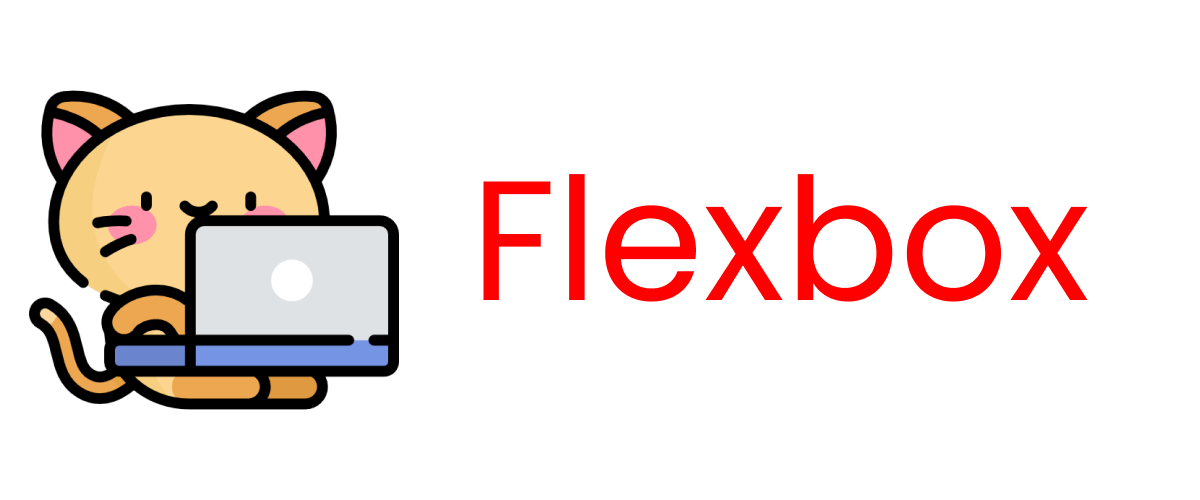
We can use Flexbox to align our content div
both along the X and Y Axis. To do that, we need to write the display: flex;
property inside the .container
class:
.container{
display: flex;
height: 100vh;
}
We'll experiment with these 2 properties:
justify-content
align-items
How to center anything horizontally using Flexbox
We can use the justify-content property to do this using these values π

To experiment with the values, write the following codeπ
.container{
display: flex;
height: 100vh;
/* Change values to π experiment*/
justify-content: center;
}
The result will look like thisπ

How to center anything vertically using Flexbox
We can use the align-items
property to do this using these values π
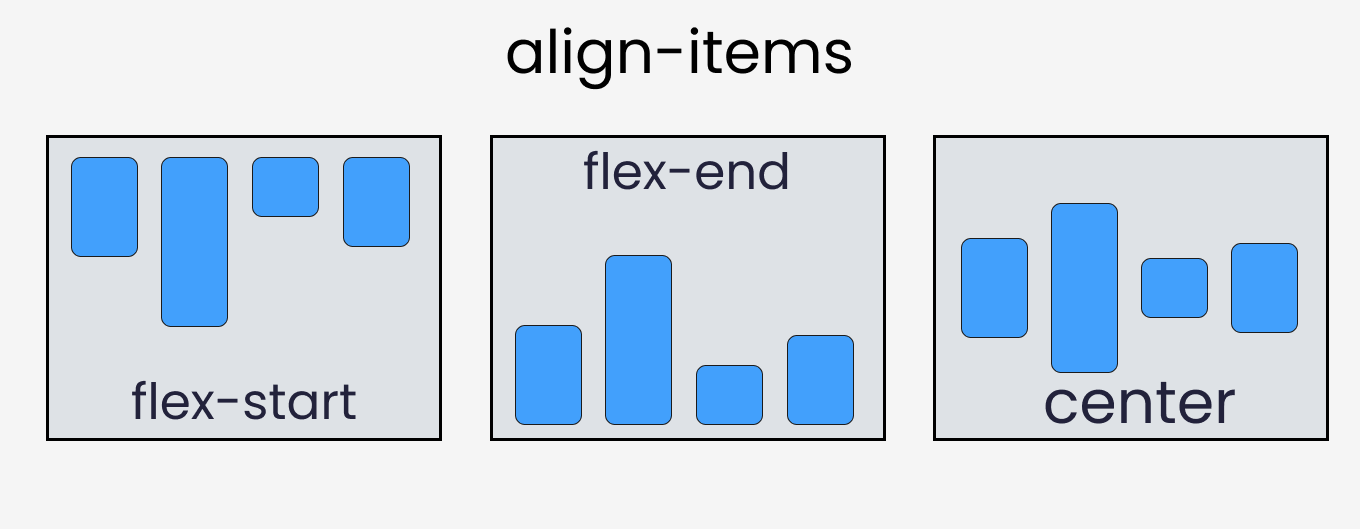
To experiment with the values, write the following codeπ
.container{
height: 100vh;
display: flex;
/* Change values π to experiment*/
align-items: center;
}
The result looks like thisπ

