Are you a new developer just getting started? Or are you a seasoned developer looking to expand your skill set?
Either way, the freeCodeCamp community's got you covered.
A lot of the time, learning how to program is not so much a straight line as it is a vast flowchart, with lots of repeated sections and loops:
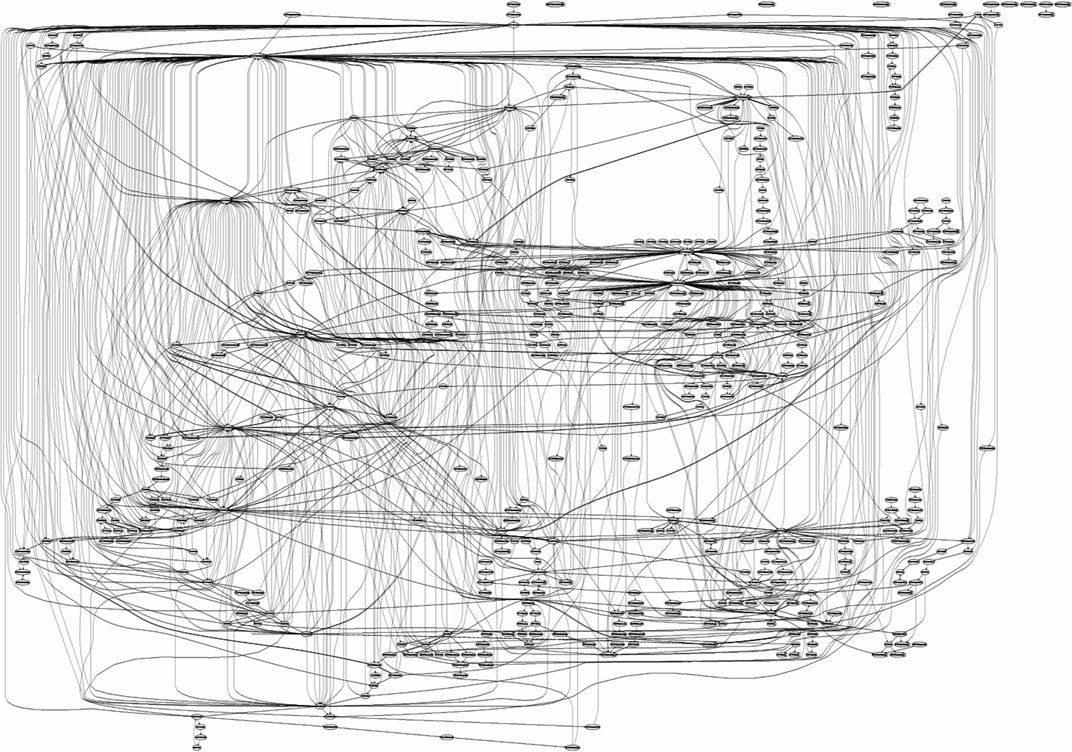
But it doesn't have to be that complicated.
I've gone through our vast catalog of tutorials and created a list of some of the best resources on how to learn pretty much everything you'd need to know as a developer.
The list is loosely organized into different sections and subsections. Feel free to browse the table of contents below and skip around to look for an article on whatever you're trying to learn.
Also, many things on this list require some prerequisite knowledge of another technology. Don't be surprised if you find yourself opening articles from multiple sections.
Finally, this is a living document, and will grow as we publish more helpful articles. Be sure to check back often, and share this with your friends if you find it helpful.
Table of Contents
- How to Build a Website
- How to Learn Programming
- How to Learn Linux
- How to Learn Git and Version Control
- How to Learn a Frontend Framework / Library
- How to Learn Web Basics and Web Security
- How to Learn Databases
- How to Learn Backend Development
- How to Learn Static Site Generators
- How to Learn Bundlers, Compilers, Dependency Managers, Task Runners, Formatters, and Linters
- How to Learn Mobile Application Development
- How to Learn Desktop Application Development
- How to Learn Data Science and Machine Learning
- How to Learn Virtualization and Containerization
- How to Learn Cloud Computing
- How to Learn DevOps
How to Build a Website
To build a basic website, all you really need are HTML (Hypertext Markup Language) and CSS (Cascading Style Sheets). HTML provides the content and structure of the site, and CSS is used to style it.
Here are some of the best resources on HTML and CSS. Once you're familiar with these technologies, move on to the next section and learn JavaScript to make your web sites more interactive.
HTML
- Learn HTML Basics for Beginners in Just 15 Minutes
- How to Make HTML Hyperlinks Using the HREF Attribute on Tags
- How to Use HTML to Open a Link in a New Tab
- HTML Link Code – How to Insert a Link to a Website with HREF
- HTML Entities – A List of HTML Space and other HTML Symbols and Special Character Codes
- How to Enable Dark Mode in HTML Email – Everything You Need to Know
- Make It Blink HTML Tutorial – How to Use the Blink Tag, with Code Examples
- HTML Basics: A Free Full-Length Course
- The HTML Handbook
CSS
- CSS Font Size Tutorial – How to Change Text Size in HTML
- HTML Background Color Tutorial – How to Change a Div Background Color, Explained with Code Examples
- Inline CSS Guide – How to Style an HTML Tag Directly
- HTML Center Text – How to CSS Vertical Align a Div
- How to Center Anything with CSS - Align a Div, Text, and More
- HTML vs Body: How to Set Width and Height for Full Page Size
- Box Shadow CSS Tutorial – How to Add a Drop Shadow to Any HTML Element
- How CSS Positioning and Flexbox Work – Explained with Examples
- Flexbox - The Ultimate CSS Flex Cheatsheet (with animated diagrams!)
- An Introduction to CSS Grid Layout (with Examples)
- Learn CSS Grid by Building 5 Layouts in 17 minutes
- How CSS Grid Changes the Way We Think About Structuring Our Content
- Flexbox vs Grid - How to Build the Most Common HTML Layouts
- Learn CSS in this free 6-hour video course
- The CSS Handbook: A Handy Guide to CSS for Developers
How to Learn Programming
In essence, programming is how humans tell computers what to do. Whether it's a laptop, a smartphone, or a browser, programming and programming languages give us a way to interact with those devices.
In this section, you'll learn the fundamentals of programming and the basics of some of the most popular programming languages today.
If you want to learn frontend and backend development, definitely learn JavaScript and Node.js. And once you're familiar with those, look into TypeScript.
If you're more interested in data science and machine learning, learn Python. For mobile apps, it helps to know Java. Game development? C++, C#, or even Java.
We've got a bit of everything, including newer programming languages like Go.
