Have you ever wondered how modern web applications are built? How you can learn and master the technologies that you can use to build your own full stack projects from scratch?
In this handbook, I'm going to introduce you to the MERN stack, a widely-used technology stack embraced by many leading companies. I'll guide you through 7 essential steps to start learning these technologies on your own.
By the time you finish reading, you'll have a solid understanding of what the MERN stack entails, its component technologies, and various resources for learning. You'll also have 10 project ideas that you can develop and showcase in your portfolio.
Table of Contents
- What is the MERN stack?
- The MERN Stack Roadmap
- Top Resources to learn the MERN Stack
- 10 project ideas you can try today
- Wrapping up the MERN stack journey
What is the MERN stack?
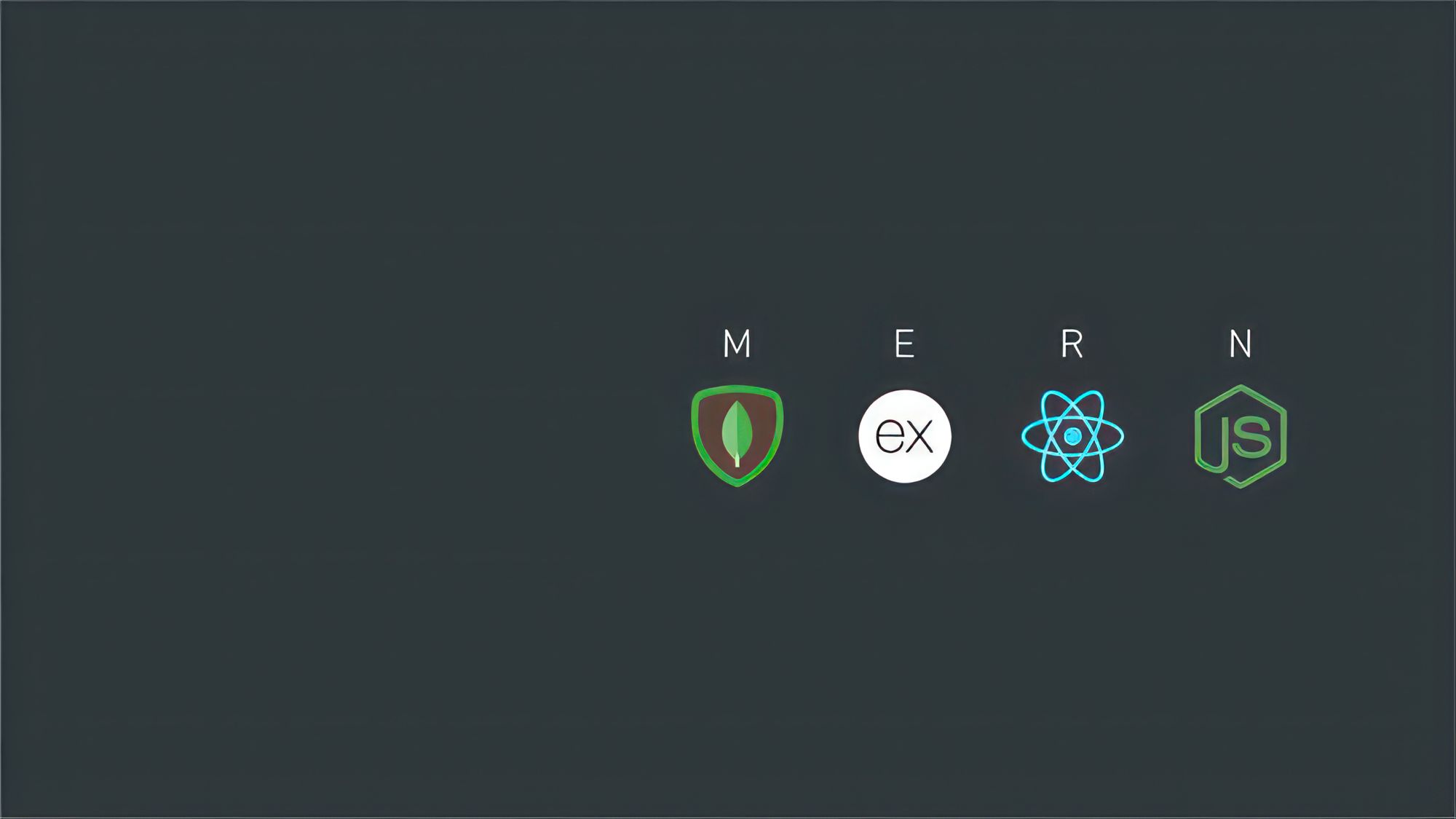
The MERN stack, comprising MongoDB, Express.js, React, and Node.js, is a cohesive set of technologies used for building efficient and scalable web applications.
Its popularity stems from the seamless integration of each component: MongoDB's flexible data handling, Express.js's efficient server-side networking, React's dynamic user interfaces, and Node.js's powerful back-end runtime environment.