How to center a div horizontally & vertically using Flexbox
Here, we can combine both the justify-content
and align-items
properties to align a div both horizontally and vertically.
Write the following codesπ
.container{
height: 100vh;
display: flex;
/* Change values π to experiment*/
align-items: center;
justify-content: center;
}
The result looks like this π
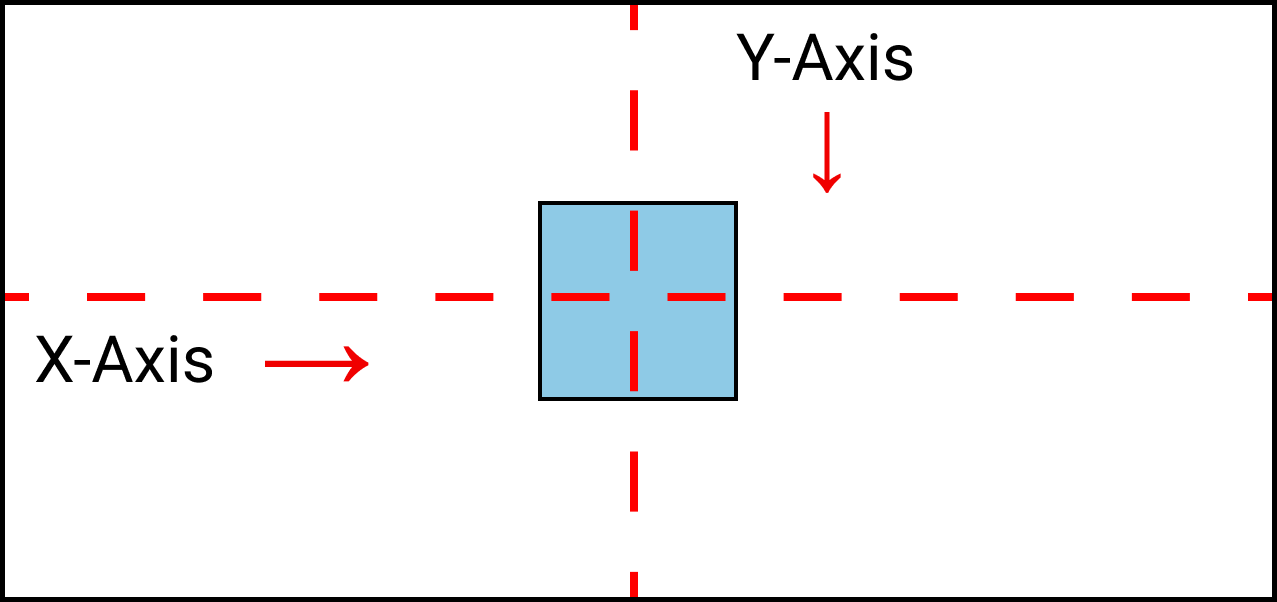
You can check out this cheatsheet to learn more about various Flexbox properties.
How to use CSS Grid to center anything
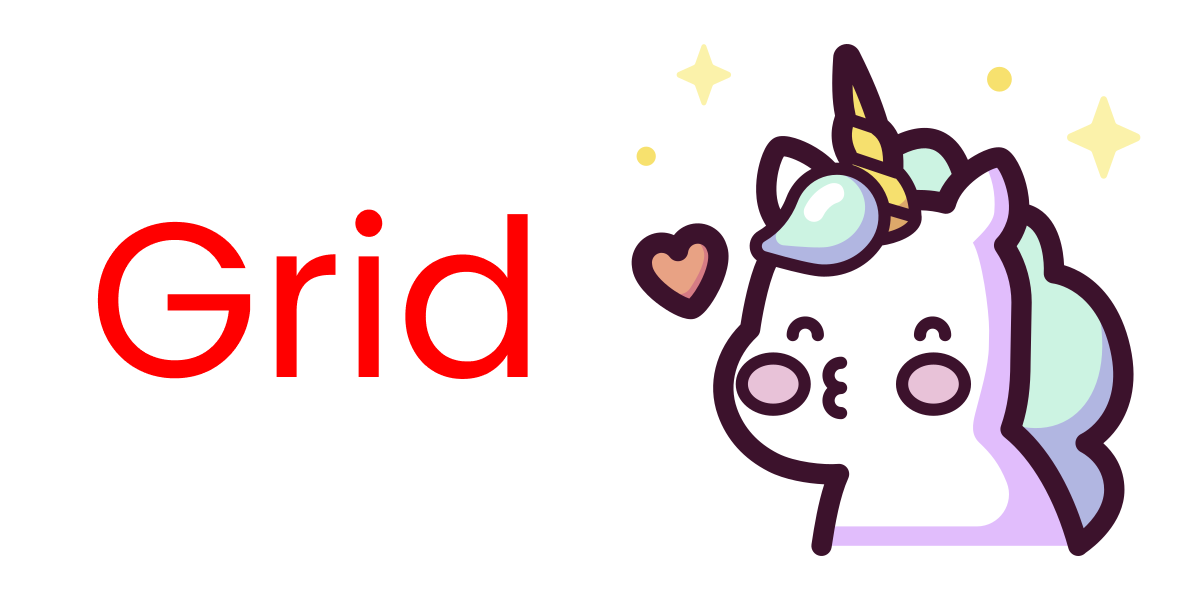
We can use grid to align our content div
both along the X and Y Axis. To do that, we need to write the display: grid;
property inside the .container
class:
.container{
display: grid;
height: 100vh;
}
We'll experiment with these 2 properties:
justify-content
align-content
Alternatively, you can use these 2 properties:
justify-items
align-items
How to center anything horizontally using CSS Grid
We can use the justify-content
property to do this using these values π