JavaScript
- JavaScript Variables – A Beginner's Guide to var, const, and let
- JavaScript Split String Example – How to Split a String into an Array in JS
- JavaScript TypeOf – How to Check the Type of a Variable or Object in JS
- How to Check if a JavaScript Array is Empty or Not with .length
- JS For Loop Tutorial – How to Iterate Over an Array in JavaScript
- JavaScript Array Sort – How to Use JS Sort Methods (With Code Examples)
- JavaScript Reverse Array – Tutorial With Example JS Code
- JavaScript forEach – How to Loop Through an Array in JS
- JavaScript Array Slice vs Splice: the Difference Explained with Cake
- JavaScript Object Keys Tutorial – How to Use a JS Key-Value Pair
- JavaScript Create Object – How to Define Objects in JS
- Object Oriented Programming in JavaScript – Explained with Examples
- The JavaScript
this
Keyword + 5 Key Binding Rules Explained for JS Beginners - A Beginner’s Guide to JavaScript’s Prototype
- JavaScript Date Now – How to Get the Current Date in JavaScript
- The Ultimate Guide to JavaScript Date and Moment.js
- What is Functional Programming? A Beginner's JavaScript Guide
- Learn JavaScript - Full 134-Part Course for Beginners
- Data Structures and Algorithms in JavaScript - Full Course for Beginners
- Sparse Arrays vs Dense Arrays in JavaScript — Explained with Examples
- JavaScript Regex Match Example – How to Use JS Replace on a String
- A Quick and Simple Guide to JavaScript Regular Expressions
- JavaScript Keycode List – Keypress Event Key Codes for Enter, Space, Backspace, and More
- JavaScript Object Destructuring, Spread Syntax, and the Rest Parameter – A Practical Guide
- How the Nullish Coalescing Operator Works in JavaScript
- Try/Catch in JavaScript – How to Handle Errors in JS
- How to Use Async/Await in JavaScript with Example JS Code
- How the Question Mark (?) Operator Works in JavaScript
- Ternary Operator JavaScript If Statement Tutorial
- Debounce – How to Delay a Function in JavaScript (JS ES6 Example)
- How to Find the Number of Vowels in a String with JavaScript
- Data Validation – How to Check User Input on HTML Forms with Example JavaScript Code
- What is Recursion? A Recursive Function Explained with JavaScript Code Examples
- The JavaScript Skills You Need For React (+ Practical Examples)
- Higher Order Functions in JavaScript – Reach New Heights in Your JS Code
- Node Module Exports Explained – With JavaScript Export Function Examples
- How to Make a Landing Page using HTML, SCSS, and JavaScript
- How to Build and Validate Beautiful Forms with Vanilla HTML, CSS, & JS
- How to Build a Responsive and Dynamic Progress Bar with HTML, CSS, and JavaScript
- The JavaScript Beginner's Handbook
Node.js
- How to Install Node.js and npm on Windows
- How to Install Node.js on Ubuntu and Update npm to the Latest Version
- Node Module Exports Explained – With JavaScript Export Function Examples
- npm Cheat Sheet - Most Common Commands and nvm
- What is npm? A Node Package Manager Tutorial for Beginners
- How to Ignore Files from Your npm Package
- How to Publish Packages to npm (the Way the Industry Does Things)
- How to Make a Beautiful, Tiny npm Package and Publish It
- How to Force Use Yarn or NPM
- How to enable ES6 (and beyond) syntax with Node and Express
- How to Automate Simple Tasks with Node.js
- The Ultimate Node.js Production Checklist
- How to Get Started with GraphQL and Node.js
TypeScript
- How to Install and Start Using TypeScript
- How to Add TypeScript to a JavaScript Project
- Learn TypeScript Data Types – From Zero to Hero
- All about TypeScript Static Members | TypeScript OOP
- No, Getters and Setters in TypeScript & JavaScript Aren't Useless
- A Crash Course in TypeScript
- TypeScript Types Explained – A Mental Model to Help You Think in Types
- The React TypeScript Cheatsheet – How To Set Up Types on Hooks
- How TypeScript Generics Help You Write Less Code
- How to Create a Great User Experience with React, TypeScript, and the React Testing Library
- Advanced TypeScript Types Cheat Sheet (with Examples)
- A Practical Guide to TypeScript - How to Build a Pokedex App Using HTML, CSS, and TypeScript
- How to Build a Todo App with React, TypeScript, NodeJS, and MongoDB
- How to Build a RocketChat Chatbot with TypeScript
- The Definitive TypeScript Handbook
- Learn TypeScript With This Crash Course
- How to Build a Quiz App Using React and TypeScript
- Build a Shopping Cart with React and TypeScript
- How to Use Typescript in React
Deno
- Learn Deno, a Node.js Alternative
- How to Build React Applications with Deno Using the AlephJS Library
- How to Build a URL Shortener in Deno
- How to Create a Todo API in Deno and Oak
- The Deno Handbook: A TypeScript Runtime Tutorial with Code Examples
- Securing Node.js RESTful APIs with JSON Web Tokens
- Learn Node.js and Start Executing JavaScript Outside of the Browser
Python
- Hello World Programming Tutorial for Python
- Python While Loop Tutorial – While True Syntax Examples and Infinite Loops
- Python New Line and How to Python Print Without a Newline
- Python Dictionaries 101: A Detailed Visual Introduction
- Python Sets: A Detailed Visual Introduction
- Python Read JSON File – How to Load JSON from a File and Parse Dumps
- Python List Files in a Directory Guide - listdir VS system("ls") Explained with Examples
- Python Write to File – Open, Read, Append, and Other File Handling Functions Explained
- Python Empty List Tutorial – How to Create an Empty List in Python
- Python List Append – How to Add an Element to an Array, Explained with Examples
- Python List Append VS Python List Extend – The Difference Explained with Array Method Examples
- The Python Sort List Array Method – Ascending and Descending Explained with Examples
- Python Unique List – How to Get all the Unique Values in a List or Array
- Truthy and Falsy Values in Python: A Detailed Introduction
- The Python Modulo Operator - What Does the % Symbol Mean in Python? (Solved)
- Python's datetime Module – How to Handle Dates in Python
- How to Handle Exceptions in Python: A Detailed Visual Introduction
- The @property Decorator in Python: Its Use Cases, Advantages, and Syntax
- The Python Sleep Function – How to Make Python Wait A Few Seconds Before Continuing, With Example Commands
- Mutable vs Immutable Objects in Python – A Visual and Hands-On Guide
- How to Build Your Very First Python Package
- Python Dictionary Guide – How to Iterate Over, Copy, and Merge Dictionaries in Python 3.9
- Binary Search in Python: A Visual Introduction
- Multithreaded Python: Slithering Through an I/O Bottleneck?