For beginners, the MERN stack is a smart choice because it uses JavaScript across all layers, simplifying the learning curve. This uniformity, coupled with a strong community and ample learning resources, makes it an accessible and practical toolkit for anyone looking to dive into full-stack development.
The MERN stack is also heavily utilized in the industry, favored by startups and large enterprises alike for its efficiency and the robust, modern web applications it can produce. This industry adoption not only validates its effectiveness but also opens up numerous career opportunities for those skilled in these technologies.
Let's look at a brief overview of what each part of the MERN Stack looks like:
Frontend (React)
The frontend of a website is like the dining area of a restaurant. It's everything you see and interact with directly on a website – the layout, design, buttons, and text.
Example: When you visit a website and see a beautiful homepage, interact with menus, or fill out forms, you're experiencing the frontend.
Backend (Node.js)
The backend is like the kitchen in a restaurant. It's where all the behind-the-scenes work happens. It includes the server, applications, and databases that work together to process the information you see on the frontend.
Example: When you order food (submit a form on the website), the kitchen (backend) processes the order (the data) and prepares your meal (the information or service you requested).
Database (MongoDB)
A database in web development is similar to a restaurant’s pantry or storage where all the ingredients (data) are kept. It stores and manages all the information needed by the website, like user profiles, content, and other data.
Example: In an online store, the database stores product information, prices, user reviews, and customer details.
REST APIs (Express)
REST APIs are like the waiters in a restaurant. They are the messengers or go-betweens for the frontend and backend. They take requests (like orders) from the frontend (customer), fetch or update data in the backend (kitchen), and then return responses (prepared orders).
The terms POST, PUT, DELETE, and GET are types of requests used in REST APIs:
- POST: Used to create new data. Like placing a new order in the restaurant.
- PUT: Used to update existing data. Similar to changing an order you've already placed.
- DELETE: Used to remove data. Like cancelling an order.
- GET: Used to retrieve data. Comparable to asking about the menu or checking the status of your order.
The MERN Stack Roadmap
STEP 1: Learn the right amount of HTML, JavaScript, and CSS
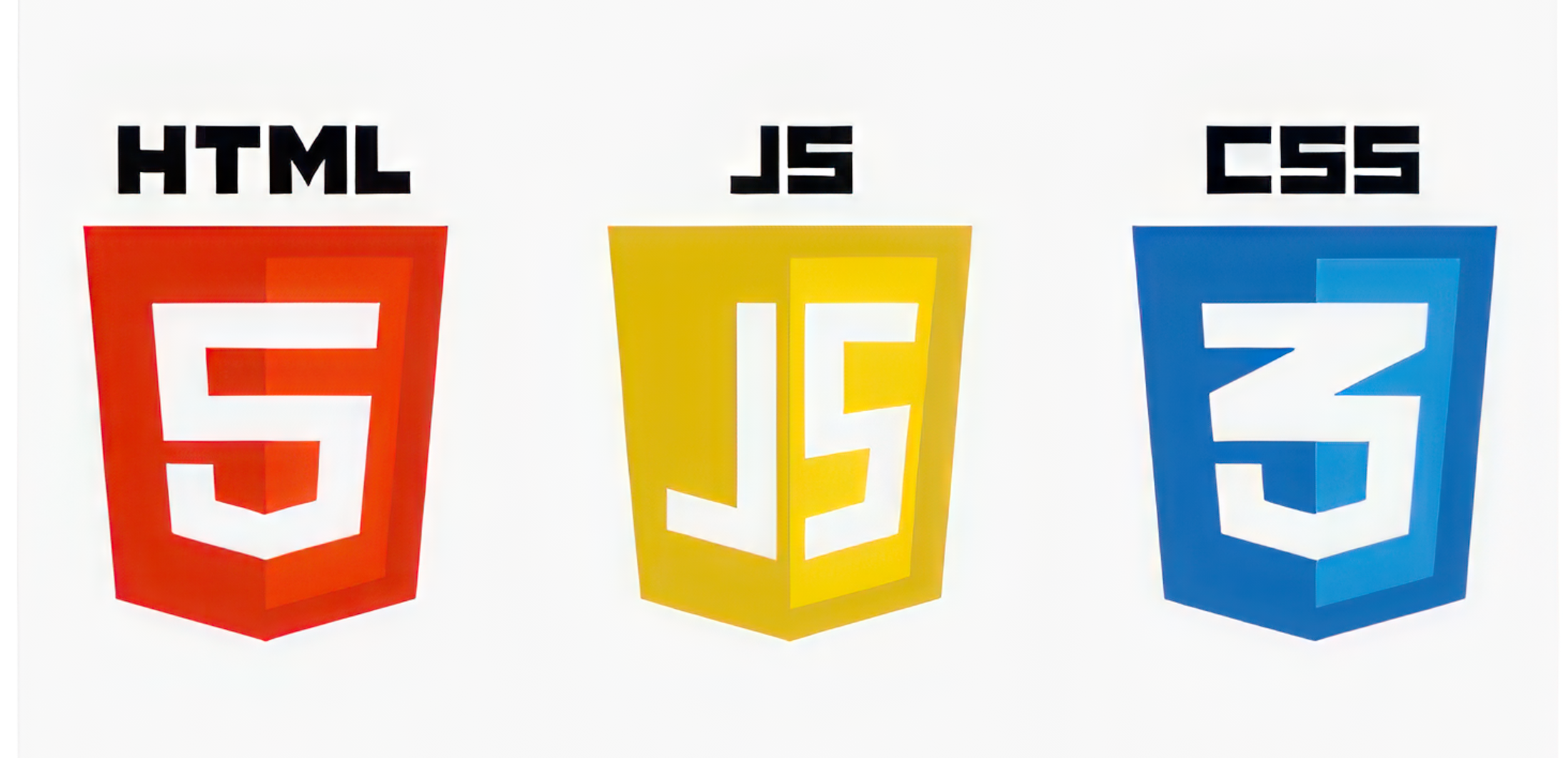
The MERN stack uses JavaScript heavily, so its a natural first step to learn. In this section you will look at the main things you will use day-to-day when creating full-stack MERN apps.