Write the following code π
.container{
height: 100vh;
display: grid;
/* Change values π to experiment*/
justify-content: center;
}
The result looks like thisπ

How to center anything vertically using CSS Grid
We can use the align-content
property to do this using these values π

Write the following code π
.container{
height: 100vh;
display: grid;
/* Change values π to experiment*/
align-content: center;
}
The result will look like thisπ

How to center a div horizontally & vertically using CSS Grid
Here, we can combine both the justify-content
and align-content
properties to align a div both horizontally and vertically.
Write the following code π
.container{
height: 100vh;
display: grid;
/* Change values π to experiment*/
align-content: center;
justify-content: center;
}
The result looks like thisπ

Alternative way
You can also use the justify-items
and align-items
properties to duplicate the same results:
.container{
height: 100vh;
display: grid;
/* Change values π to experiment*/
align-items: center;
justify-items: center;
}
The place-content Property in CSS Grid
This is the shorthand of 2 properties of CSS Grid->
justify-content
align-content
Follow along π
.container{
height: 100vh;
display: grid;
place-content: center;
}
We get the same result π

Check out this cheatsheet to find out the difference between various Grid properties.
Take a break!
So far so good β take a break.

How to use the CSS Position Property to center anything

This is a combination of these properties ->
position
top, left
transform, translate
Write the following code π
.container{
height: 100vh;
position: relative;
}
Along with this:
.box-1{
position: absolute;
width: 120px;
height: 120px;
background-color: skyblue;
border: 2px solid black;
}
First... Understand the center point of a div
By default, this is the center point of a div π
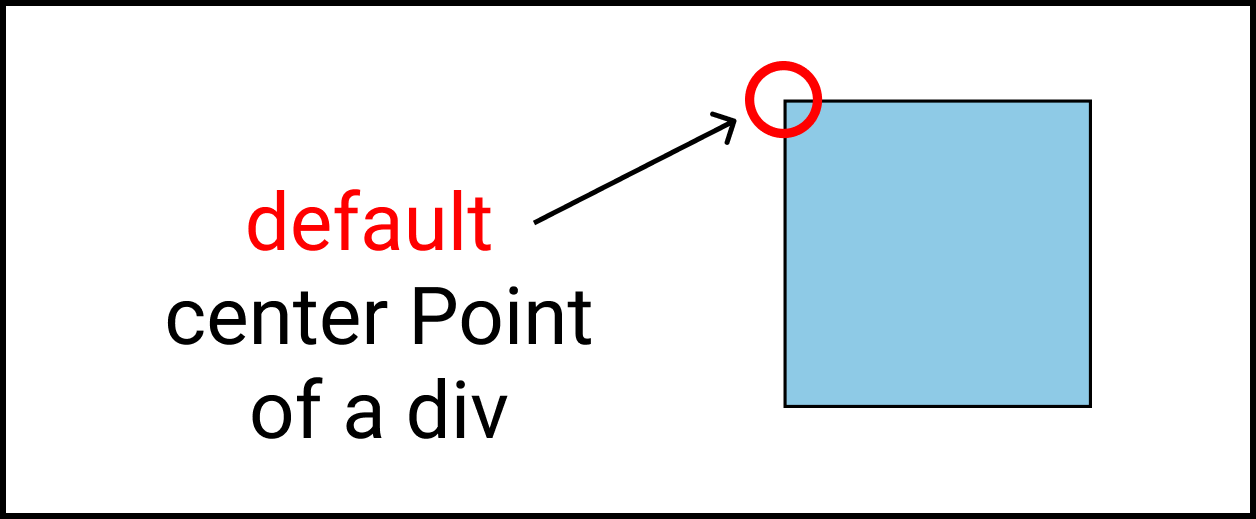
That's why we see this odd behavior π
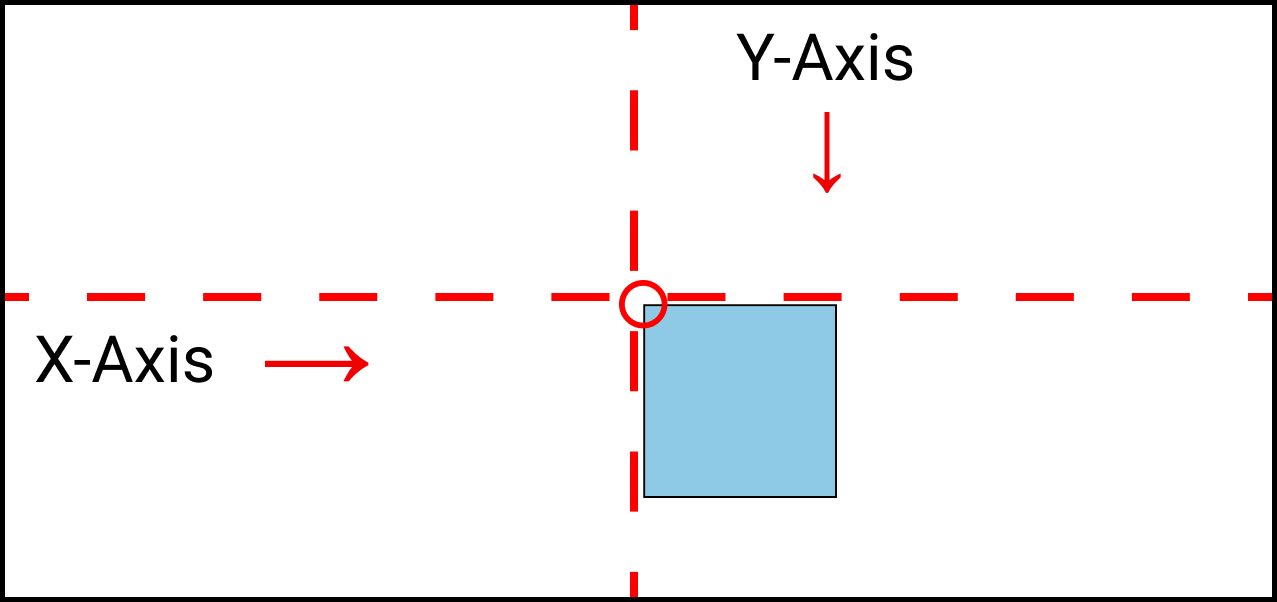
Notice that the box is not at the exact center in the image above. π
By writing this line π
transform: translate(-50%,-50%);
We fix the problem π
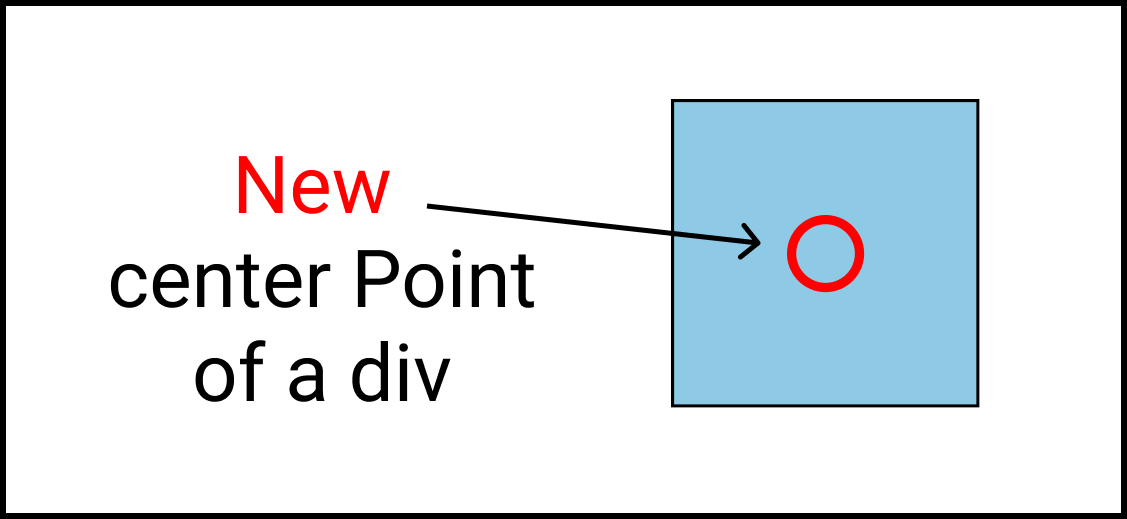
And we get this result π