- How to Set Up a Python Virtual Environment on Ubuntu 20.04
- How to Set Up Virtualenv with Virtualenvwrapper on Ubuntu 18.04
- Installing Multiple Python Versions on Windows Using Virtualenv
- Take Your Python Skills to the Next Level With This Free 6-Hour Video Course
- The Python Handbook
Java
- Java String to Int – How to Convert a String to an Integer
- Java List Methods Tutorial – Util List API Example
- Java Array Methods – How to Print an Array in Java
- Using Java’s Arrays.sort() for any List of Objects
- How to Handle NullPointerException in Java
- Priority Queues in Java Explained with Examples
- Object-Oriented Programming Principles in Java: OOP Concepts for Beginners
- Polymorphism in Java Tutorial – With Object Oriented Programming Example Code
- Learn Functional Programming in Java - Full Course
- Multithreading in Java: How to Get Started with Threads
- Java Random Number Generator – How to Generate Integers With Math Random
- Garbage Collection in Java – What is GC and How it Works in the JVM
- JVM Tutorial - Java Virtual Machine Architecture Explained for Beginners
- Build a Java Android App Using a REST API - Network Data in Android Course
- How to Set Up Java Spring Boot JWT Authorization and Authentication
- JVM Tutorial - Java Virtual Machine Architecture Explained for Beginners
- Use Spring Boot and Java to Create a Rest API (Tutorial)
- How to Build a Sudoku Game Java Desktop Application – A Free 2-hour Course
Go (Golang)
- Go (Golang) Programming Language
- Learning Go — From Zero to Hero
- How to Automate Your GitHub Profile README
- How to Build Your Own Serverless Subscriber List with Go and AWS
- How to Validate SSL Certificates in Go
- How to Design a Transactional Key-value Store in Go
- How I built a Web Server Using Go — and On ChromeOS
- How to Set Up gRPC Server-Side Streaming with Go
- How to Set Up a Real-World Project with Go and Vue
- How to Implement Elasticsearch in Go
- How to Implement Heap-Sort in the Go Standard Library
- Learn the Fast and Simple Go Programming Language (Golang) in 7 Hours
- Learn Go in This Crash Course
Rust
- Rust for Beginners – Get Started with the Most Loved Programming Language
- How to Learn Rust Without Installing Any Software
- Rust Programming Language Tutorial – How to Build a To-Do List App
- How to Build Powerful GraphQL Servers with Rust
C
- Boost Your Programming Skills by Reading Git's Code
- Format Specifiers in C
- File Handling in C — How to Open, Close, and Write to Files
C++
- How Classes Work in C++
- Do While Loops in C++ with Example Loop Syntax
- How to Overload Operators in C++
- C++ Map Explained with Examples
- How to Write Clean Code in C++
- How to Compile Your C++ Code in Visual Studio Code
- Learn Object Oriented Programming (OOP) in C++ | Full Video Course
C#
- C# Programming: An Intro for Beginners
- C# Basics - Your First C# Program, Types and Variables, and Flow Control Statements
- Is the C# Internal Keyword a Code Smell?
- How to Build an SPA with Vue.js and C# Using .NET Core
- Learn C# and Unity by Making Digital Tabletop Games
- Create a C# Application from Start to Finish - Complete 24-Hour Course
How to Learn Linux
Whether you know it or not, you probably use Linux every day. Android is based on Linux, and macOS, which is based on Unix just like Linux, is a close cousin. And an estimated 74.2% (as of March, 2021) of all web servers run on Unix, the vast majority of which are probably Linux.
In short, if you work on the web, you should get comfortable with Linux and its default shell, Bash. And here are some of our best tutorials to get started:
- Linux Commands - Basic Bash Command Line Tips You Should Know
- The Cat Command in Linux – Concatenation Explained with Bash Examples
- The Cat Command in Linux – How to Create a Text File with Cat or Touch
- Grep Command Tutorial – How to Search for a File in Linux and Unix with Recursive Find
- Linux: How to Add Users and Create Users with useradd
- Linux User Groups Explained: How to Add a New Group, a New Group Member, and Change Groups
- The Linux LS Command – How to List Files in a Directory + Option Flags
- Tar in Linux – Tar GZ, Tar File, Tar Directory, and Tar Compress Command Examples
- The Tar Command in Linux: Tar CVF and Tar XVF Explained with Example Commands
- Symlink Tutorial in Linux – How to Create and Remove a Symbolic Link
- Linux Package Management with Snaps
- How to Build Your Own Linux Dotfiles Manager from Scratch
- Learn the Basics of Linux and How It Can Be Used by Ethical Hackers
- How to Configure and Operate Linux Servers - Full Course
- The Linux Command Handbook
How to Learn Git and Version Control
Once your programs start growing in size and complexity, you'll want a way to track your changes in case you need to roll back to an earlier version.
Git lets you do just that, and is the most popular version control software in use today. If you want to collaborate with other developers and get a job in the industry, it's important to know how Git works.
Some of our best articles on Git are listed below. Also, when you use Git it'll probably be through the command line, so make sure you know some basic Linux / Bash before diving in.
- What is Git? A Beginner's Guide to Git Version Control
- Learn Git and Version Control in an Hour
- Git vs GitHub – What is Version Control and How Does it Work?
- What is GitHub? What is Git? And How to Use These Developer Tools
- Git Commands You Should Know, with Code Examples
- Git Cheat Sheet – 50 Git Commands You Should Know
- Git Reset to Remote Head – How to Reset a Remote Branch to Origin
- Git Checkout Remote Branch Tutorial
- How to Use Branches in Git – the Ultimate Cheatsheet
- A Beginner’s Guide to Git — How to Write a Good Commit Message
- How to Write Good Commit Messages: A Practical Git Guide
- A Beginner’s Guide to Git — What is a Changelog and How to Generate it
- How to Get and Configure Your Git and GitHub SSH Keys
- How to Use Multiple Git Configs on One Computer
- How to Understand and Solve Conflicts in Git
- How to Undo Mistakes with Git
- How to Use Git Aliases to Increase Your Productivity
- Git Reset Explained – How to Save the Day with the Reset Command
- Git Secrets: 7 Commands You Might Not Know
- How to Uncommit Sensitive Files from Git
- Git Pull Force – How to Overwrite Local Changes With Git
- Git Clone Branch – How to Clone a Specific Branch
- How to Switch Between Issues in Your Local Git Repository
- How to Sync Your Fork with the Original Git Repository
- Git and GitHub Crash Course
How to Learn a Frontend Framework / Library
Once you know how to build basic websites with HTML, CSS, and JavaScript, level up your skills by learning a frontend framework / library. Of these, the three most popular are React, Vue, and Angular.
Angular is considered a framework because it includes a lot of things like routing out of the box.
React, on the other hand, is usually referred to as a library because it doesn't come with a lot by default. Instead, you'll need to add some extra packages to handle routing and other things.
Vue falls somewhere in the middle in terms of functionality and weight.
Whatever you call them, each has its own strengths and weaknesses. There is no best framework / library – just choose one that seems the most interesting, or that companies in your area are hiring for, and go from there.