Understanding JavaScript is like knowing the right amount of ingredients needed for a recipe. You don't need to master everything at once, just the essential ingredients that make your particular dish (or your web project) come to life.
Variables
Variables in JavaScript are like labelled jars in your kitchen. You can store things in them (like numbers, text) and use these jars later in your cooking (or coding).
Example: A variable storing the user's name, so you can use it later to say "Hello, [Name]!"
Functions
Functions are like recipes in a cookbook. They are sets of instructions that perform a specific task. You can reuse these recipes whenever you need to perform that task again.
Example: A function that calculates the total price of items in a shopping cart.
Objects & Arrays
Objects are like information cards holding details about something (like a contact card), and arrays are like lists.
Example of an Object: A card holding a user's information (name, age, email).
Example of an Array: A list of all the user's favorite book titles.
If/else Statements, Switch Statements
These are like decision-making processes. If/else statements are like choosing what to wear based on the weather, and switch statements are like a more complex decision, like choosing what to cook based on multiple ingredients you have.
Example: If it's raining (if), take an umbrella (else), take sunglasses.
Callbacks/Promises/Async Await
These are ways to handle tasks that take some time, like ordering food and waiting for it. Callbacks are like calling a friend to do something when they’re free. Promises are like your friend promising to do it. Async-await is like making a plan to do tasks one after another in an organized way.
Example: Ordering a coffee (a task) and waiting to get it before leaving the cafe (ensuring order of actions).
ECMAScript (Template Strings, Destructuring Assignment, Spread Operator, Default Parameters, and so on)
These are advanced tools and shortcuts in JavaScript to make coding easier and cleaner. It's like having a food processor in your kitchen that makes chopping and mixing faster and more efficient.
Example: Automatically creating a welcome message like "Hello, [Name]!" without manually joining words and variables.
TypeScript
TypeScript is like JavaScript but with more rules for organizing your code (like a more detailed recipe book). It helps in managing larger projects by adding types to your code, making sure you don’t mix incompatible ingredients. This guide teaches you TypeScript basics.
Example: Specifying that a function should only take a number as an input, not text or anything else.
STEP 2: Get Familiar with React

Once you've got a feel for JavaScript, its time to venture into the wonderful world of frontend development.
React.js is a popular JavaScript library used for building user interfaces, particularly known for its efficiency in rendering dynamic, interactive web pages. It enables developers to create large web applications that can change data, without reloading the page, making for a smoother user experience.
Below is a list of common things you want to know when working with React.
Components
Think of components as individual LEGO blocks in a large LEGO model. Each block is a small, reusable piece that you can use to build different parts of your web application.
Example: A 'button' component in a website that can be used in many places, like for submitting a form or closing a pop-up.
JSX (JavaScript XML)
JSX lets you write your website's design code (like HTML) inside your JavaScript code. It's like writing the recipe and the cooking instructions in one place for easier reference.
Example: Writing a piece of JSX code that includes both JavaScript and HTML-like tags to create a user login form.
Props (Properties)
Props are like instructions or settings you pass to your LEGO blocks (components) to tell them what they should look like or do.
Example: Passing a 'color' prop to a 'button' component to make it red or blue depending on the situation.
State
State is like a personal notebook for each component, where it keeps track of its own information, like whether a button is clicked or not. Here's a course all about state management in React.
Example: A 'like' button keeping track of whether it has been clicked (liked) or not.
Hooks
Hooks are special tools in React that let you add features like state to your components without needing to use complex code structures.