What is the Translate property in CSS?
Translate is the shorthand of 3 properties ->
translateX
translateY
translateZ
How to center a div horizontally using CSS Position property
We're gonna use the left
property inside the`.box-
` class. Follow along π
.box-1{
/* other codes are here*/
left: 50%;
transform: translate(-50%);
}
And we get this result π

How to center a div vertically using CSS Position property
We're gonna use the top
property inside the`box-
` class. Follow along π
.box-1{
/* Other codes are here*/
top: 50%;
transform: translate(0,-50%);
}
And we get this result π

How to center a div horizontally & vertically using CSS position property
To achieve this result, we're gonna combine these properties together ->
top, left
transform, translate
Follow along π
.box-1{
/*Other codes are here */
top: 50%;
left: 50%;
transform: translate(-50%,-50%);
}
And we get this result π
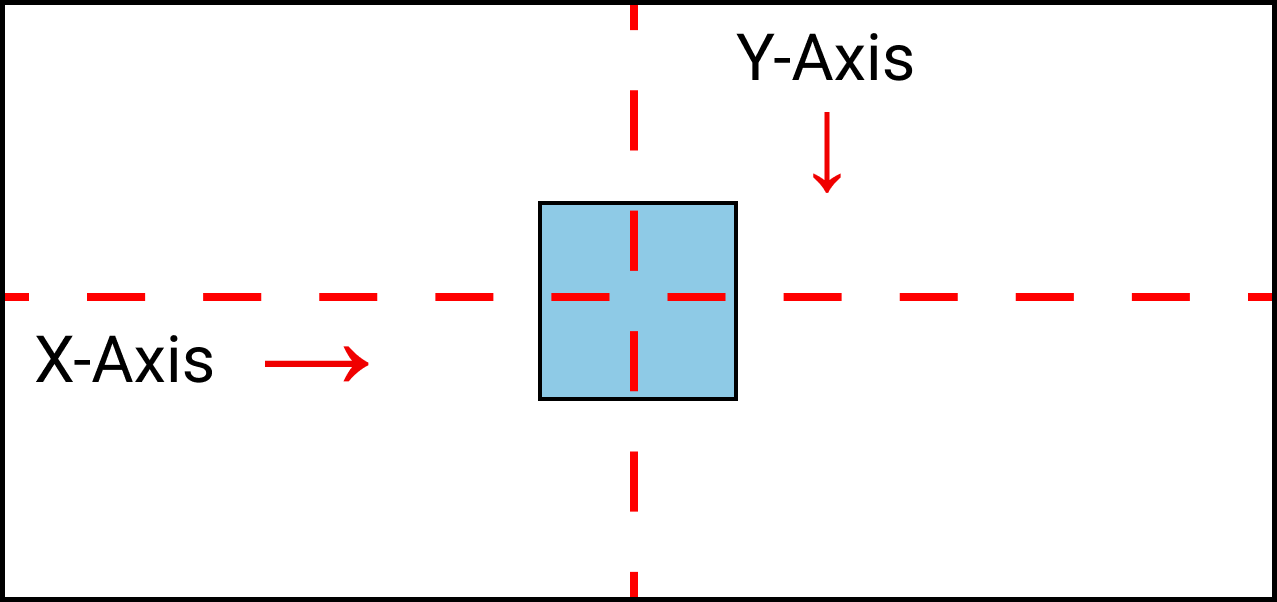
How to use the margin Property to center anything
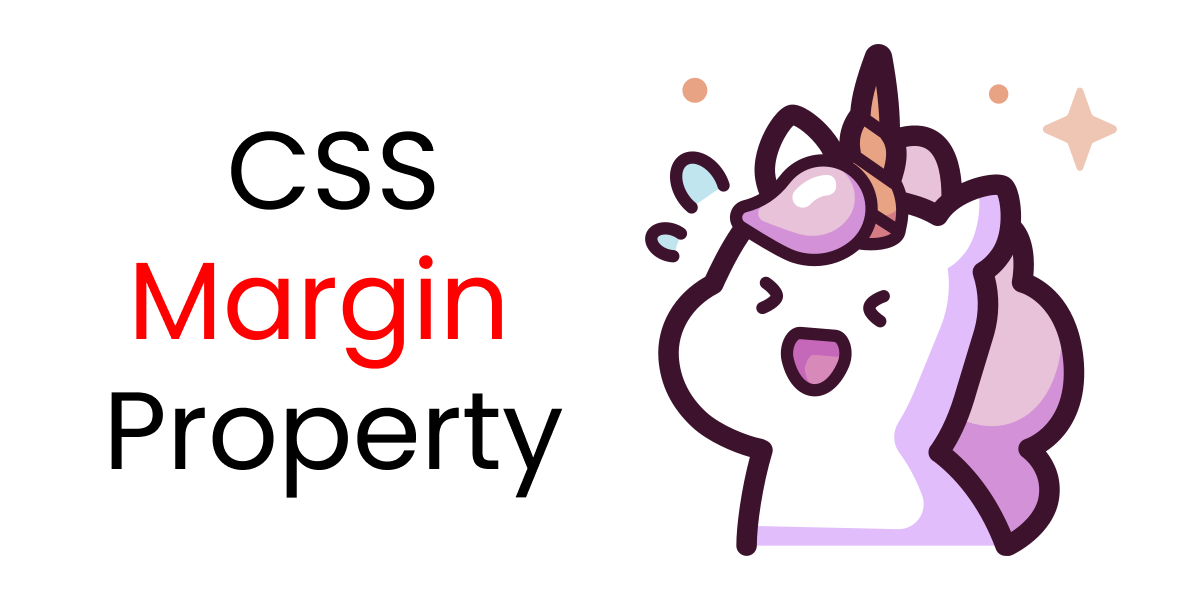
The margin property is the shorthand of 4 properties
margin-top
,margin-bottom
margin-left
,margin-right
Write this code to set it up π
.container{
height: 100vh;
display: flex;
}
.box-1{
width: 120px;
height: 120px;
background-color: skyblue;
border: 2px solid black;
}
How to center a div horizontally using CSS margin property
We're gonna use the margin
property inside the .box-1
class. Write the following code π
.box-1{
//Other codes are here
margin: 0px auto;
}
The result looks like thisπ