React
- How to Install React.js with create-react-app
- React Functional Components, Props, and JSX – React.js Tutorial for Beginners
- JSX in React – Explained with Examples
- React Background Image Tutorial – How to Set backgroundImage with Inline CSS Style
- How to Build an Accordion Menu in React from Scratch – No External Libraries Required
- How to Build React Forms the Easy Way with react-hook-form
- How to Build Your Own React Hooks: A Step-by-Step Guide
- React Testing Library – Tutorial with JavaScript Code Examples
- How to Build a Weather Application with React and React Hooks
- How to Add Drag and Drop in React with React Beautiful DnD
- How to Use SVG Icons in React with React Icons and Font Awesome
- How to Build a Shopping List Using React Hooks (w/ Starter Code and Video Walkthrough)
- Build a React Budget Tracker App – Learn React & Context API with this Fun Project
- The Best File Structure for Your React Components
- React Props Cheatsheet: 10 Patterns You Should Know
- How to Turn Google Sheets into a REST API and Use it with a React Application
- How to Fetch Data in React: Cheat Sheet + Examples
- How to Use the YouTube IFrame API in React
- How to Setup HTTPS Locally with create-react-app
- How to Create a React App with a Node Backend: The Complete Guide
- How to Add a Serverless Database to your React Projects
- The React Scripts Start Command – Create-React-App npm Scripts Explained
- Build a Shopping Cart with React and TypeScript
- Learn React.js by Building Projects – Create a Birthday Reminder App
- How to Create a Next.js Starter to Easily Bootstrap a New React App
- Learn How to Use React and GraphQL to Make a Full Stack Social Network
- React for Beginners – A React.js Handbook for Front End Developers
Vue
- Learn Vue: A 3-minute Interactive Vue JS Tutorial
- Learn How to Use the Vue.js CLI
- Learn Vue.js - Full Course for Beginners
- Vue Components: An Interactive Vue JS Tutorial
- How to Use Routing in Vue.js to Create a Better User Experience
- Build a Markdown Previewer with Vue.js
- How to Add Internationalization to a Vue Application
- How to Add Charts and Graphs to a Vue.js Application
- How to Build a Memory Card Game with Vue.js
- How to Create and Publish a Vue Component Library
- How to Build a Full Stack RPG Character Generator with MongoDB, Express, Vue, and Node (the MEVN Stack)
- How to Add Authentication to a Vue App Using Firebase
- How to Add Authentication to a Vue App Using Auth0
- How to Add Authentication to a Vue App Using AWS Amplify
- The Vue Handbook: A Thorough Introduction to Vue.js
Angular
- How to Install Angular on Windows: A Guide to Angular CLI, Node.js, and Build Tools
- Angular 9 for Beginners - Components and String Interpolation
- Angular 9 for Beginners — How to Install Your First App with Angular CLI
- Everything you need to know about ng-template, ng-content, ng-container, and *ngTemplateOutlet in Angular
- What Could Go Wrong? How to Handle Errors in Angular
- How to Build a Generic Form Validator in Angular
- How to Validate Angular Template-Driven Forms
- How to Validate Angular Reactive Forms
- How to Create a Reusable Loading-Indicator for Angular Projects
- How I Built a Customizable Loading-Indicator with Angular Dynamic Components
- How to Create an Online Poll with ASP.NET Core, Angular 5, and Highcharts
- How to Generate QR Codes in Angular 10
- Use Angular Material to add modern UI components to your Angular projects
- Angular RxJS In-Depth
- How To Create An Optical Character Reader Using Angular And Azure Computer Vision
- Learn Angular - Full Tutorial Course
How to Learn Web Basics and Web Security
When you get familiar with building web sites and web applications in the framework / library of your choice, you'll want to deploy them. But before you put your work online, it helps to know how the web works and the basics of web security.
Web Basics
- How HTTP Works and Why it's Important – Explained in Plain English
- HTTP 401 Error vs HTTP 403 Error – Status Code Responses Explained
- HTTP Error 403 Forbidden: What It Means and How to Fix It
- Error 403 Forbidden Explained - How Can I Fix This HTTP Error Code?
- HTTP Error 500 – Internal Server Error Explained in Plain English
- HTTP Error 503 Service Unavailable Explained – What the 503 Error Code Means
- An In-depth Introduction to HTTP Caching: Cache-control & Vary
- An Introduction to HTTP: Everything You Need to Know
- What is the TCP/IP Model? Layers and Protocols Explained
- WPA Key, WPA2, WPA3, and WEP Key: Wi-Fi Security Explained
- What is TLS? Transport Layer Security Encryption Explained in Plain English
HTTPS
- What is HTTPS? A Guide to Secure Web Browsing and Browser Encryption
- WTF is HTTPS?
- How to Protect Your WordPress Website With HTTPS in 5 Simple Steps
- How to Redirect HTTP to HTTPS Using .htaccess
- Simple Site Hosting with Amazon S3 and HTTPS
- HTTPS Explained with Carrier Pigeons
- How to Get HTTPS Working on Your Local Development Environment in 5 Minutes
- How to Add HTTPS to Your Website for Free in 10 Minutes, and Why You Need to Do This Now More Than…
Cookies
- Web Security: How to Harden Your HTTP Cookies
- Everything You Need to Know About Cookies for Web Development
- What are Cookies on the Web and How Do You Use Them?
How to Learn Databases
By this point you've probably built a bunch of websites and applications. You've likely used an API to get data on things like the weather, or to grab a random quote to display on the page.
But if you've ever wanted to create your own API, or store information from your users, you'll need to learn how to use a database.
Generally speaking, databases fall into two categories: relational, or SQL, and non-relational, or NoSQL. SQL stands for "structured query language", and is a broad term to refer to relational databases. NoSQL, or "not only SQL" refer to non-relational databases.
Neither type of database is better or worse than the other – it mostly comes down to the project you're working on, and the sort of data you'll be working with.
Here's a list of some of the best articles we have on databases. I'll make a note about whether the database system is relational (SQL) or non-relational (NoSQL) if it isn't clear:
SQL / MySQL
- What is SQL? What is a Database? Relational Database Management Systems (RDBMS) Explained in Plain English
- Why You Should Learn SQL – Even If You're Not a Developer
- Basic SQL Commands - The List of Database Queries and Statements You Should Know
- Learn SQL with These 5 Easy Recipes
- SQL Create Table Statement - With Example Syntax
- SQL Operators Tutorial – Bitwise, Comparison, Arithmetic, and Logical Operator Query Examples
- SQL Joins Tutorial: Cross Join, Full Outer Join, Inner Join, Left Join, and Right Join
- SQL Foreign Key VS Primary Key Explained with MySQL Syntax Examples
- SQL View Explained - How to Create a View in SQL and MySQL
- The SQL Update Statement Explained: Queries for Updating Tables (including MySQL Examples)
- SQL Insert Into and Insert Statements: With Example MySQL Syntax
- SQL Create Table Explained with Syntax Examples for MySQL and Postgres
- Check Constraint in SQL - Explained with MySQL and SQL Server Syntax Examples
- SQL Delete Row Statement - How to Remove Data From a Table With Example Queries
- Primary Key SQL Tutorial – How to Define a Primary Key in a Database
- Learn the Basics of SQL Injection and How to Protect Your Web Apps
- SQL Injection Tutorial - What is SQL Injection and How to Prevent it
- SQL Update Statement — Example Queries for Updating Table Values
- How to Make Sure Your MySQL Database is Secured
- How to Create and Manipulate SQL Databases with Python
- How to Build Your First CRUD App with Laravel and MySQL
- SQL and Databases - A Full Course for Beginners
MongoDB / Mongoose (NoSQL)
Note: Mongoose is a tool for MongoDB that lets you do things like object data modeling (ODM) to create models or schemas for your data. A lot of people use Mongoose to interact with a MongoDB database, so I've combined them here.