Example: Using the useState hook to keep track of a counter in a component.
Event Handling
This is how you tell a component to do something when a user interacts with it, like clicking a button or entering text in a form.
Example: Setting up an event handler so that when a user clicks a 'submit' button, it sends their information to the server.
Conditional Rendering
This is like having a magic painting that can change its picture based on certain conditions. In React, you can show different components based on different conditions.
Example: Showing a 'login' button if a user is not logged in, and a 'logout' button if they are.
Lists and Keys
Keys are like name tags for items in a list. They help React keep track of which items are new, changed, or removed.
Example: Displaying a list of messages in a chat app, where each message has a unique key.
Context API
The Context API a way to share information (like a theme setting or user data) across many components without passing the information through each level manually.
Example: Using Context API to share the current logged-in user's information across different components in a web app.
Fragment
Fragments let you group several components or elements together without adding extra layers to the website. It's like putting multiple LEGO pieces on a baseplate without the baseplate being part of the final model.
Example: Grouping a list of items together in a menu without adding extra wrappers or divs around them.
STEP 3: Understand REST API's and how a backend server works using Express/Node
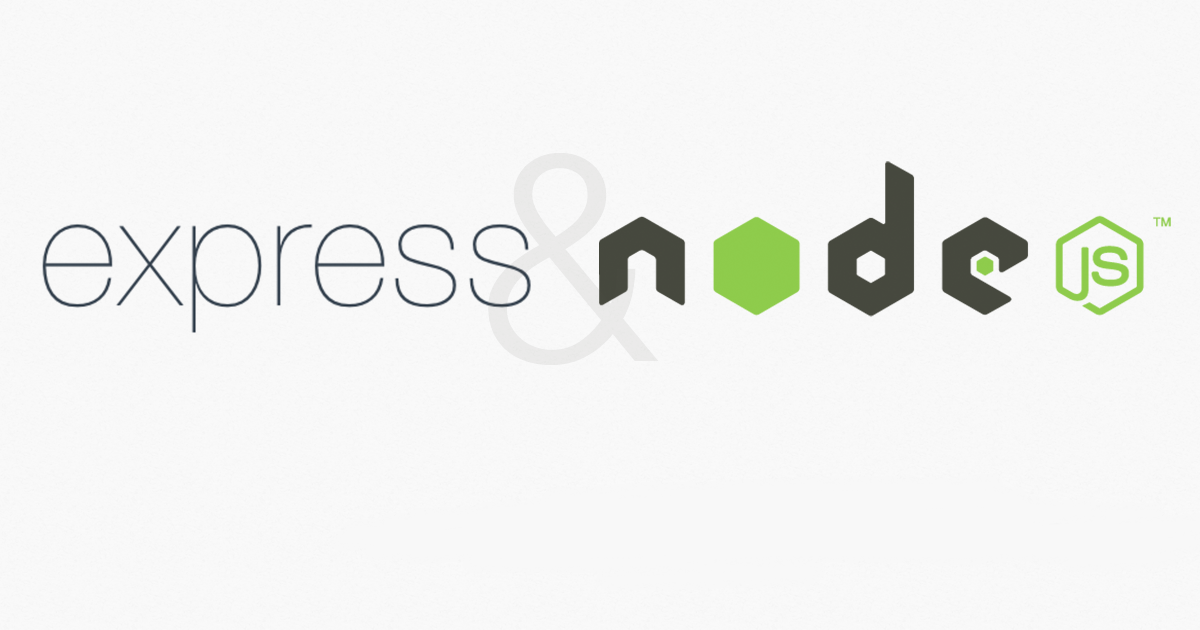
Every UI needs a way to store and retrieve the data it needs to make the frontend work. This is where our backend comes in.
In the MERN stack, the backend is composed of 3 main bits: Express, a Node.js server, and a database. We'll cover the database shortly, for now we'll focus on the Express/Node pieces of the MERN stack, as they're closely related.
Express.js is a lightweight, flexible framework for Node.js, designed to build web applications and REST APIs with ease and efficiency. Node.js is a powerful JavaScript runtime that allows for the development of scalable server-side applications, making the duo a popular choice for backend development.
Node.js: Foundation for Building Web Applications
Think of Node.js as the foundation for constructing a modern building. It's a platform that lets you build web applications, similar to how you would use a set of basic tools and materials to start building a house. You might hear this being to referred to as the "backend".
Example: Building a chat application where multiple users can send messages in real-time.
Express: Streamlining REST API Development
Express is a helper tool for Node.js. It's like having a pre-built kit that makes building certain parts of your house easier and faster, providing templates and shortcuts so you don't have to start from scratch.
Example: Using Express to quickly set up routes for a website, like a route to a contact page or a product catalog.
Modules and Packages: Ready-Made Components
In Node.js, modules are like pre-made components or sections of a house (like a bathroom unit or a kitchen set) that you can simply choose and add to your building project.