How to center a div vertically using CSS margin property
We're gonna use the margin
property inside the .box-1
class. Write the following code π
.box-1{
//Other codes are here
margin: auto 0px;
}
The result looks like this π
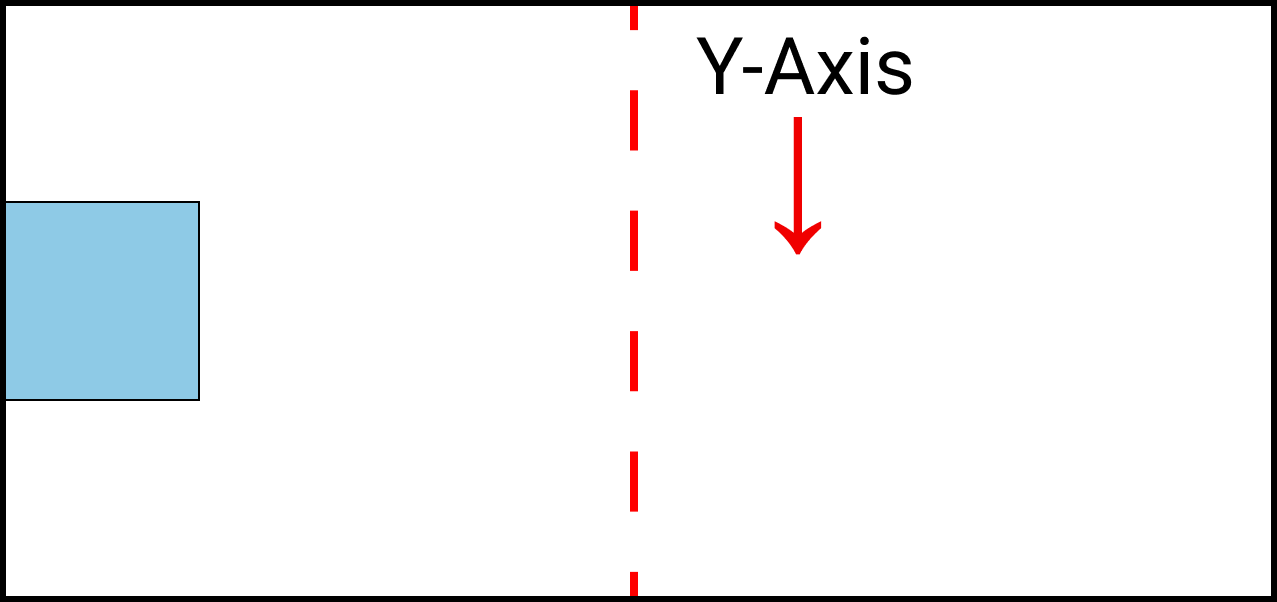
How to center a div horizontally & vertically using CSS margin property
We're gonna use the margin
property inside the`.box-
` class. Write the following code π
.box-1{
//Other codes are here
margin: auto auto;
}
The result looks like thisπ

Additional Resources
Credits
Conclusion
Now, you can confidently align or center your content using any of these four methods in CSS.
Here's your medal for reading until the end β€οΈ
Suggestions & Criticisms Are Highly Appreciated β€οΈ
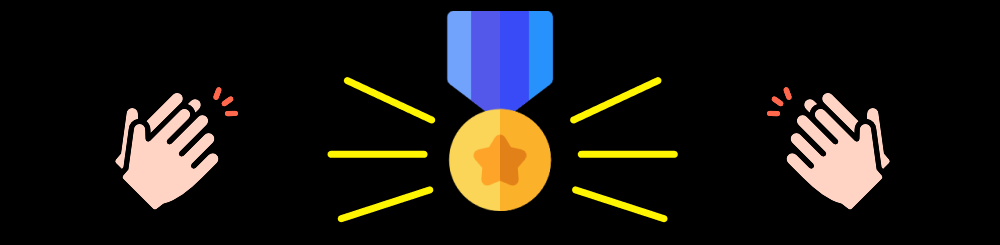
YouTube / Joy Shaheb
LinkedIn / Joy Shaheb
Twitter / JoyShaheb
Instagram / JoyShaheb