- How to Get Started with MongoDB in 10 Minutes
- How to Handle Advanced Data Processing with MongoDB's Aggregation Framework
- Learn Node + MongoDB by Creating a URL Shortener Project
- How to Use MongoDB + Mongoose with Node.js – Best Practices for Back End Devs
- How to Deploy a MERN Application to Heroku Using MongoDB Atlas
- How to Build a Todo App with React, TypeScript, NodeJS, and MongoDB
- How to Build a Full Stack RPG Character Generator with MongoDB, Express, Vue, and Node (the MEVN Stack)
- How to build a blazing fast GraphQL API with Node.js, MongoDB and Fastify
- How to Create a Realtime App Wsing Socket.io, React, Node & MongoDB
- How to Build Blazing Fast REST APIs with Node.js, MongoDB, Fastify and Swagger
- Introduction to Mongoose for MongoDB
- How to Log a Node.js API in an Express.js App with Mongoose Plugins
- Mongoose 101: An Introduction to the Basics, Subdocuments, and Population
- How to Allow Users to Upload Images with Node/Express, Mongoose, and Cloudinary
- MongoDB Quickstart with Python
- MongoDB Tutorial - CRUD App from Scratch Using Node.js
- MongoDB Full Course w/ Node.js, Express, & Mongoose
- How to Build a RESTful API Using Node, Express, and Mongo
Redis (NoSQL)
- How to Use Redis to Supercharge Your Web APIs
- A Quick Guide to Redis Lua Scripting
- How the Redis Hash Table Scan Function Works
- How to Build a Multi-Step Registration App with Animated Transitions Using the MERN Stack
Postgres / PostgreSQL
- How to Get Started with PostgreSQL
- Learn These Quick Tricks in PostgreSQL
- How to Use Fuzzy String Matching with PostgreSQL
- How to Update Objects Inside JSONB Arrays with PostgreSQL
- How to Deploy a Rails 5.2 PostgreSQL App on AWS Elastic Beanstalk
- How to Create a Django Server Running uWSGI, NGINX and PostgreSQL on AWS EC2 with Python 3.6
- How to Build Web APIs with NestJS, Postgres, and Sequelize - A Beginner's Guide
- How to Deploy a React App to Production on AWS Using Express, Postgres, PM2 and NGINX
- Docker Development WorkFlow — a Guide with Flask and Postgres
- Learn SQL with This Free 4-hour Course on the Popular PostgreSQL Database
How to Learn Backend Development
Similar to how frontend development is a broad subject, backend development can refer to many things, and encompasses a lot of different technologies.
Usually when you start working on the backend, which controls how sites and web apps work behind the scenes, you'll use a framework like Express, Flask, or Django.
Express
- How to Enable ES6 (and beyond) Syntax with Node and Express
- How to Deploy Your App to the Web Using Express.js and Heroku
- How to Add a GraphQL Server to a RESTful Express.js API in 2 Minutes
- Express.js Security Tips: How You Can Save and Secure Your App
- How to Build a Full Stack RPG Character Generator with MongoDB, Express, Vue, and Node (the MEVN Stack)
- How to Build a Multiplayer Card Game with Phaser 3, Express, and Socket.IO
- How to Build a Multiplayer Tabletop Game Simulator with Vue, Phaser, Node, Express, and Socket.IO
- How to Make Input Validation Simple and Clean in Your Express.js App
- How to Write a Production-Ready Node and Express App
- How to Build a RESTful API using Node, Express, and Mongo
- Learn Express.js in This Complete Course
Flask
- How to Develop an End-to-End Machine Learning Project and Deploy it to Heroku with Flask
- Learn About Python Microservices by Building an App Using Django, Flask, and React
- How to Use Python and Flask to Build a Web App — An In-Depth Tutorial
- Setting up CI/CD on GitLab for Deploying Python Flask Application on Heroku
- Learn Flask Web Development for Python in This Free 1-hour Course
- Learn Web Programming with Flask from Harvard's CS50
- Learn the Flask Python Web Development Framework by Building an Ecommerce Platform
Django
- How to Write Efficient Views, Models, and Queries in Django
- How to Manipulate Data with Django Migrations
- Django Project Best Practices That'll Keep Your Developers Happy
- Django Test Suite Introduction – How to Increase Your Confidence as a Python Developer
- ELI5 Full Stack Basics: Breakthrough with Django & EmberJS
- I Built a Members' Area on My Website with Python and Django. Here's What I Learned.
- How to Build a Web-Based Dashboard with Django, MongoDB, and Pivot Table
- How to Create an Analytics Dashboard in a Django App
- How to Build an E-commerce Website with Django and Python
- Build a Moodle / Blackboard clone with Django Rest Framework & React
- How to Build a Progress Bar for the Web with Django and Celery
- How to Document Your Django Project Using the Sphinx Tool
- Python Django Web Framework - Full Course for Beginners
- Learn About Python Microservices by Building an App Using Django, Flask, and React
How to Learn Static Site Generators
Static Site Generators were created to make development easy, and they represent the "M" in JAMstack (JavaScript, APIs, and Markup). With a static site generator, it's much easier to create a quick, scaleable website, blog, or web app with modern benefits like server-side rendering.
Gatsby
- Gatsby Starter Blog: How to Add Header Images to Posts with Support for Twitter Cards
- How to Create an Image Gallery Using Gatsby and Cloudinary
- How to Build a Blog with Gatsby and Netlify CMS – A Complete Guide
- Create a Full-Stack Website with Strapi and GatsbyJS
- How to Create a Travel Bucket List Map with Gatsby, React Leaflet, & GraphCMS
- How to Enable Offline Mode for Your Gatsby Site
- What Are Environment Variables and How Can I Use Them with Gatsby and Netlify?