Example: Adding a 'date-time' module to display current times and dates on your web application.
Node Package Manager (NPM): The Tool and Material Warehouse
NPM acts like a vast warehouse where you can find all sorts of tools and materials (modules) you might need. It's a central place to get additional resources for building your web applications.
Example: Installing 'body-parser' from npm to handle JSON data in your web application.
Routing: Directing Web Traffic
Routing in Express is like setting up roads and paths in a housing complex. It's about directing the flow of traffic (data and user requests) to different parts of your web application.
Example: Creating routes in an online store, like /products
for the product list and /products/:id
for individual product details.
Middleware: Additional Functional Layers
Middleware in Express can be seen as extra layers or services in your building, like security, plumbing, or electrical systems. They add specific functionalities to your web application.
Example: Adding 'cookie-parser' middleware to handle cookies in your web application.
Request and Response: Communication Channels
Requests and responses in Express are like sending and receiving mail or messages. They are the way your web application communicates with users, sending them data or receiving their inputs.
Example: Your application receiving a user's login request (request) and then sending back a confirmation message (response).
Environment Variables: Secure Storage Spaces
Think of environment variables as secure storage spaces or safes in your building. They're used to store sensitive information like passwords or personal settings, keeping them secure and separate from the main construction.
Example: Storing the database connection string in an environment variable to keep it secure.
Security: Building Safeguards
In the context of web applications, security is about building safeguards into your project. It's like installing locks, security systems, and fire safety measures in a building to protect it from various threats.
Example: Implementing HTTPS to secure data transmission and using JWT (JSON Web Tokens) for user authentication to protect user data.
STEP 4: Storing data with MongoDB and Mongoose

MongoDB is a NoSQL database that offers high flexibility and scalability for storing and managing data, making it ideal for handling large volumes and diverse types of data.
Mongoose is an Object Data Modeling (ODM) library for MongoDB, providing a straightforward schema-based solution to model application data, enhancing database interaction with useful features and validation.
MongoDB: A NoSQL Database
MongoDB is a type of database that stores data in a flexible, JSON-like format. This makes it different from traditional databases, which use tables and rows. It's great for handling large volumes of data and is very scalable.
Example: Using MongoDB to store user profiles where each profile can have different fields.
Collections and Documents
In MongoDB, data is stored in 'collections', which are similar to tables in a relational database. Inside these collections, data is stored in 'documents'. Think of a document as a single record in your collection, like an entry in a diary or a contact in an address book.
Example: A 'users' collection with documents each representing a user with details like name and email.
Mongoose: A MongoDB Object Modeling Tool
Mongoose is a library for Node.js that makes it easier to interact with MongoDB. It provides a straightforward, schema-based solution to model your application data. It's like having a personal assistant to help manage the communication between your application and MongoDB.
Example: Using Mongoose to easily add, retrieve, and manage user data in a MongoDB database.
Schemas
In Mongoose, a schema is a structure that defines the format of the data to be stored in MongoDB (like defining fields and data types). Think of it as a blueprint for how your data should look.
Example: Creating a Mongoose schema for a blog post with fields like title, author, and content.
Models
A model in Mongoose acts as a constructor, compiled from the Schema definitions. It represents documents in a MongoDB database. Models are responsible for creating and reading documents from the underlying MongoDB database.
Example: Defining a 'User' model based on a user schema to interact with the 'users' collection in the database.
CRUD Operations
CRUD stands for Create, Read, Update, Delete. These are the basic operations you can perform on the database. Mongoose provides easy methods to execute these operations on your data.
Example: Using Mongoose methods to add new users, find users by name, update user information, or delete users.
Connecting to MongoDB
You can use Mongoose to connect your Node.js application to a MongoDB database. This is like setting up a phone line between your application and the database so they can talk to each other.
Example: Writing a Mongoose connect function to link your Node.js application to a MongoDB database hosted on Atlas.
Querying Data
Mongoose allows you to query your MongoDB database. This means you can search for specific data, filter your data based on certain criteria, and more.
Example: Using a Mongoose query to find all blog posts written by a specific author.
Data Validation
Mongoose provides built-in validation. This is a way to make sure the data being saved to your database is in the right format, like checking if an email address looks like an email address.
Example: Defining a schema in Mongoose where the email field must match the format of an email address.
Middleware (Pre and Post Hooks)
Mongoose middleware are functions which can be executed automatically before or after certain operations, like saving a document. They're useful for complex logic like hashing passwords before saving them to the database.
Example: Using a pre-save middleware in Mongoose to hash user passwords before saving them to the database.
Indexes
Indexes in MongoDB are used to improve the performance of searches. They are similar to indexes in a book, helping the database find data faster.
Example: Creating an index on the 'email' field in a user collection to speed up the search for users by email.
Aggregation
Aggregation in MongoDB is a powerful way to process data and get computed results. It's like having a sophisticated calculator to perform complex operations on your data, such as summing up values or averaging them.
Example: Using MongoDB's aggregation framework to calculate the average number of comments on blog posts.
STEP 5: Writing Tests
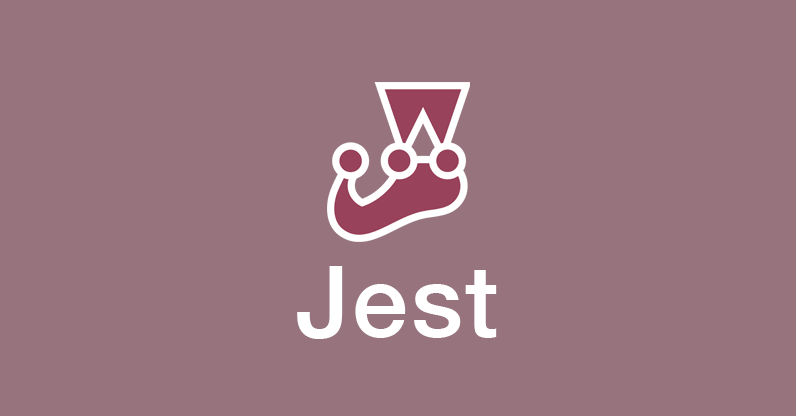
Testing in software development is like a safety check to ensure everything in your application works as expected. It's a crucial step in the development process where you look for bugs or issues before your users do. Think of it like proofreading an essay or checking a car before a road trip – it helps catch and fix problems early.
There are different types of testing, each serving a unique purpose:
Unit Testing
This is the most basic form of testing. Here, you test individual parts of your code (like functions or components) in isolation. It's like checking each light bulb in a string of Christmas lights.
In the MERN stack, tools like Jest or Mocha are commonly used for this. They let you write small tests to check if a specific part of your application behaves as expected.
Integration Testing
This type of testing checks how different parts of your application work together. It's like making sure all the light bulbs light up when connected and the string is plugged in.
For the MERN stack, you might still use Jest or Mocha, combined with other tools like Chai for assertions, to ensure that different components or services in your application interact correctly.
End-to-End (E2E) Testing
Here, you test your application's workflow from start to finish. It's like checking if the entire Christmas tree lights up and twinkles as expected.
For a MERN stack application, Cypress or Selenium are popular choices. They simulate real user scenarios, ensuring the entire application, from the front end in React to the back end with Express and Node.js, functions smoothly together.
Performance Testing
This checks if your application can handle stress, like heavy traffic or data processing. It's akin to ensuring the Christmas tree lights don't blow a fuse when they're all on. Tools like Loader.io or Apache JMeter can be used here.
Each type of testing serves to ensure a different aspect of your application is working correctly, and together, they contribute to building a robust, reliable, and user-friendly application.
By employing these tests in the MERN stack, you not only catch bugs early but also maintain a high standard of quality for your application.
STEP 6: Using Git
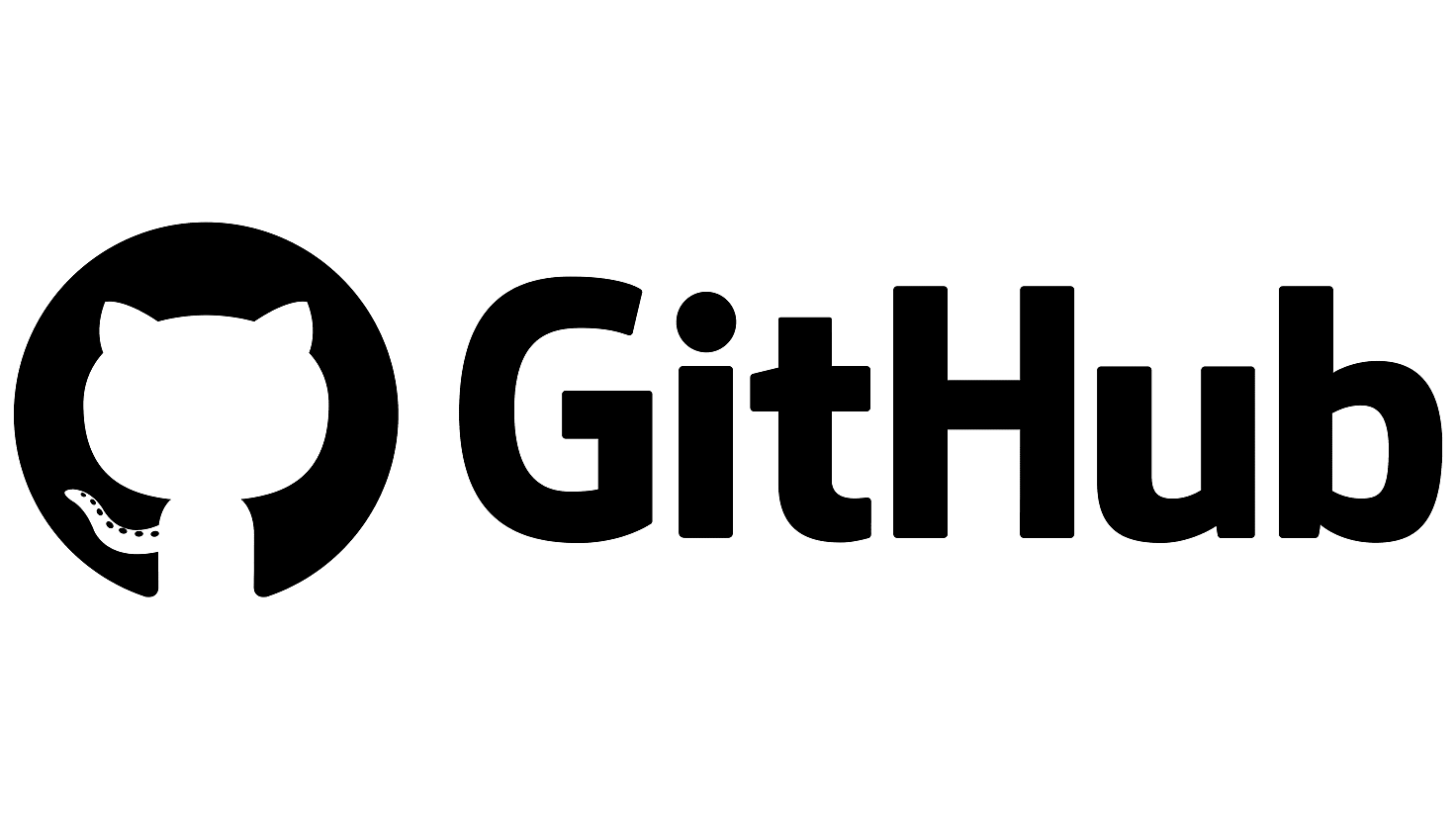
Git is a version control system, a tool that tracks changes in your code over time. Think of it like a detailed diary for your coding project. Every time you make changes to your code, Git keeps a record of what was changed, when, and by whom. This becomes incredibly useful when you’re working on complex projects, like those involving the MERN stack.
Why is Git so important when building with the MERN stack, or any software for that matter?
Collaboration
Git is like a team playbook. It allows multiple developers to work on the same project without stepping on each other's toes.