- 3 Ways to Edit Markdown with TinaCMS + Gatsby
- How to Build Your Coding Blog From Scratch Using Gatsby and MDX
- What Is Gatsby and Why It's Time to Get on the Hype Train
- How to Build Authenticated Serverless JAMstack Apps with Gatsby and Netlify
- How to Keep State Between Pages with Local State in Gatsby.js
- How to Automatically Cross-post from Your GatsbyJS Blog with RSS
- How to Create a Searchable Log with Gatsby
- From Zero to Deploy: How I Created a Static Website from Scratch Using Netlify + Gatsby
- Get Your GraphCMS Data into Gatsby
- The Great Gatsby.js Bootcamp
Next.js
- What is Static Site Generation? How Next.js Uses SSG for Dynamic Web Apps
- Next.js Basics Tutorial – Server-side Rendering, Static Sites, REST APIs, Routing, and More
- Routing in Next.js – A Complete Beginner's Guide
- How to Create a Next.js Starter to Easily Bootstrap a New React App
- How to Build a Jamstack Site with Next.js and Vercel - Jamstack Handbook
- How to Create a Contact Form with Netlify Forms and Next.js
- How to Build an Image Gallery with NextJS Using the Pexels API and Chakra UI
- How to Add Interactive Animations and Page Transitions to a Next.js Web App with Framer Motion
- How to Use Github Actions to Deploy a Next.js Website to AWS S3
- How to Use Chakra UI with Next.js and React
- How to Run Visual Regression Testing on a Next.js App with Cypress and Applitools
- How to Fetch GraphQL Data in Next.js with Apollo GraphQL
- Discover Next.js and Write Server-Side React Apps the Easy Way
- The Next.js Handbook
Hugo
- How to Create Your First Hugo Blog: a Practical Guide
- A Portable Makefile for Continuous Delivery with Hugo and GitHub Pages
- Two Ways to Deploy a Public GitHub Pages Site from a Private Hugo Repository
- Hugo + Firebase: How to Create Your Own Static Website for Free in Minutes
- Hugo vs Jekyll: an Epic Battle of Static Site Generator Themes
- How to Self-Host a Hugo Web App
Nuxt.js
- How to Use Flat-File Data in a Static Nuxt App
- Up & Going with Nuxt.js, Bulma and Sass
- Universal Application Code Structure in Nuxt.js
- How to Architect a DApp Using Nuxt.js and Nebulas
- Deploy a Nuxt App to S3 in 5 Minutes
Vuepress
How to Learn Bundlers, Compilers, Dependency Managers, Task Runners, Formatters, and Linters
Once you start working with frontend frameworks / libraries, or your projects start to grow in size and complexity, things can quickly get out of hand.
To keep things organized and tidy, it helps to learn linting, especially if you work on large teams. With linting, you can catch errors before they happen, and with a formatter like prettier, you can enforce a code style guide for your entire team.
And though a lot of Angular, Vue, and React projects already include a bundler like Webpack, it's helpful to learn more about how it works in case you need to adjust its behavior later.
Webpack and Babel
- An Intro to Webpack: What It Is and How to Use It
- How to Create a Production-Ready Webpack 4 Config From Scratch
- How to Share Variables Across HTML, CSS, and JavaScript Using Webpack
- How to Combine Webpack 4 and Babel 7 to Create a Fantastic React App
- How to Set up & Deploy Your React App from Scratch Using Webpack and Babel
- How to Use Babel Macros with React Native
- Learn Webpack to Simplify and Speed Up Your Website
ESLint and Prettier
- What Is Linting and How Can It save You Time?
- Don’t Just Lint Your Code - Fix It with Prettier
- How to Create Your Own ESLint Config Package
- ESLint: The Essential Facts About Essential Front End Tools
- How to Stop Errors Before They Ever Hit Your Codebase with Travis CI and ESLint
Parcel
- How to Set Up a React App with Parcel
- How to Use Parcel to Bundle Your React.js Application
- How to Build Chrome Extensions with React + Parcel
- Using Parcel Bundler with React
Gulp
- Super Simple Gulp Tutorial for Beginners
- Using Gulp 4 in Your Workflow for Sass and JS Files
- How to Minify Images with Gulp & Gulp-imagemin and Boost Your Site’s Performance
npm Scripts
- Why I Left Gulp and Grunt for npm Scripts
- The React Scripts Start Command – Create-React-App npm Scripts Explained
How to Learn Mobile Application Development
These days, a lot of mobile app development is done with a framework like React Native.
While in the past you had to know a specific language like Java to develop a mobile app, with a framework, a lot of your frontend framework / library knowledge can be used to develop a mobile app.
Also, if you use a framework, you can just build the app once, and create both iOS and Android versions from the same code base.
React Native
- How Animations Work in React Native
- How to Use Video As a Background in React Native
- How to Handle Navigation in React Native with react-navigation 5
- Why I Switched to React Native to Create a Super Easy Bottom Sheet
- How React Native Constructs App Layouts (and How Fabric is About to Change It)
- How to Create a Camera App with Expo and React Native
- How to Build Your First Serverless React Native App with User Authentication
- How to Add Authentication to React Native in Three Steps Using Firebase
- How to Build a React Native App and Integrate It with Firebase
- How to Set Up Google Login in React Native & Firebase
- Add Gestures and Animations to React Native Projects
- How to Use Babel Macros with React Native
- Build an Instagram Clone with React Native, Firebase Firestore, Redux, and Expo
- React Native Course: How to Build an iPhone App, Android App, and Website - All with the Same Codebase
- How to Integrate Redux into Your Application with React Native and Expo
- How to Convert a React App to React Native
- Intro to React Native Course
Ionic
- How to Write "Hello, World!" in Ionic
- How to Create a CRUD To-do App Using Ionic 3
- How to Build Your First Ionic 4 App with API Calls
- How to Get Push Notifications Working with Ionic 4 and Firebase
- How to Develop a Great Facebook Login Flow with Firebase and Ionic
- How to Integrate Google Login into an Ionic App with Firebase
- Learn Ionic 4 and start creating iOS / Android Apps
Flutter
- A Simplified Introduction to Dart and Flutter
- An Introduction to Flutter: The Basics
- How to Serialize An Object In Flutter
- How to Handle State in Flutter Using the BLoC Pattern
- How to Use Streams, BLoCs, and SQLite in Flutter
- How to Handle Navigation in Your Flutter Apps
- How to Use the Provider Pattern in Flutter
- How to Build a Chat App UI With Flutter and Dart
- How to Add Push Notifications to a Flutter App using Firebase Cloud Messaging
- How to Integrate Google AdMob into Flutter
- How to Build a Native Communication Bridge in Flutter with WebView and JavaScript
- How to Use Flutter to Build a Tip Calculator
- How to Build a Cryptocurrency Price List App Using Flutter SDK
- Flutter UI Tutorial – How to Build a Chat App with Stories Using the Flutter SDK
- Flutter Course – How to Create a Production iPhone and Android App with the Flutter UI Tookit
- Use Flutter to Make an App for Mobile, Web, and Desktop - All with One Codebase
- Learn to Build iOS and Android Apps with Flutter
How to Learn Desktop Application Development
Similar to modern mobile app development, a lot of desktop apps these days are developed using a framework. This has a lot of the same advantages, and means that you can write your desktop app just once, and create Windows, macOS, and even Linux versions from the same code base.