Everyone can work on different features or parts of the application simultaneously (like MongoDB database schemas, React components, or Express.js routes). Git helps manage these contributions, ensuring changes can be merged smoothly into the main project.
Tracking Changes
Imagine you've made changes to your React component, and suddenly things aren't working as they used to. Git allows you to go back in time and see what changes were made and by whom.
This historical data is invaluable for understanding how your project evolved and for fixing issues without having to start from scratch.
Branching and Merging
Git’s branching feature lets you diverge from the main line of development and experiment with new features or ideas in a controlled way.
You can create a branch, make your changes, and then merge those changes back into the main project when they're ready. This ensures the main project (often referred to as the 'master' or 'main' branch) remains stable.
Backup and Restore
With Git, your project's entire history is stored in the repository. If anything goes wrong, you can roll back to a previous state. It’s like having a fail-safe backup system.
Documentation
Commit messages in Git provide a narrative for your project. They allow you to document what changes were made and why, which is extremely helpful for both your future self and other developers who might work on the project.
When building applications with the MERN stack, Git offers a safety net and a collaborative workspace. It keeps your project organized, tracks every change, and allows multiple developers to work together more efficiently.
Using Git is essential for managing complex development projects in today's software development world.
STEP 7: Deployments

Code deployment is the process of taking code written by developers and making it operational on a live environment where users can interact with it.
In the context of the MERN stack, which involves technologies like MongoDB, Express.js, React, and Node.js, deployment is the final step in the journey of bringing a full-stack application to life.
Imagine you've built a house (your web application). Deployment is like moving it from the construction site (development environment) to its actual location where people can live in it (production environment). This process involves several key steps to ensure that everything works as expected when users access your app.
Preparing for Deployment
Before deploying, you need to prepare your application. This involves ensuring your code is complete and tested, dependencies are properly managed, and your app is configured for the production environment.
For MERN stack applications, this might mean setting up environment variables, configuring your database (MongoDB) connection for production, and optimizing your React front-end for performance.
Hosting and Servers
Choosing where to host your application is crucial. For MERN stack apps, you can use cloud-based hosting services like AWS, Heroku, or DigitalOcean. These platforms offer services to host both your Node.js backend and MongoDB database, and they often provide additional features like scaling, monitoring, and security.
Continuous Integration/Continuous Deployment (CI/CD)
CI/CD is a methodology that automates the deployment process. Whenever you make changes to your codebase (like fixing a bug or adding a new feature), CI/CD tools automatically test your code and deploy it if the tests pass. This ensures that your application is always up-to-date with the latest changes.
Tools like Jenkins, Travis CI, or GitHub Actions are commonly used for this purpose.
Monitoring and Maintenance
After deployment, it’s important to monitor your application for any issues and perform regular maintenance. This could involve checking server logs, updating dependencies, or rolling out fixes for any bugs that might arise.
Rollbacks
A good deployment strategy also includes plans for rollbacks. If something goes wrong after deployment, you need to be able to revert to the previous version quickly to minimize downtime.
In the MERN stack, each component (MongoDB, Express.js, React, and Node.js) might require specific considerations for deployment. For instance, you might use Docker containers to package your Node.js and Express.js backend, ensuring it runs consistently across different environments. React apps might be minified and bundled for optimal performance.
In essence, deployment in the MERN stack is about getting your application from your local machine to a server where it can serve users reliably and efficiently. It involves careful planning, choosing the right hosting solutions, automating the process as much as possible, and being prepared to address issues post-deployment.
Top Resources to Learn the MERN Stack
Now that you have a pretty good idea of what you need to learn to master the MERN stack, lets look at a list of free resources you can start using today to begin your journey!
- The freeCodeCamp curriculum is one of the best places to learn how to code for free. The curriculum is super in depth and covers alot of the topics mentioned in this post.
- The freeCodeCamp YouTube channel has a ton of free courses you can try as well. This MERN stack book project is an excellent one for beginners.
- Full Stack open is another good resource. It doesn't teach MongoDB but you learn about SQL instead which is equally as useful.
- My MERN stack hotel booking app project on YouTube is a free 15 hour course where you will learn everything talked about in this post.
10 Project Ideas You Can Try Today
One of my favourite ways to learn new technologies is to build projects. If you're struggling with coming up with ideas, here's 10 projects you can start building today. I'll be building some of these on my YouTube channel so feel free to subscribe to stay up to date!
Personal Blog Website
- Functionality: Users can read posts, and the admin can create, edit, or delete posts.
- Learning Focus: Basic CRUD operations, user authentication, integrating React with Express API, and using MongoDB for data storage.
Task Manager (To-Do List)
- Functionality: Users can add, view, edit, and delete tasks. Tasks can have deadlines and priority levels.
- Learning Focus: React state management, Express route handling, MongoDB operations, and basic UI development.
Simple E-commerce Site
- Functionality: Display products, add to cart, and checkout functionality. Admin features for adding or removing products.
- Learning Focus: React components for product listing, cart management, Express.js for product APIs, MongoDB for product data, and handling user inputs.
Recipe Sharing Application
- Functionality: Users can post, view, edit, and delete recipes. Implement a rating or comment system for interaction.
- Learning Focus: File upload for images, user-generated content management, and MongoDB schema design.
Budget Tracker
- Functionality: Users can input their expenses and income, categorize them, and view a summary of their finances.
- Learning Focus: Handling forms in React, creating RESTful services with Express, and effective data structuring in MongoDB.
Event Planning Application:
- Functionality: Users can create and manage events, send invitations, and track RSVPs.
- Learning Focus: Date handling in JavaScript, complex MongoDB documents, and Express middleware for authentication.
Fitness Tracker:
- Functionality: Log workouts, track progress over time, set fitness goals.
- Learning Focus: Data visualization with React, creating API endpoints for varied data types, and MongoDB for storing time-series data.
Chat Application:
- Functionality: Real-time chat rooms, private messaging, and user profiles.
- Learning Focus: WebSockets with Node.js for real-time communication, MongoDB for message storage, and React for dynamic UI updates.
Book Review Platform:
- Functionality: Users can post book reviews, rate books, and browse reviews by other users.
- Learning Focus: Integrating external APIs for book data, user-generated content moderation, and complex querying in MongoDB.
Local Business Directory:
- Functionality: Listing of local businesses with categories, user reviews, and contact information.
- Learning Focus: Geolocation, advanced querying and indexing in MongoDB, and creating a responsive layout with React.
Wrapping Up the MERN Stack Journey
As we reach the end of our exploration of the MERN stack, I hope this guide has illuminated the path for your journey into the world of full-stack development.
We've covered the fundamental components – MongoDB, Express.js, React, and Node.js – and delved into the practical aspects of building, testing, and deploying applications using this versatile stack.
Remember, the road to mastering the MERN stack is both challenging and rewarding. Each project you undertake will add to your skills and confidence. Use the resources and project ideas provided as stepping stones to build your portfolio and deepen your understanding.
The beauty of the MERN stack lies in its community-driven nature, so don't hesitate to seek help, collaborate, and share your experiences.
If you want to get in touch, you can reach me over at LinkedIn, or drop a comment on my YouTube channel. Good luck and happy coding!