Electron
- Writing OS-specific Code in Electron
- Building an Electron Application with create-react-app
- Quick, Painless, Automatic Updates in Electron
- Here’s How I created a Markdown App with Electron and React
- How to Create an Electron App Using Angular and SQLite3
- Things I Wish I Knew Before Working with Electron.js
- How to Build an Electron Desktop App in JavaScript: Multithreading, SQLite, Native Modules, and Other Common Pain Points
Proton Native
How to Learn Data Science and Machine Learning
Data science and machine learning are all the rage, and the number of jobs in each field is growing every year.
Put simply, data science refers to a broad range of techniques used to analyze and make sense of vast amounts of data.
Machine learning falls under the umbrella of data science, and it employs techniques that data scientists use to enable computers to learn from all this data.
It's a lot to take in, but no worries – here are some of the best articles and courses we have on machine learning, and the different libraries and frameworks you'll use on the job.
General Machine Learning
- Machine Learning Basics for Developers
- What Is a Convolutional Neural Network? A Beginner's Tutorial for Machine Learning and Deep Learning
- Clustering Algorithms in Machine Learning that All Data Scientists Should Know
- Key Machine Learning Algorithms Explained in Plain English
- Random Forest Classifier Tutorial: How to Use Tree-Based Algorithms for Machine Learning
- Google BERT NLP Machine Learning Tutorial
- SVM Machine Learning Tutorial – What is the Support Vector Machine Algorithm, Explained with Code Examples
- Machine Learning with Scikit-Learn—Full Course
Pandas
- The Ultimate Guide to the Pandas Library for Data Science in Python
- How to Get Started with Pandas in Python – a Beginner's Guide
- How to Use Python and Pandas to Map Major Storms, Pessimism, and Hard Data
- How to Analyze Data with Python, Pandas & Numpy - 10 Hour Course
Numpy
- The Ultimate Guide to the NumPy Package for Scientific Computing in Python
- Python NumPy Crash Course – How to Build N-Dimensional Arrays for Machine Learning
- Learn NumPy and Start Doing Scientific Computing in Python
Scikit-Learn
- Machine Learning with Scikit-Learn—Full Course
- How I Used Regression Analysis to Analyze Life Expectancy with Scikit-Learn and Statsmodels
Seaborn
Matplotlib
- Matplotlib Course – Learn Python Data Visualization
- How to Embed Interactive Python Visualizations on Your Website with Python and Matplotlib
- How to Create Auto-Updating Data Visualizations in Python with IEX Cloud, Matplotlib, and AWS
- Python Data Analysis: How to Visualize a Kaggle Dataset with Pandas, Matplotlib, and Seaborn
- Python Data Science – A Free 12-Hour Course for Beginners. Learn Pandas, NumPy, Matplotlib, and More
TensorFlow
- Learn How to Use TensorFlow 2.0 For Machine Learning in This Massive Free Course
- Learn Natural Language Processing with Python and TensorFlow 2.0 – No Machine Learning Experience Required
- Learn to Apply Deep Learning with Pytorch in This Full Course
- Learn to Develop Neural Networks Using TensorFlow 2.0 In This Beginner's Course
PyTorch
- PyTorch Tensor Methods – How to Create Tensors in Python
- How to Build a Neural Network from Scratch with PyTorch
- Learn How to Use PyTorch for Deep Learning
- Free Live Course: Deep Learning with PyTorch
Keras
- Keras Course – Learn Python Deep Learning and Neural Networks
- How to Classify Butterflies with Deep Learning in Keras
- How to Build Your First Neural Network to Predict House Prices with Keras
How to Learn Virtualization and Containerization
Once you learn the basics of Linux, you'll want to learn about virtual machines / virtualization, and containerization.
The main difference between the two is that virtualization is an abstraction on the hardware level, and allows multiple emulated machines to run on a single machine.
For example, with virtualization, you can split up a single machine's resources (CPU, SSD, RAM, and so on) into two smaller machines, with one running Windows server and another running Ubuntu.
On the other hand, containerization is emulation on the software level. This allows you to package applications and all their dependencies into a small, portable container that runs pretty much anywhere.
With containerization, you have a Node.js app that runs on Ubuntu. You can include your app, all its node_module
files, and even the entire Ubuntu OS, in a small ~1 GB container. VMs are typically between 20 - 160 GB in size.
But both are useful, and serve different purposes. Check out our tutorials below to learn more about both virtualization and containerization.
Virtual Machines
- Linux Server Virtualization: The Basics
- VirtualBox: Are You Getting Your Money’s Worth?
- How to install Ubuntu on VirtualBox
- What is a Virtual Machine And How to Setup a VM on Windows, Linux, and Mac
Docker
- What is Docker Used For? A Docker Container Tutorial for Beginners
- A Comprehensive Introduction to Docker, Virtual Machines, and Containers
- Docker 101 - How to Get from Creation to Deployment
- A Beginner’s Guide to Docker — How to Create Your First Docker Application
- Docker Remove Image: How to Delete Docker Images Explained with Examples
- How to Get A Docker Container IP Address - Explained with Examples
- How to Install Docker on Ubuntu 18.04 [Guide for both CE and EE]
- How to Run Docker on Windows 10 Home Edition
- How to Debug a Node.js Application with VSCode, Docker, and Your Terminal
- Docker Exec - How to Run a Command Inside a Docker Image or Container
- Where are Docker Images Stored? Docker Container Paths Explained
- Docker Data Containers
- Docker Image Guide: How to Delete Docker Images, Stop Containers, and Remove All Volumes
- Cleaning Up Docker
- A Quick Introduction to Docker Tags
- How to Enable Live-reload on Docker-based Applications with Docker Volumes
- A Practical Introduction to Docker Compose
- A Beginner’s Guide to Docker — How to Create a Client/Server Side with docker-compose
- Docker Deployment Guide – How to Deploy Containers to the Cloud with AWS Lightsail
- The Docker Handbook – 2021 Edition
- Free 4-Hour Course on Docker and Kubernetes
- Learn DevOps Basics with This Free 2-hour Docker Course
Kubernetes
- Kubernetes VS Docker: What's the Difference? Explained With Examples
- A Simple Introduction to Kubernetes Container Orchestration
- A Friendly Introduction to Kubernetes
- How to Develop Kubernetes Applications with Joy
- What is a Helm Chart? A Tutorial for Kubernetes Beginners
- Helm Charts Tutorial: The Kubernetes Package Manager Explained
- Learn Kubernetes in Under 3 Hours: A Detailed Guide to Orchestrating Containers
- Docker Swarm vs Kubernetes: How to Setup Both in Two Virtual Machines
- The Kubernetes Handbook
- An Introduction to the Helm Package Manager for Kubernetes
How to Learn Cloud Computing
Once you learn the basics about virtual machines, you'll want to learn about cloud computing.
Not too long ago, if a company wanted to run a server to host a website, they would have to build and maintain the server themselves.
With cloud computing, you can spin up virtual machine running your OS of choice in a few minutes. Better yet, the company hosting your VM will take care of the general maintenance for you, and ensure that the server is online and highly available.
And with cloud computing, you don't even have to have a server running 24/7 – with certain services, you can run a function and just pay for the milliseconds of time it took to complete.
Check out our tutorials below to learn more about cloud computing on the three big players in this space: Amazon Web Services, Google Cloud Platform, and Microsoft Azure.
Amazon Web Services (AWS)
- AWS Training – Learn the Basics of Amazon Web Services
- AWS Cheatsheet: The Top 5 Things to Learn First When Getting Started with Amazon Web Services
- Everything You Need to Know About AWS S3
- How to Spin Up a Remote Server on AWS
- How to Install the AWS Elastic Beanstalk CLI on a Mac
- AWS CLI Tutorial – How to Install, Configure, and Use AWS CLI to Understand Your Resource Environment
- How to Host a Static Site in the Cloud in Four Steps
- How to Host and Deploy a Static Website or JAMstack App to AWS S3 and CloudFront
- How to Host your Static Website with AWS - A Beginner's Guide
- Cron Job AWS Lambda Functions Tutorial – How to Schedule Tasks
- How to Build and Deploy AWS Applications on Your Local Machine
- How to Build a Serverless Application Using AWS SAM
- How to Build a Serverless Application Using AWS Chalice
- How to Design Almost Any Backend and Deploy It to AWS with No Code
- How to Add Authentication to a Vue App Using AWS Amplify
- How to Build a Screenshot Capture API Using Terraform, AWS API Gateway, and AWS Lambda
- How to Build Your Own Serverless Subscriber List with Go and AWS
- How to Secure Your Workloads on AWS
- How to Build a Full Stack App with AWS Amplify and React
- How to Use Github Actions to Deploy a Next.js Website to AWS S3
- How to Optimize your AWS Cloud Architecture Costs
- The Complete Guide to building an API with TypeScript and AWS
- How to Build and Deploy a GraphQL Server in AWS Lambda Using Node.js and CloudFormation
- How to Build a Complete Back End System with Serverless
- Simple Site Hosting with Amazon S3 and HTTPS
- Pass the AWS SysOps Administrator Associate Exam With This Free 14-Hour Course
- DynamoDB Cheatsheet – Everything you need to know about Amazon Dynamo DB for the 2020 AWS Certified Developer Associate Certification
- Pass the AWS Developer Associate Exam With This Free 16-Hour Course
Google Cloud Platform (GCP)
- Google Cloud Platform Tutorial: From Zero to Hero with GCP
- How to Create and Connect to Google Cloud Virtual Machine with SSH
- How to Pass Almost Every Google Cloud Platform Professional Certification Exam
- How to Run Laravel on Google Cloud Run with Continuous Integration - a Step by Step Guide
- How to perform CRUD operations using Blazor and Google Cloud Firestore
- The JavaScript + Firestore Tutorial for 2020: Learn by Example
- Firestore: How to Stay Within the Limits of Firebase's New Database Free Tier
- Build an Instagram Clone with React Native, Firebase Firestore, Redux, and Expo
- How to Get Started with Firebase Using Python
- How to Add Authentication to a Vue App Using Firebase
- How to Build an Android App with Firebase and Kotlin
- How to Authenticate Users And Save Data in a Database Using Firebase
- How to Set Up Google Login in React Native & Firebase
- How to Build a TodoApp Using ReactJS and Firebase
- How to Build an Event Booking App Using HTML, CSS, JavaScript, and Firebase
- How I Got Netlify Functions, Firebase, and GraphQL to Work Together At Last
- You Can't Get There from Here: How Netlify Lambda and Firebase Led Me to a Serverless Dead End
- Build an Evernote clone using React and Firebase (Video Tutorial)
- Learn How to Create a Social Media App from Scratch Using React, Firebase, Redux, and Express
Microsoft Azure
- How to Get Started with Microsoft Azure - Function Apps, HTTP Triggers, and Event Queues
- A Quick Introduction to Azure Function Proxies
- Making Sense of Azure Durable Functions
- An Introduction to Azure Durable Functions: Patterns and Best Practices
- How to Implement Azure Serverless with Blazor WebAssembly
- How to Use Azure Functions to Process High Throughput Messages
- Azure Fundamentals Certification (AZ-900) – Pass the Exam With This Free 3-Hour Course
How to Learn DevOps
Now that you know virtualization, containerization, and cloud computing, it's time to take things to the next level.
DevOps is equal parts software development and IT operations. If you're involved in DevOps, not only can you build an application, but you can spin up the VMs, deploy the app, monitor the servers, and scale the app and resources as more people start using it.
There's a lot to cover, and these articles should get you started on your DevOps path.
General DevOps
- The 2020 Web Developer Roadmap – A Visual Guide to Becoming a Front End, Back End, or DevOps Developer
- How to Make Your Startup’s Cloud More Stable: 4 Practical DevOps Tips
- Learn DevOps Basics with This Free 2-hour Docker Course
- Want to learn DevOps? This Free 3-Hour Course will Teach You the Prerequisites to Get Started
Travis CI
- How to Stop Errors Before They Ever Hit Your Codebase with Travis CI and ESLint
- How to Automate Deployment on GitHub-Pages with Travis CI
- How to Set Up Advanced Automatic Deployment with Travis CI
- How to Use Travis CI and GitHub for Your Web Development Workflow’s Heavy Lifting
Jenkins
- You Rang, M'Lord? Docker in Docker with Jenkins Declarative Pipelines
- How to Make an iOS On-demand Build System with Jenkins and Fastlane
GoCD
Ansible
Chef
Kafka
- How to Implement Change Data Capture Using Kafka Streams
- What to Consider for Painless Apache Kafka Integration
- How to Ingest Data into Neo4j from a Kafka Stream
- How to Build a Aimple Chatops Bot with Kafka, Grafana, Prometheus, and Slack
Terraform
- Terraform Workflow: How to Work Individually and in a Team
- What Are Terraform Modules and How Do They Work?
- How to Use Terraform to Automate Your AWS Cloud Infrastructure – Tutorial
- How to Extend Your AWS Infrastructure with Direct Connect Using Terraform
- How to Manage Wavefront Resources Using Terraform
- How to Build a Screenshot Capture API Using Terraform, AWS API Gateway, and AWS Lambda
In Closing
Thanks for reading this far. If you found this compilation of resources helpful, share it with your friends so they can learn something, too.
Was there an article or video tutorial you liked? Did I miss anything? Let me know over on Twitter.